Arrange points in circle with a Sweep parameter
Hey, so I'm positioning some points in a circle. Which is easy, but I want to add a 'sweep' parameter.
The issue is if I just subtract 1 from the point count when calculating the angle increment. Then the last and first point will be at the same position when
sweep
is 1
. But if I don't, then the points will look like they are 'off by one'. Meaning with sweep
at 0.5
, the first and last points will not be perfectly inline.
Not sure how to go about fixing this. I included a little graphic showing what is currently happening and what I want to help
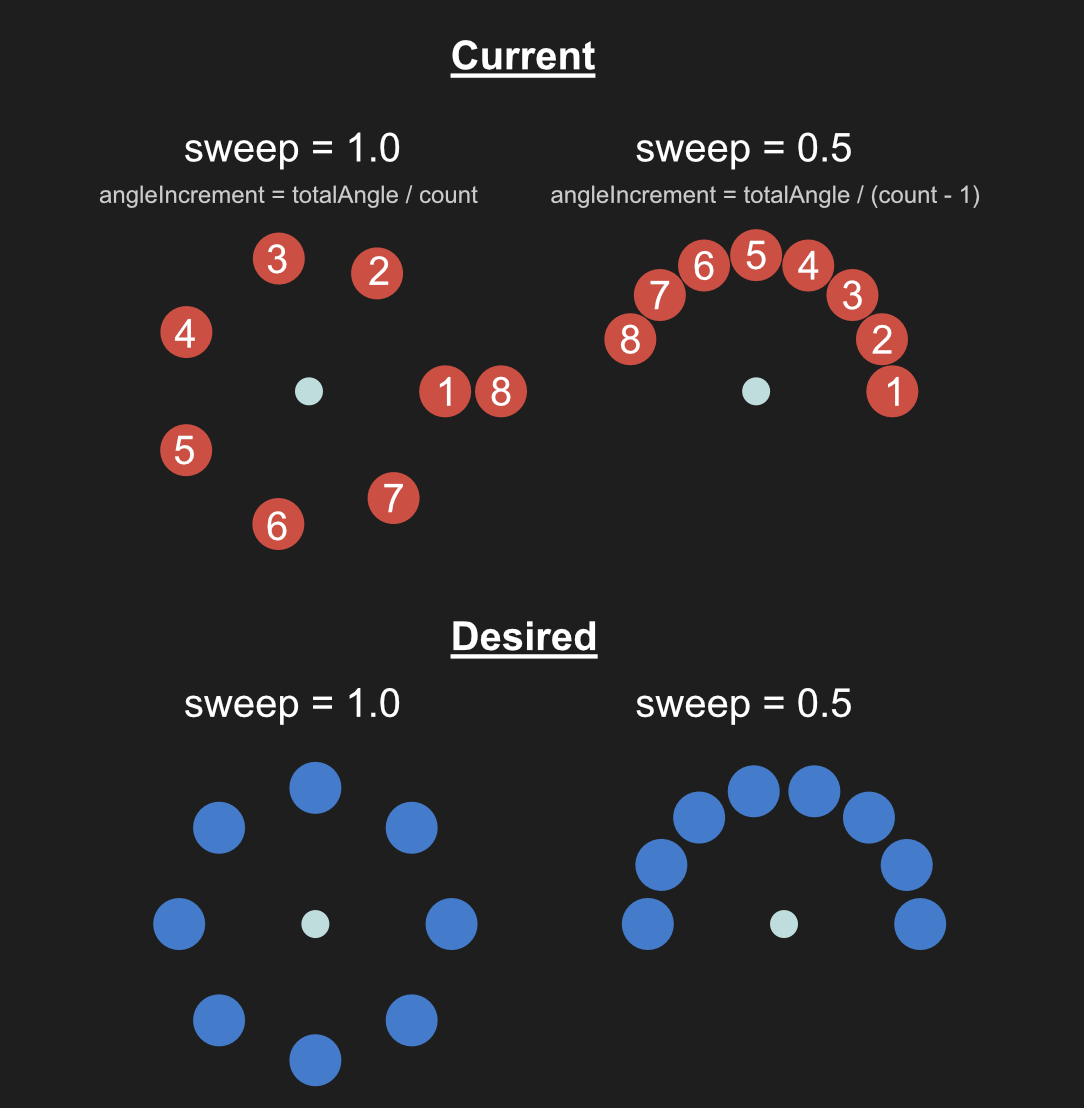
5 Replies
Things is, there's really 2 different things you are doing, trying to find a unifying formula that does both is impossible.
There can be multiple solutions to your problem, you can handle the 1.0 case separately, but this will give weird behavior when sweep is close to 1.0. You can try setting a threshold near 1.0 that you determine empirically, where you use different formulas depending on if it's above or below it. You can use a bool paramater to switch between the two. You can decide which approach to use depending on how the function is going to be used
@MechWarrior99 I think you should just divide by the count.
In your "current, sweep = 1.0", to get that graph you divided by "count -1", not by "count" like you state.
You have 8 points. It's 360 degrees. It means "increase by 45 degrees per point" if you divide properly. You'll have the image of "desired".
It holds for sweep 0.5 too.
I think that you were just confused when you plotted it and you ploted your "count-1" try for both.
His images are swapped, if he divides by count he'll get the problem on right side of the image.
total angle = 180.
increment = 180/8 = 22.5
The last point is 7 increments after the first, so at angle 157.5, not 180 as expected
there really are 2 different things he wants to do. At sweep = 1, he wants to place uniformally on a circle, where the distance between first and last is same as distance between any other. But for sweep = 0.5, he wants to place the points uniformally on a line, where first and last are on the edges
You're right. I stand (sit) corrected. gj
Ahh you're right. That's unfortunate, oh well. Thank you for explaining how I was thinking about it wrong. Guess I will just pick something.