✅ using Logging with ASP.NET
ASP.NET has a logging service. It looks way better than mine. I believe it gets set up here:
Where can i find/modify this method? How can i trigger my own logs?

66 Replies
you can inject that logger with
ILogger<TheTypeDoingTheLogging>
Logging in .NET Core and ASP.NET Core
Learn how to use the logging framework provided by the Microsoft.Extensions.Logging NuGet package.
ok, almost there. i figured out how to use the ILogger, but now i need to generate a new ILogger<MyType> logger and i cant figure out how to do that in a way that works...
it should Just Work
you don't need to generate that yourself, DI can figure out how to construct the service you're asking for
yes, i passed
app.Logger
into my process, but it logs as though is ASP.NET.
i think i explaned that badly...
My App has two processes running: an ASP.NET api and a discord bot. right now when my discord bot needs to log something it just prints to the console. this works, but ASP.NETS logging looks way better so i want to implement this for my discord bot. So somehow i need to generate an istance of ILogger<Bot>
that i can pass to my discord bot so it can log stuff and look nice.
oh, ist an ILogger.i'm confused, what setup do you have for both processes to log to the same console?
becasue i didnt know there was another option?
theres one console...
and do you actually need 2 processes compared to hosted services?
i dont know how to create a hosted service.
Background tasks with hosted services in ASP.NET Core
Learn how to implement background tasks with hosted services in ASP.NET Core.
you could run the bot portion as a hosted service in your ASP.NET Core application
that sounds more integrated at the least... i still want to create a seprate logger for the bot to more easily identify when the bot loggs somthing and when the API logs somthing.
right, all that is involved in creating a "separate" logger is injecting
ILogger<T>
where T is any type you want (but typically the type you're injecting the logger into)
This doesnt work
when i try and log somthing it dosnt do anything.
i don't know what that is, that's not what i'm recommending
then when you create that class through DI it will inject an appropriate instance for you
alternatively get it yourself with
serviceProvider.GetRequiredService<ILogger<MyClass>>()
there's a whole bunch of logging infrastructure that is set up for you in DI, but with your code you're basically creating an empty unconfigured logger so it won't do anythingin this block of code, i generate a Logger with the type of my Bot class. Then i pass that logger inot my discord bot and run it.
but that's not how you're supposed to use the ASP.NET Core logging infrastructure
which is why it's not working
Ok and your saying i have to make my bot a hosted service before it can access that?
no
i'm saying that's better than 2 processes, but at this point i don't think you're actually using 2 processes
so i'm confused
2 processes would mean you have 2 different exes that you're running
but if you want to run a discord bot in-process with your ASP.NET Core application, a hosted service is the most proper way to do that
no i am not doing that, sorry i don't have a lot of the nomenclature yet :(
perhaps this will help:
This is the end of my main method. after it builds the my API it adds the task running the API and the task running the discord bot to a list and runs them symaltaniously
i mean, that's a way to do it
but definitely not standard
only way i know how to do it, so i am doing it. Whats the normal way?
Jimmacle
Embed Type
Link
Quoted by
<@901546879530172498> from #using Logging with ASP.NET (click here)
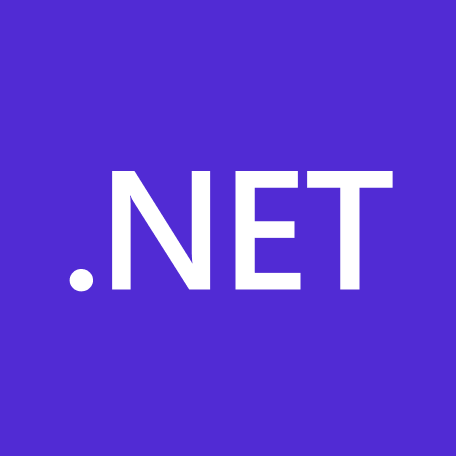
React with ❌ to remove this embed.
so createing a hosted service... i thought i wasent running a service? you said you expected that to be its own exe...
when you say "2 processes" that means "2 exes"
i never brought up processes, you shouldn't have 2
if you want to run a long-running task inside an ASP.NET Core app (like a discord bot) then hosted service is the correct solution
ah i see. so i need to implement my bot as a hosted service of the ASP.NET api. Then i can use the ASP.NET logging infostructure.
you register the hosted service in your app's DI and it will start and stop it for you when needed
ok, i will be back after i figure that out.
the registering it as a hosted service
the general look of a hosted service and registering it is this section in particular https://learn.microsoft.com/en-us/aspnet/core/fundamentals/host/hosted-services?view=aspnetcore-8.0&tabs=visual-studio#timed-background-tasks
if you inherit from a BackgroundService instead of implementing IHostedService it will take care of a lot of the boilerplate for you
ok. that was super useful
i think the last hurtle is my bot needs to access values within appsettings.json. How can a hosted service access the builders configuration?
prevously i did this by calling
builder.Configuration.GetSection("BotSettings").Get<DiscordSettings>();
then passing this value to the instance of bot, but i am not calling bot anymorestack exchange to the rescue: https://stackoverflow.com/a/58448732
Stack Overflow
Pass Parameters to AddHostedService
I am writing a .Net Core windows service and here is a snippet of code:
internal static class Program
{
public static async Task Main(string[] args)
{
var isService ...
ok. My Bot is a hosted service, and i can pass my bots config to it.
now back to the Logger,
i need to build a logger and pass that to the bot so it can log stuff.
ideally a
ILogger<Bot>
instead of an ILogger<WebApplication>
though that might block it?Unknown User•6mo ago
Message Not Public
Sign In & Join Server To View
not allowed to share this one :/
Unknown User•6mo ago
Message Not Public
Sign In & Join Server To View
yep, everything's in appsettings.json now (which is not on the git)
Unknown User•6mo ago
Message Not Public
Sign In & Join Server To View
separate project for an internship.
Unknown User•6mo ago
Message Not Public
Sign In & Join Server To View
NDA.
Unknown User•6mo ago
Message Not Public
Sign In & Join Server To View
ah no
Unknown User•6mo ago
Message Not Public
Sign In & Join Server To View
stupid me
sry
Unknown User•6mo ago
Message Not Public
Sign In & Join Server To View
give me a few
Unknown User•6mo ago
Message Not Public
Sign In & Join Server To View
these hands are under NDA
Unknown User•6mo ago
Message Not Public
Sign In & Join Server To View
GitHub
GitHub - hutonahill/huts-logging-tests
Contribute to hutonahill/huts-logging-tests development by creating an account on GitHub.
wont actualy work if you compile it but it should get the message across.
Unknown User•6mo ago
Message Not Public
Sign In & Join Server To View
... yep one sec
thats super weird
done
Unknown User•6mo ago
Message Not Public
Sign In & Join Server To View
no i think that showed up when i was figureing out .NET identity...
Unknown User•6mo ago
Message Not Public
Sign In & Join Server To View
yes, got a bunch of values the discord bot needs. figured no point in implimenting that here. not where the logger is or anything, but thats how the discord bot gets my token along with a bunch of other settings.
dont know what you mean here...
figgured
Unknown User•6mo ago
Message Not Public
Sign In & Join Server To View
cool
and that will grab the settings out of the appsettings.json file?
under the BotSettings key?
Unknown User•6mo ago
Message Not Public
Sign In & Join Server To View
yea, Secrets > appsettingsDevelopment > appsettings
or somthing like that
Unknown User•6mo ago
Message Not Public
Sign In & Join Server To View
Thanks a ton!
Unknown User•6mo ago
Message Not Public
Sign In & Join Server To View
If you have no further questions, please use /close to mark the forum thread as answered
will do