JS Shorthand
Steps to reproduce:
1]using if statement:
2]using shorthand
=>This statement gives me an error stating ';' expected.javascript
let compValue: undefined.
Can anyone pls explain me this
60 Replies
randomNum>=0&&randomNum<1/3 && compValue='Rock';
This line as it is doesn't make any sense and will return a JS error but not the one that you are getting.
Can you provide the complete code block/function?maybe you want this??
This is the shorthand for IF-ELSE
GUARD OPERATOR
This can be written as a shorthand using only if statement isnt it?
not in javascript
rather,
randomNum>=0&&randomNum<1/3 && compValue='Rock';
isn't valid javascript
I know some other languages allow that, but JS isn't one of them
you could write if (randomNum>=0&&randomNum<1/3) compValue='Rock';
if you wantthis would do it, but it will assign 'false' as the else
if you want a single line, this could also work
Its called as the && operator in js
yes, and you can't use it to chain assignments
Meaning?
you can't use && and then at the end assign something to a variable
it's just not allowed in JavaScript
there are other languages where that works, but not in JavaScript
instead of assigning what else can be done apart from that?
it's a logical AND operator, so you use it to check multiple conditions
can we do this?
you have to wrap it, then it will work. Like I showed here
(here an article explaining it: https://dev.to/dailydevtips1/javascript-if-shorthand-without-the-else-13ch)
could you pls explain this in detail.
no not really. i don't like these kind of assignments, for me it doesn't read nice.
The && operator is used to combine the condition check with a value ('rock' in this case).
As i understand:
const compValue = (randValue >= 0 && randValue < 1 / 3) && 'rock'
If the condition on the left (randValue >= 0 && randValue < 1 / 3)
is true, the entire expression evaluates to the value on the right ('rock')
.
If the condition is false, the entire expression evaluates to false.the third example represents the same as i have done in my example
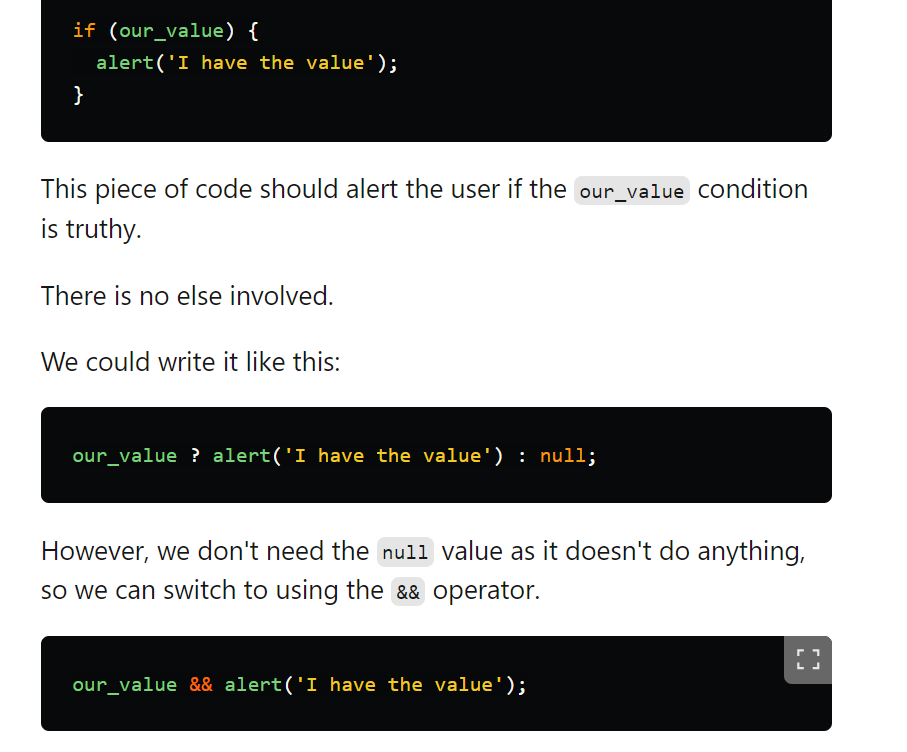
yea, but your condition has already the and && operator in it, so to make it logically correct, you have to wrap it in
()
yes. But again, ask yourself if this is easy to understand/readable. (imo, don't do this)
I am getting your point .But the term says Shorthand.
this is also a shorthand
it throws an errror actually.
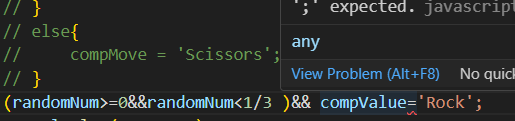
Sorry, i missed that, you're not assigning
let compValue = (randomNum>=0&&randomNum<1/3 ) && 'Rock'
why we cant assign here
because that syntax is for a conditional expression. not an assignment. It's just not how that works.
Got it.
what if the randValue is 0.2. it seems confusing whether to get into rock or paper isnit?
no thats 0.2 < 1 / 3 (0.33333)
0.2<2/3 as well
the first condition that matches will assign
if smaller than 1/3 ? => rock
else if smaller than 2/3 => paper
else => scissors
Got it .Thanks for explaining in detail .
no problem
=>compMove is false,rather we want compMove to be Rock
yes, then probably the randVal is not smaller than 1/3
as I've said
in some case it doesnt work
randVal is 0.5785133209921858

waste of time
The problem is your logic doesn't branch so it is as if only the line
compMove=(randomNum>=2/3) && 'Scissors'
is run as the other lines are just trampled by this line.
