How to get EXE file?
Hi i just started coding in c#
I was wondering how can i get an .exe file out of my Project.
When i build it or publish it, it creates an exe file but in a Folder with more files so it cant get executed alone. I can only execute it when its in the Folder but how i actually make it executable without all the folders ? Ty for your help!
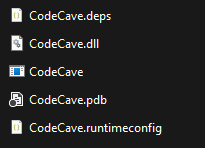
37 Replies
Open your project in Visual Studio (assuming you're using Visual Studio).
Right-click on the project in the Solution Explorer and select Publish.
In the Publish dialog, click Start to create a new profile, or select an existing profile if you have one.
Choose Folder as the publish target.
In the Configure your publish settings section:
Set the Target Framework to the appropriate .NET version.
Set Deployment Mode to Self-contained.
Select the appropriate Target Runtime (e.g., win-x64 for 64-bit Windows).
Ensure that the checkbox for Produce single file is checked.
Click Publish.
then it creates a setup file... which cant be open alone
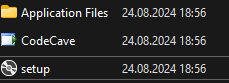
Open your project in Visual Studio.
Go to Build > Publish [Your Project Name].
Choose Folder as the target for publishing (instead of ClickOnce).
Under the Configuration section:
Choose Self-contained for the deployment mode.
Choose the appropriate target runtime (e.g., win-x64).
Make sure to check the option Produce single file.
Publish the project.
try like this
If Visual Studio continues to create a setup file, double-check that you aren't using ClickOnce deployment. You can disable ClickOnce by going to the project properties:
Right-click on your project in Solution Explorer and select Properties.
Go to the Publish tab.
Uncheck the Enable ClickOnce Security Settings if it's enabled.
ty for helping it worked!
Now i have a issue that the exe file is 60mb big. Any easy methods to compress the file size?
Cut out unused packages or use dotnet 8 AOT
C# programs typically aren't self contained, they require a runtime to be installed
making your program self contained puts the whole runtime inside your exe, which makes it a lot bigger
they also have additional files like DLLs based on the libraries your program uses
if you want a single file without the runtime, you can set PublishSingleFile to true and SelfContained to false
$singlefile
dotnet publish -c Release -r <runtime identifier> -p:PublishSingleFile=true
Use of -p:PublishSingleFile=true
implies --self-contained true
. Add --self-contained false
to publish as runtime-dependent.
-r RID
and -p:PublishSingleFile=true
can be moved to .csproj as the following properties:but to target multiple RIDs, you have to use dotnet publish
with the -r
option for each RID.
You can also add -p:IncludeNativeLibrariesForSelfExtract=true
to include native libraries (like Common Language Runtime dlls) in the output executable.
You might want to instead publish your application compiled Ahead Of Time to native code, see $nativeaot for examples.
Single file publishing | Runtime Identifier (RID) catalog | dotnet publish
$nativeaot
Problem is when i try to use AOT i cant create a single file

Alternatively cut down unused packages
And zip it afterwards
you probably don't want AOT
it has limitations that you need to be aware of and some programs just aren't AOT compatible
:SCfeet:
What's the benefit of AOT then
i dont understant how else i can compress my file then...
:SCchips:
what do you mean by that?
by using the other options
In Visual Studio you get the option to not include unused packages
no runtime requirement basically, probably smaller file size
i don't think there are many actual advantages, it's not even necessarily faster because there's no PGO
but you also can't use reflection, runtime code generation, or anything along those lines
so if you don't know better trying to compile AOT could just break your program
:SCshocked:
same deal with other more aggressive methods of reducing file size, there are ways to remove unused code but it's not smart enough to account for reflection etc
Zip files should do the job then
i mean, that doesn't really solve the problem once you actually want to use the program
but for distribution sure
I'm assuming the problem is the distribution
Can anyone explain if its possible to get my 14mb exe file to around 1mb?
:SCshocked:
maybe if you use C or C++
unless youre building malware that needs to spread over bad connections you do not need a program that small specially if its anything more than a hello world program
:stare:
why?
the answer is a giant $itdepends
$winforms
my fav speed dial
not sure how that's relevant here
because when try to create stuff with forms the file gets 150mb even if its only "Hello World" in the code. There must be a way to reduce size no?
That's because your forms applications with "only hello world in the code", does not only contain "hello world" in the code
that wasnt my question but ty
The only things you can do is not include dotnet but then the target system needs it or zip it
There's no other realistic way to drastically reduce file size