Single react query doesn't seem to hit then endpoint in production (Vercel)
Hi there,
Thought i'd ask here as i'm at a loss
I have a dashboard page with 2 queries:
1.
getRecentContent
2.getDashboardStats
When I load the page in production, the Vercel logs show that only getDashboardStats
is getting hit, getDashboardStats
doesn't, even though its on the same page
So I figured it was a FE problem, so I went to log the query stuff, I notice:
1. The query is running
2. It flips from isLoading instantly, suggestive of something being returned very quickly before a network request is being made
3. The other query takes far longer to load in because it is actually hitting the API
It executes as expected in development
I would have thought there was some weird caching thing, but the two procedures are on the same router, and accessible via the same api route
Has anyone faced something similar, or can suggest something to look into?5 Replies
Here are some code examples if interested:
FE:
BE:
its being cached
its not a code thing, check vercel docs
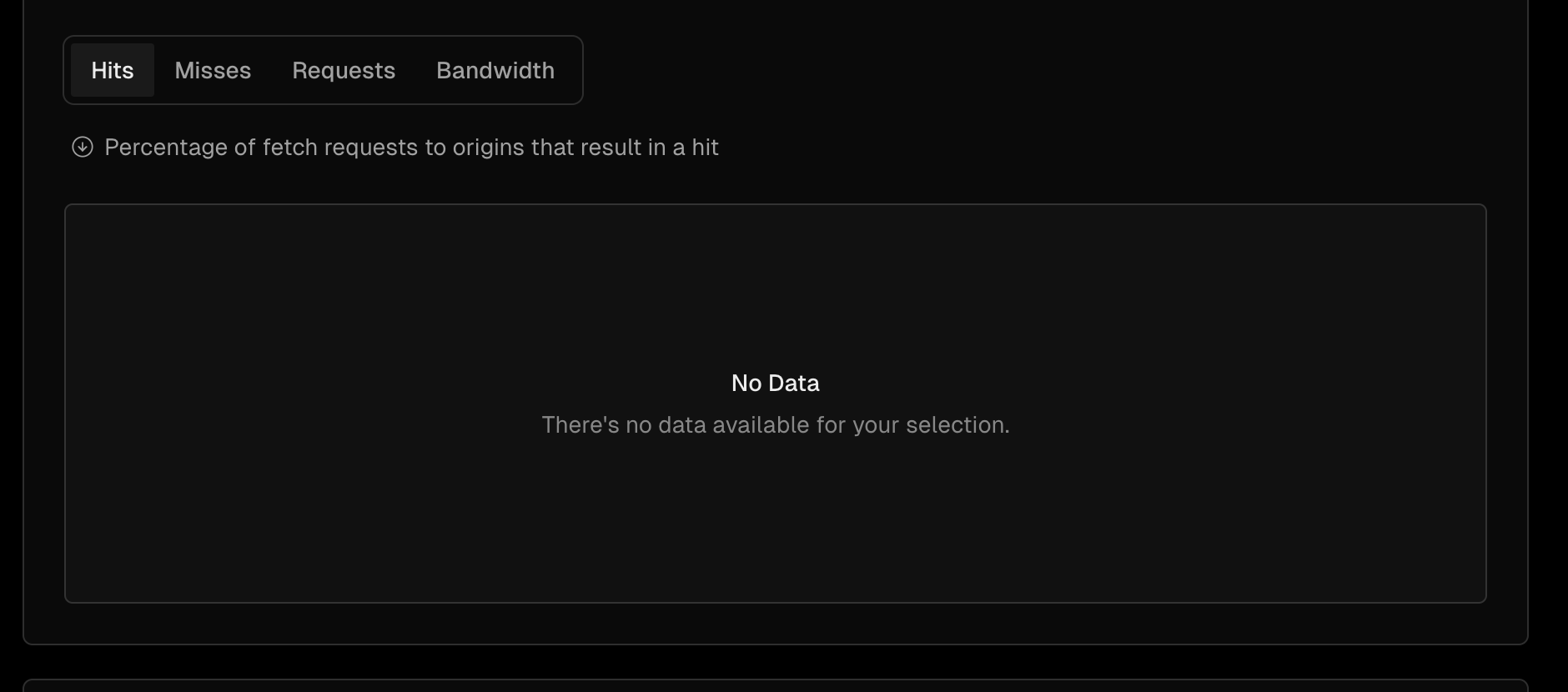
There doesn't seem to be any caching, and there isn't anything else that has this kind of behaviour
have you checked the Network tab of the browser dev tools? should tell you if the request is actually hitting your backend