JavaScript Array Manipulation: Issues with forEach
When i want to modify an array like below, it doesn't modify the original array. Why is that?
If i change the
arr = arr.map((item) => item = "");
to gameBoard[index] = arr.map((item) => item = "");
it works as expected and modify the original array. What is the difference here?130 Replies
Array.map() creates and returns a new array, it does nothing to the original.
Array.forEach(), on the other hand, doesn’t create a new array or return any value. Instead, it performs a function on each value inside the array.
Also, the
forEach()
method takes a function that has the item and index, not the array and index
So of course the item will be the same as the gameBoard[index]
:pYes, know that but, my logic is that
arr
is an object that has a reference to an array (depends on the index) inside the gameBoard so, i think i can change it but, this isn't the right logic it seems. I'm confused with arr here actually.What exactly are you trying to do? Your code is a bit confusing so I’m not sure what the current results are vs what want them to be
Also, what does an item in your game board array look like?
I want to change the array. This array has three sub arrays and i want to access all of them and change their values to "". So, i thought if i forEach the gameBoard array; arr parameter gives me arrays inside gameboard Arrays and i can change this arrays.
Sorry, if i misleading you to wrong direction
and gameBoard array is 2D array.
it has 3 arrays inside it
and they have 3 empty strings
I will change to my phone because i will go to my home but, i want to talk about it if you have time.
is this for tictactoe?
yes
why dont you use a single flat array?
actually, i dont know. You are right about it 🙂 but, i want to know why this behaviour happens though
If you’re resetting everything why do a recursive search? Why not just set the main game board to a new, empty/reset array?
^
also, foreach doesnt update the array values
Yes guys, you are right about your implementations and mine is bad i know it. I just wonder why in this situation JS behaves like that
you are updating a local variable inside a foreach
and that's it
the value will be lost
it behaves like that because foreach is the wrong tool
But why gives true when i check the equality between them
copy on write?
i think v8 uses that
Arrays are shallow copies, so you’re comparing, in essence, the exact same thing
when we compare arrays isnt it comparing reference value on memory?
sorry i dont understand
copy on write
it copies when you write into the shallow clone
or reference
probably my knowledge isnt quite good about this topic, i will research it
Edit fiddle - JSFiddle - Code Playground
Test your JavaScript, CSS, HTML or CoffeeScript online with JSFiddle code editor.
check that
you will see it shows
true
then false
wait, i was right and wrong
map returns a new array
it wont update the old arrayyes
in essence, you are changing a local variable
When comparing an array I believe it compares each item in the array and checks the memory address for complex objects, or discrete values for primitives, ands if any are different they’re not considered equal
