OOP design pattern question
I have an interface called IModel for voxel models. I don't want to change it because it has lots of existing implementations without a base class.
There are about a dozen different operations that can be done on the IModel interface. Currently these are static methods in a static class with no global state.
Certain special implementations of IModel have special implementations of some of these methods that get the same result more efficiently from taking advantage of their internal data structures beyond what IModel offers. These are only available for some operations on some IModel implementations some of the time, not consistently.
I want to make it so that when user code calls one of these operations, the program will choose the more efficient special implementation when available or resort to the default IModel version if not available.
What OOP design pattern should I be following to make that happen?
17 Replies
just to clarify, when you say the default IModel version, do you mean a default interface method?
I hate smart phones and the Internet in general so much. I hate it all.
Right now, I can't edit my question on my phone. I can get in to make my edit but the "Save" button is unresponsive.
I think maybe the real solution is to join the Amish. My plow wouldn't do this crap to me.
Um no. The IModel interface doesn't currently cover these dozen or so operations at all.
I can post a link to the code
my first thought would be to add another interface...
IHasSpecialImplementation { T SpecialImplementation(Foobar arg); }
and then you can check if a given IModel is an instance of IHasSpecialImplementation.
if (foobar is IHasSpecialImplementation special) { special.SpecialImplementation(); } else { foobar.Whatever() }
in that case, if you want a default impl. for things to fall back on, the default interface method feature sounds like what you want
Default interface methods - C# feature specifications
This feature specification describe the syntax updates necessary to support default interface methods. This includes declaring bodies in interface declarations, and supporting modifiers on declarations.
Oh that might be nice
I think that would probably do it. I could just overload the default methods when needed.
yes, i think that would work
apologies, i thought your question was different than what it actually was originally
Can the overriding methods refer back to the default methods?
Like if special case, do special implementation, else run the default method from the interface?
i'm honestly unsure as i've not used the feature myself before
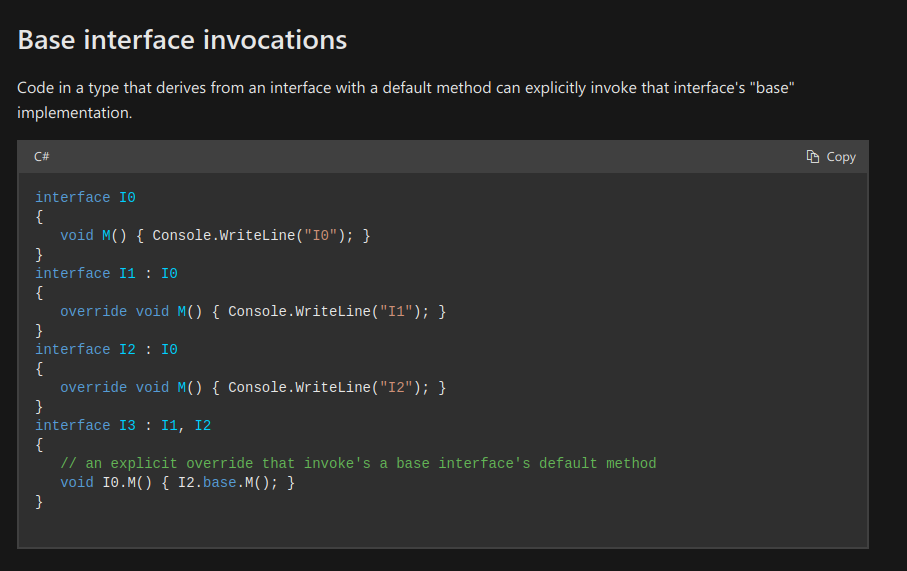
seems like the answer is yes
It looks like this is actually a proposal, not a real existing C# feature.
Also I'm limited to .NET Standard 2.0 + PolySharp
I would use the default methods if it were available but I think it is not.
I'll probably need to use something like this.
That IHasSpecialImplementation interface ... What members would it have?
Maybe one method to check which special implementation methods are available and one to actually run them?
they're real, they've been in for multiple versions
Oh nice
if you're on .net standard you may be screwed though
Assuming I had to use that IHasSpecialImplementation interface, what members would it have?
like this
Oh, I didn't quite get what you were saying before. I think I see now that you included an idea of what it could look like in your post.
I am going to need to make sync and async versions of everything available so that will muck it up a bit in terms of complexity.