Issue with select
How would I handle doing what I'm doing in the raw part during the select?
I get the following error:
No overload matches this call.
Overload 1 of 3, '(selections: readonly SelectExpression<Database & { townhallDistribution: { level: number; amount: string | number | bigint; }; }, "clan">[]): SelectQueryBuilder<Database & { ...; }, "clan", { ...; }>', gave the following error.
Type 'RawBuilder<unknown>' is not assignable to type 'SelectExpression<Database & { townhallDistribution: { level: number; amount: string | number | bigint; }; }, "clan">'.
Type 'RawBuilder<unknown>' is missing the following properties from type 'DynamicReferenceBuilder<any>': #private, dynamicReference, refType
Overload 2 of 3, '(callback: SelectCallback<Database & { townhallDistribution: { level: number; amount: string | number | bigint; }; }, "clan">): SelectQueryBuilder<Database & { ...; }, "clan", { ...; }>', gave the following error.
Argument of type '(string | RawBuilder<unknown>)[]' is not assignable to parameter of type 'SelectCallback<Database & { townhallDistribution: { level: number; amount: string | number | bigint; }; }, "clan">'.
Type '(string | RawBuilder<unknown>)[]' provides no match for the signature '(eb: ExpressionBuilder<Database & { townhallDistribution: { level: number; amount: string | number | bigint; }; }, "clan">): readonly SelectExpression<Database & { ...; }, "clan">[]'.
Overload 3 of 3, '(selection: SelectExpression<Database & { townhallDistribution: { level: number; amount: string | number | bigint; }; }, "clan">): SelectQueryBuilder<Database & { ...; }, "clan", { ...; }>', gave the following error.
Argument of type '(string | RawBuilder<unknown>)[]' is not assignable to parameter of type 'SelectExpression<Database & { townhallDistribution: { level: number; amount: string | number | bigint; }; }, "clan">'.ts(2769)
8 Replies
Did you notice the documentation is full of examples? There's also an examples section in kysely.dev.
I've been scrolling through that to understand the builder, but I must've missed the example that pertains to my issue
You need to provide a type and an alias for the raw SQL snippet.
I'm sorry but which example? It's been a long day so it's probably the fatigue
https://kysely.dev/docs/examples/select/complex-selections
https://kysely-org.github.io/kysely-apidoc/interfaces/SelectQueryBuilder.html#select
On the second one, I can't link to the exact position, but it's there
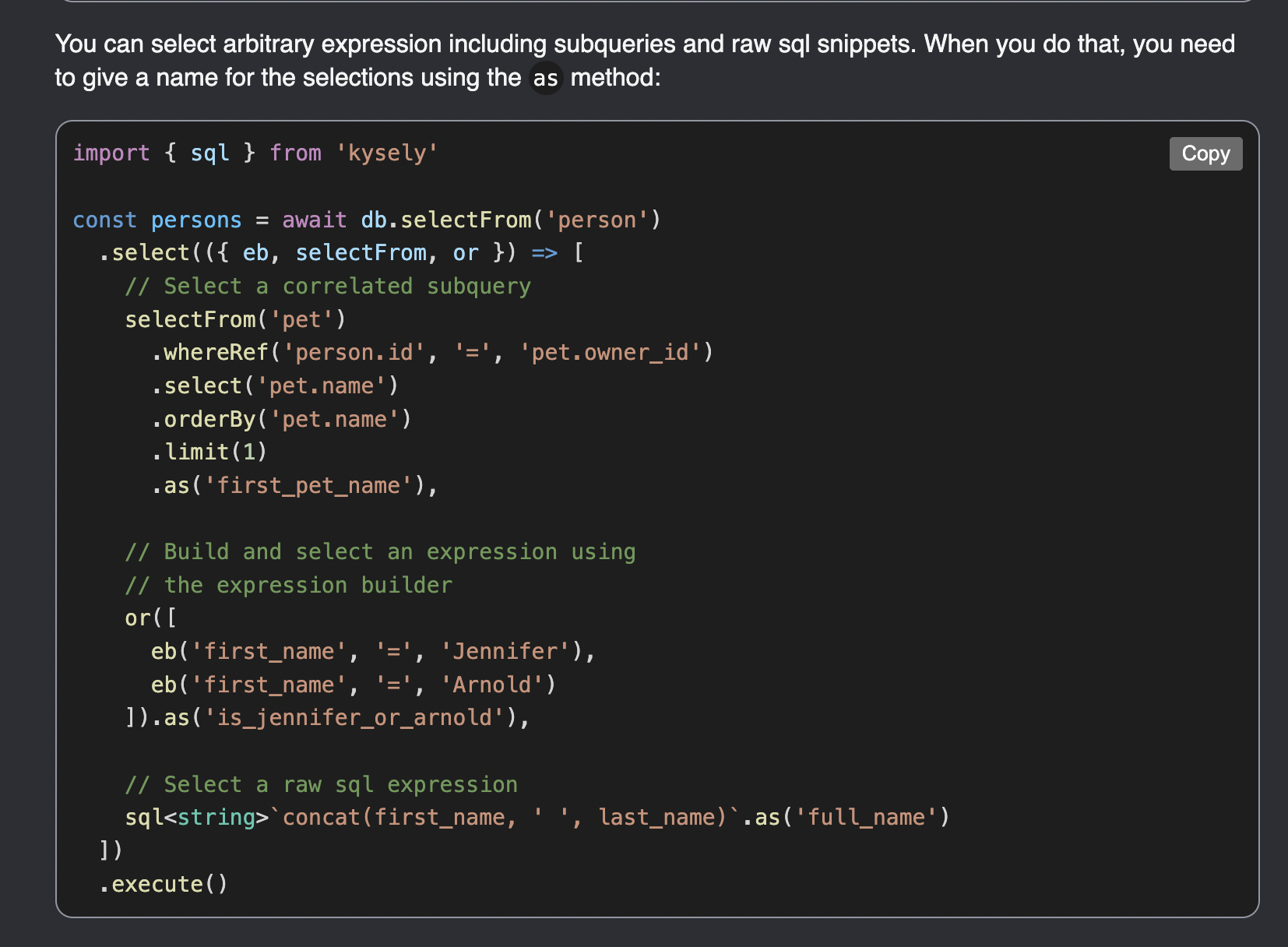
You can see that same documentation just by hovering over the
select
methodReally must've missed it, didn't mean to annoy you my bad. Very new to kysely and TS, and tbh as I've learned in this project coding in general
I'll post my full query if someone stumbles upon this