its an object, so, its compared by reference
reference is the same? then it's true
different references are false, even with the same elements
thats the confusing part for me actually
[] is not the same as []
If a reference contains another reference it has to compare each inner reference
comparing both is always false
and when we pass arguments as an object to function, parameter is a local variable of course but it still holds a reference right?
Array methods create shallow copies, which is a reference to the actual object yes
nope
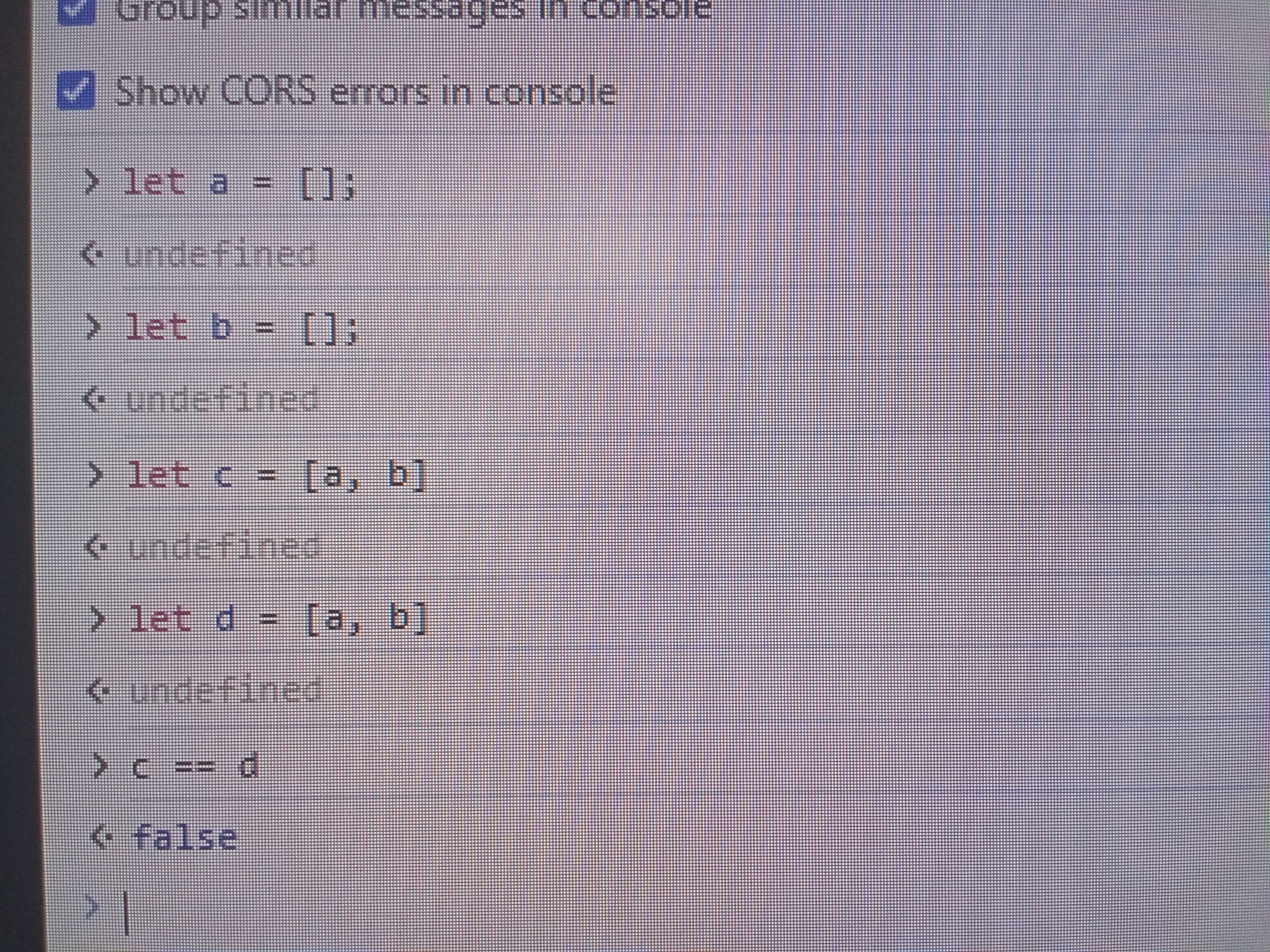
but when you assign a nee array, it doesnt reference the old value anymore
Cool, thanks for checking. In on my phone waiting at work so I’m going off of memory here
you're welcome
js is complicated
no worry guys at least you have a memory
my brain melts rn
😃
let me draw it for you then
yes js is confusing
Now do:
d.forEach( (item, idx) => console.log(item === c[idx]) );
That’s gonna be true
for both, IIRCyes because it has the same reference?
yes
Indeed
but when you assign
item.map
, it received a new arrayyes and then i returned the new array to arr
wich didnt work
foreach doesnt do anything with returns
the value is ignored
Whelp, time to actually work. Have fun, y’all! :x13ladWave:
good time my man thank you
map will update the value in the array, foreach wont do anything
returning a value is like tossing it into a void
it doesnt update it returns a new array
not foreach
yes foreach doesnt return anything
map updates the value on a shallow copy of the array
as far as i know it creates a new array with given callback function
doesnt mutate the original one
neither of those is true
this is what it does
as far as i know
Can you look it up on mdn
you are right
because i am phone i cant give a link my phone is slow as hell
it creates a new array
not a shallow copy
yes
that sounds inneficient
what do you mean with shallow copy actually
isnt it a primitive and object values combined
a shallow copy is when you copy a value, but keep the references of the other elements in the object
hmm
for example, you have x = []
now you create y = [x]
it is a reference right
to x in the array
if you clone y into z, then y[0] and z[0] will reference x
thats a shallow clone, shallow copy or whatever
yes
there are many names
a deep clone or copy will also clone x, and will clone all the values in x
shallow copy is an object with objects then right
not exactly
hmm
my brain failing me today
you create a new object, but any references in it aren't copied
they still reference the object
hmm
a deep copy goes deep and copies everything
imagine you have x = []
yes
and then you create y = [x]
the first element of y is a reference to x, right?
yes and x is an object
it's an array, but yes
so, a shallow copy is the same as doing [x] again
a deep copy is the same as doing [[]]
if x = [12345]
then a shallow copy is still just another [x]
but a new deep copy is [[12345]]
hmm
as far as y is concern x's actual value isnt important because they are pointing the same block on memory?
