Problems with modal
This is my modal, I wanna talk about the quantityModal here, the button for it is there, but it just gives an error when im trying to execute it, im unsure where even beginn to look for the problem
7 Replies
- What's your exact discord.js
npm list discord.js
and node node -v
version?
- Not a discord.js issue? Check out #other-js-ts.
- Consider reading #how-to-get-help to improve your question!
- Explain what exactly your issue is.
- Post the full error stack trace, not just the top part!
- Show your code!
- Issue solved? Press the button!
- ✅
Marked as resolved by OPThats the error it basically just says, something went wrong please try again
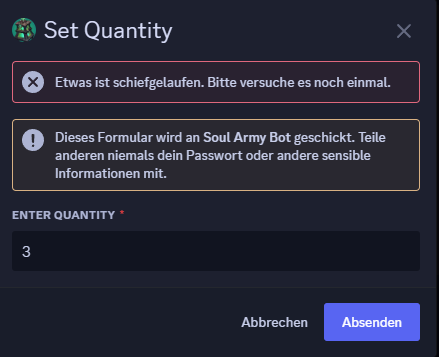
modals aren't message components, so modal submits can't be collected with a message component collector
if you want a collector, you'd want to use
<Interaction>.awaitModalSubmit()
:method: ButtonInteraction#awaitModalSubmit()
@14.15.3
Collects a single modal submit interaction that passes the filter. The Promise will reject if the time expires.
Ahh, that makes total sense damit
Ill give it a read and mark the case as solved then, thanks
I just notice you said, "if you want a collector" for me it implies there are other options for it?
@duck
well there's always just listening to the
interactionCreate
event
you can also instantiate InteractionCollector
yourself if you didn't want a promisified collectorDamn, I guess I need to read the docs again about those options, but since I managed to make it run with the change you named me Im happy for now. thanks alot