Why not just chain ternaries.
compMove = (randomNum>=0 && randomNum < 1/3) ? 'Rock' : randomNum < 2/3 ? 'Paper' : 'Scissors';
getting your point for ternaries(if else-if).Why lines are trampled rather this is && operator(multiple if statement) i have to get to know why its not working.Else guard operator doesnt have meaning in mine example.
===============================
Maybe that will make a little more sense, I feel like you are close to understanding the issue but there is just a little switch before it comes together. If this doesn't clear it up at all I can try again.
You have three assignments statements but only the last one will win. So you can change it so the last one is the most comprehensive or you can change it down to one assignment. Another option would be:
This is still a single assignment statement but it is only using
and
and or
to get the value.
here though randomNum is <1/3 why is it returning false? thats my first doubt.
because you're assinging it 3 times... "// The following line will set compMove to false or 'Scissors'"
Bro, learn basic coding flow before you start writing garbage.
Because the syntax you are using is boolean && string.
The boolean is for shortcutting the rest of the logic, if the first part is
The boolean is for shortcutting the rest of the logic, if the first part is
false
then stop executing logic on the and
as no further values matter. If the value is true
check to see if the next value is true
.
So that means if the first part evaluates to false
the assignment will be false
, it will not even attempt to look at the string.
However if it is true
then further evaluation need to be had.
Non-empty string are true
in js but the do not return the value true
but rather the value of the string.
Therefore those assignment statements must return your string literal or false
This isn't helpful, many times I have written esoteric code to understand the code better. This could very well be this person path to understanding basic code flow.I'm not trying to be helpful, I'm trying to communicate or shame someone into realizing they are wasting other people's time forcing code to do things they do not understand.
You do not need to read this you are wasting your own time, don't blame others for your decisions. This is a forum for help, if you have no intention in being helpful your input is not welcome.
They don't want to hear that some code patterns are not used because of readability; they don't want to slow down to understand ternaries and chaining, they want to get a thing to do a thing the way they want.
There is value in that, pushing a code to its limits has merit in learning. If this were production code, it would be inadvisable, if this is just for learning, and I suspct it is since it is Rock, Paper, Scissors, then this is perfectly fine.
Pushing code to its limit lol
yeah, you can encourage this mess all you like.
wrt this, evaluates to true because randomNum <1/3 so the right part should be continued i.e 'Rock'
it is, and then you re-assign it false twice.
it doesn't magically break out of the code somehow.
More accurately the following statement when true will return 'Rock'
More simply put:
So if that is the case then the following will also never return 'Rock'
So in your code sample from above what would this do:
compMove will be set to
false
;
compMove will be set to 'Paper'
;
compMove will be set to false
;
Therefore trampling 'Paper'
got it .
this gives the clear picture.
Highly appreciate the patience,efforts,time to give such an example and make me understand the flow.A very big thank you for you great help.Thank you
no problem, I have seen many people help out on this discord and I believe most people see this as a way to learn and teach. When you are further down your coding journey then it will be your time to teach, but for now just keep trying to learn 🙂
To make it into a single line and avoid multiple assignment to compVal why cant we do
simply because JavaScript does not allow anything other than a variable name and optionally the keywords
let
, const
, or in a pinch var
on the left side of the assignment operator
also, if I'm being brutally honest, it's entirely unreadable and likely to cause bugs
as are most of the workarounds. Write code that is readable firstAssignment has to follow
{{varname}} = {{value}}
so you cannot do {{value}} {{operator}} {{varname}} = {{otherValue}}
This is why I proposed:
instead of doing that, why don't you use arrays?
['rock', 'paper', 'scissors'][Number.random() * 3]
if you want to allow translations, you can do this:
if you want to translate to other languages, just change the value for each key
(btw, don't forget to truncate the number)You were told hours ago that you can't assign a value that way, and handed multiple "single line" solutions written in actually useable ways. Like I said, you don't want to learn, or aren't able to due to gaps in basics.
I would be shocked if they are able to work with objects.
you can, if you wrap in parenthesis
that then becomes a valid expression
minified code does this a lot, and this is HORRIBLE!!!!
DO NOT WRITE CODE LIKE THAT
you're not a machine, you're a human
write code you can read in 2 days, not code only god and v8 will understand
Exactly. The more I read this discussion, the more I thought I was looking at some production-built ultra-compressed JS external script manual generation tutorial. The only thing I anticipated to see but didn't are those random letter variable and function names and maybe, just maybe, you should add them to this code, you know, just for good measure.
Jokes aside one reason some people (myself included) may get confused by && (especially because they might have learnt it through experiential learning, not text-book memorization) is because in jsx inside {} if you add a condition which is true && <Comp/> it will render
that's because the
&&
operator resolves the expression by passing the right-side element as the result
if you do 1 && 5
you get 5
instead of true
but again, the code that op is trying to write is code that only minifiers should be writting
you need to focus on making human readable code