server actions function use inside useEffect return undefined
export async function getRecipesForUser(
userId: string
): Promise<RecipeResponse> {
const user = await validateRequest();
const ownerId = user?.id ?? "";
if (!user)
return {
data: null,
error: "No user found",
};
try {
const recipes = (await findRecipesForUserById(userId, ownerId)) as Recipe[];
if (!recipes)
return {
data: null,
error: "cannot find user",
};
return {
data: recipes,
error: null,
};
} catch (error: any) {
return {
data: null,
error: error,
};
}
}
export async function getRecipesForUser(
userId: string
): Promise<RecipeResponse> {
const user = await validateRequest();
const ownerId = user?.id ?? "";
if (!user)
return {
data: null,
error: "No user found",
};
try {
const recipes = (await findRecipesForUserById(userId, ownerId)) as Recipe[];
if (!recipes)
return {
data: null,
error: "cannot find user",
};
return {
data: recipes,
error: null,
};
} catch (error: any) {
return {
data: null,
error: error,
};
}
}
"use client";
import React, { useEffect, useState } from "react";
import type { Recipe, RecipeResponse } from "@/types";
import RecipeCard from "./RecipeCard";
import { getRecipesForUser } from "@/lib/actions/recipe";
export default function OwnerRecipeList({
userId,
ownerId,
}: {
userId: string;
ownerId: string;
}) {
const [recipes, setRecipes] = useState<Recipe[] | null>(null);
const [error, setError] = useState<string | null>(null);
useEffect(() => {
const fetchRecipes = async () => {
try {
const recipeList = await getRecipesForUser(userId);
console.log(recipeList);
} catch (error) {
console.error(error);
setError("Failed to fetch recipes. Please try again later.");
}
};
// Call the async function
fetchRecipes();
}, [userId]);
if (error) return <div>{error}</div>;
console.log(recipes);
return (
<div className="w-[70%] pl-5">
{recipes?.length ? (
recipes.map((recipe) => (
<RecipeCard
key={recipe?._id?.toString()}
recipe={recipe}
ownerId={ownerId}
/>
))
) : (
<div>No recipes available.</div>
)}
</div>
);
}
"use client";
import React, { useEffect, useState } from "react";
import type { Recipe, RecipeResponse } from "@/types";
import RecipeCard from "./RecipeCard";
import { getRecipesForUser } from "@/lib/actions/recipe";
export default function OwnerRecipeList({
userId,
ownerId,
}: {
userId: string;
ownerId: string;
}) {
const [recipes, setRecipes] = useState<Recipe[] | null>(null);
const [error, setError] = useState<string | null>(null);
useEffect(() => {
const fetchRecipes = async () => {
try {
const recipeList = await getRecipesForUser(userId);
console.log(recipeList);
} catch (error) {
console.error(error);
setError("Failed to fetch recipes. Please try again later.");
}
};
// Call the async function
fetchRecipes();
}, [userId]);
if (error) return <div>{error}</div>;
console.log(recipes);
return (
<div className="w-[70%] pl-5">
{recipes?.length ? (
recipes.map((recipe) => (
<RecipeCard
key={recipe?._id?.toString()}
recipe={recipe}
ownerId={ownerId}
/>
))
) : (
<div>No recipes available.</div>
)}
</div>
);
}
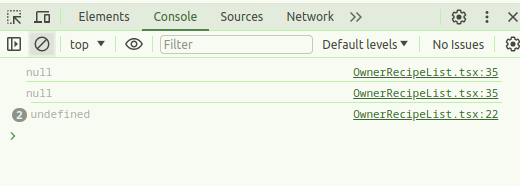
1 Reply
thank you guys so much