✅ Beginner needs help in C# HtmlAgilityPack and Linq query
Hi! I'm a beginner who try to webscrape some informations about soccer players from a website (transfermarket.com) for personal use.
I already got the informations I want into a datatable/datagridview by the following text:
This writes the table into my datagridview and is fine so far.
The only thing I want (and failed so far) is to get a link for each soccer player which is not part of the "td.InnerText", but "td.InnerHtml".
In the screenshot I show you on left the original website, middle my scraped datagridview and on the right the last information that I want to scrape to the datagridview too (for each player / each datarow)
How can I extend the Linq Table query to extend only that one (in my screenshot the marked line of my browser editor) or do I need to create an additional query?
Thanks!! 🙂
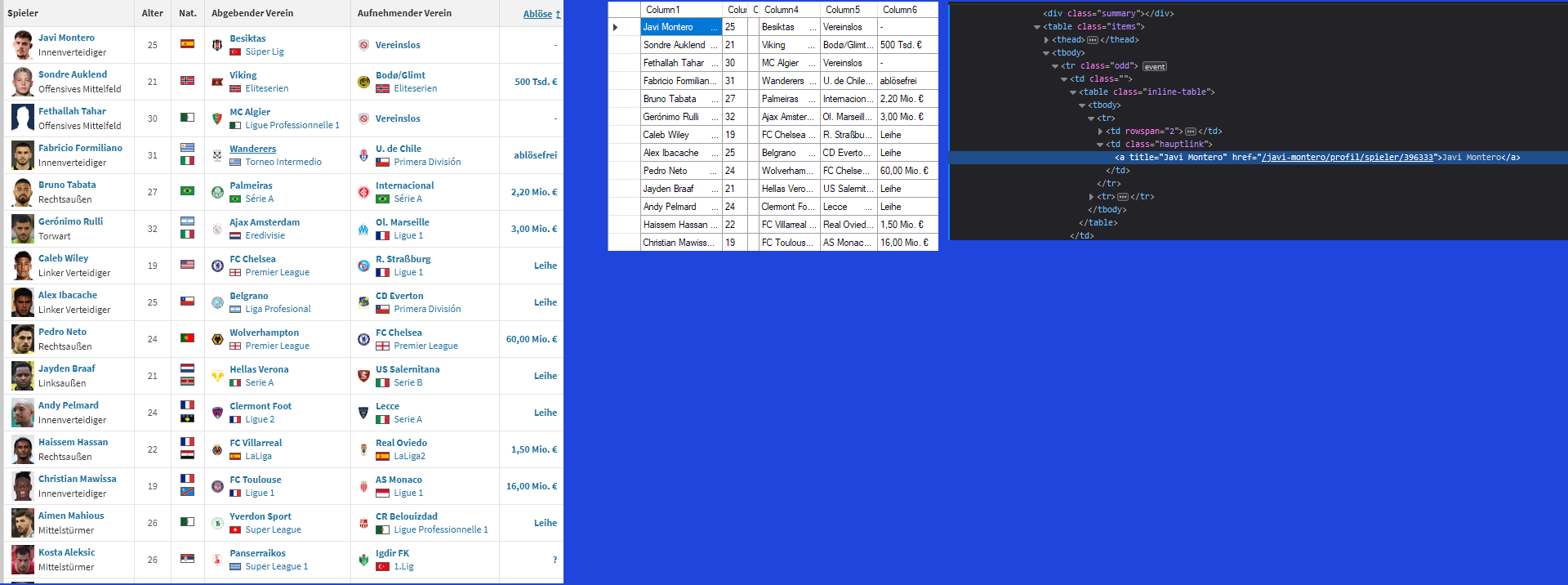
8 Replies
Why not something like:
That way you get a strongly-typed Player object as well, where you can map the different properties onto your DataGrid columns, rather than just having an anonymous "Column 1", "Column 2", etc
And you get to have different parsing logic for the different properties, which is what you need
Wow thanks 🙂 I didnt know I can just mix the InnerText and also the attributes
I'm running on a older c# version, so primary constructors are not available, but I'll try to upgrade it. 😄
That's a record (available slightly earlier). You can also just write that as a normal class:
Or in even older C# versions:
(Then construct with
new Player() { Name = ..., etc }
of course)Yep, that worked, thanks!
I've it now this way:
This works perfect and also helped me to understand how easy I can user some classes to help me for structured data. thank you so much canton 🙂
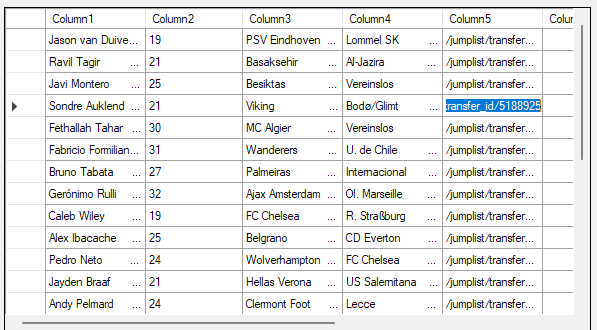
Cool, good stuff!
In that DataGrid, you should be able to manually map columns to properties (exactly how you do that differs between winforms and WPF)
That will let you put proper column names on there, rather than "Column1" etc
👍
Tbh I'd break that linq expression apart, and just use an expression to get the trs/tds, then use a normal foreach loop to construct the PlayerScrape instances
It'll be a few more lines of code, but easier to read and probably easier to debug as well
$close
If you have no further questions, please use /close to mark the forum thread as answered