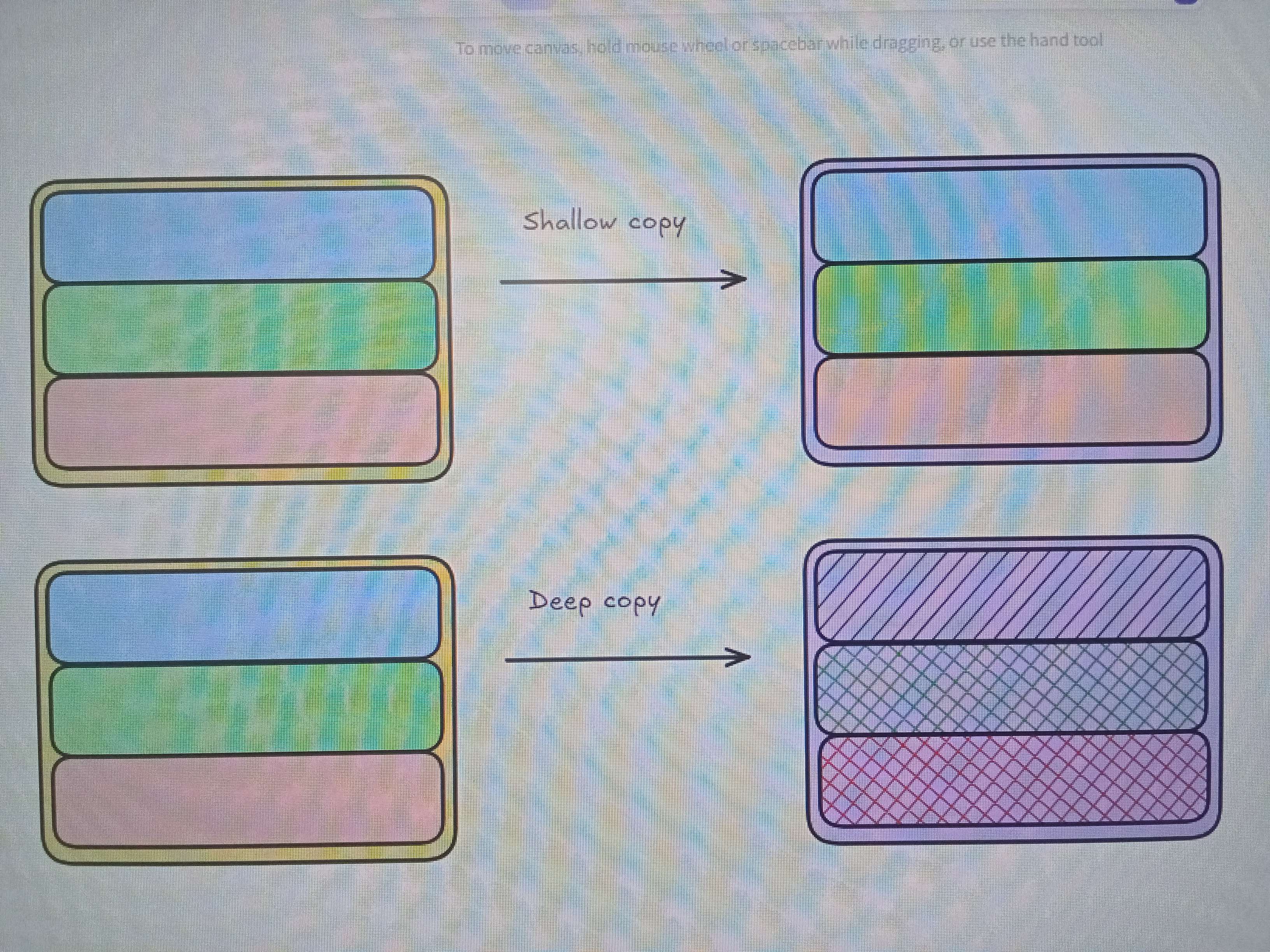
this is a shallow vs deep copy
deep copy is another object then
but shallow is same
no, always a new one
notice how the right side is purple
instead of yellow
oooo
shallow copy is another object but if it has objects to reference they dont bother to copy them
it keeps the same reference
yes
the deep copy will copy the object it is referencing
thats why i said object in object but you said it wrong how
yes
if it is primitive
i said it was an array, in the example, because the prototype is different
but an array is an object, but an object isnt an array
yes
no, no. a deep copy will copy EVERYTHING
because arrays prototype is object
the prototype of the array prototype is object
yes i understand but if it has objects then it cant do that right because it holds reference then
yes sorry
and in prototype chain there will be object.prototype
Thank you for taking your time man i dont want to bother you because of this nonsense that i dont understand
but it was a nice conversation
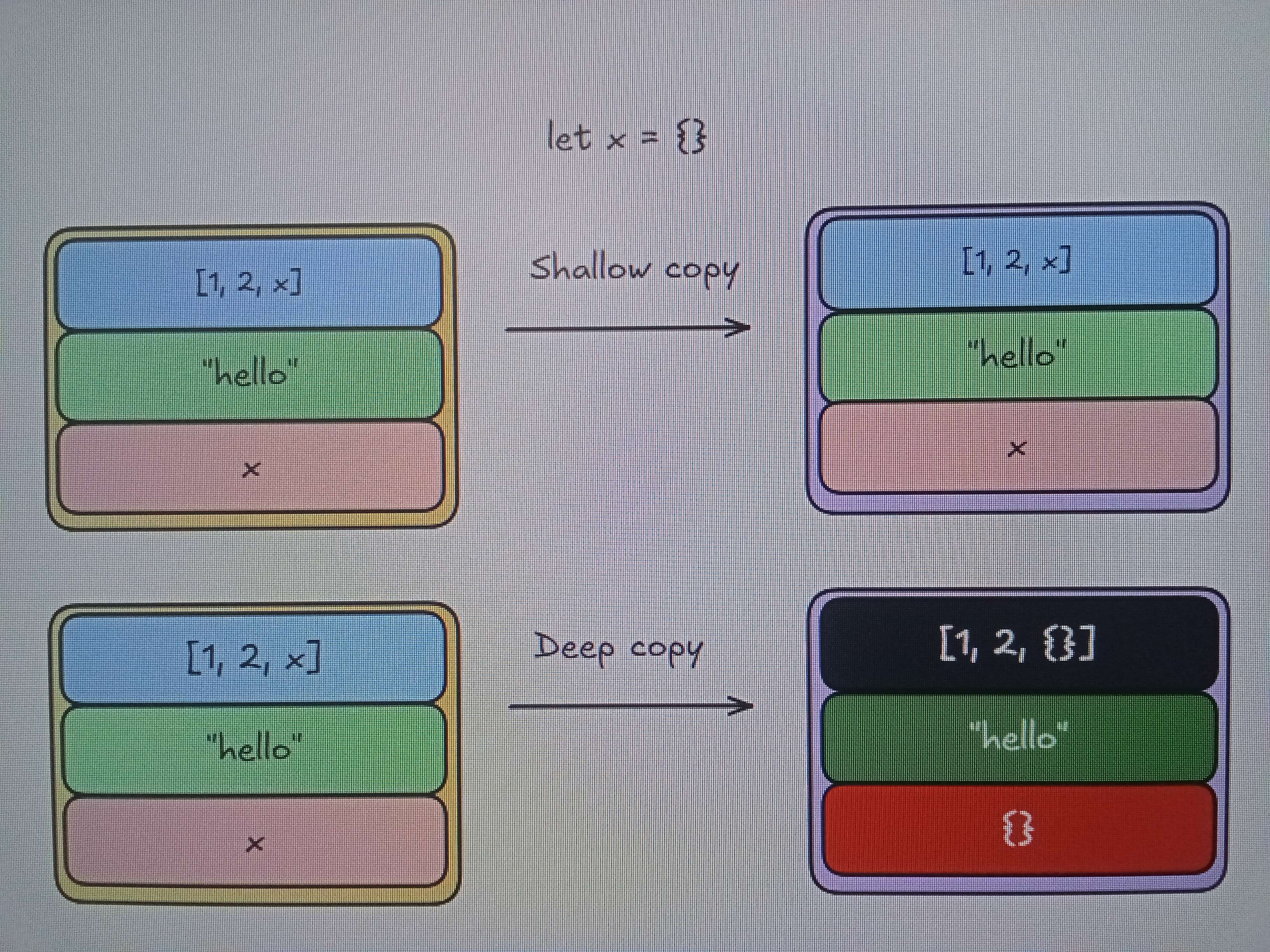
and now, does it make sense?
notice how the x isnt x at the bottom
you're not bothering, but, you're welcome
yes i understand that actually but "hello" needs to be deep copied right?
on the top one
it is always deep copied because its a scalar value
thanks mate
can i add you as a friend. i will read an articles about what mentioned then we can talk with more knowledge but, there is one issue i still didnt understand my first local variable question and i didnt find a good answer on the internet. Maybe i need to research it a little bit more
finally managed to send a proper copy
this is a much more readable version
thanks
very appreciate it
nothing personal, but i would prefer not to. ive rejected that others add me. i want to avoid coming to discord and not being able to use it because people are always asking stuff in private
Okay, not a problem. You have the right to do so
you can ping me here, in this channel
Thank you
you're welcome. if you want to do stuff like that, or edit it, use excalidraw
just upload the svg there and you have everything editable
it is a good one
Okay, i will keep in mind
it is, and it is very simple
and easier to use than paint
i have used it actually
straight to the point one
i use it a lot
you have any interest in graphic design
or just showing some stuff with it
not really, im awful at it and have very little interest in it
the graphics you made was good on that one you send me
its just squares and rectangles with the automatic align tool
nothing special
i know excali has built in tools for that but you can use it on blogs if you have one
i dont have one
nvm then
but i see lots of diagrams on blogs and stuff
@ἔρως i think i understand
but i will read more
alright
do you understand why the js now isnt doing what you expect it to do?
please send your code in codeblocks, not inline code
sorry my bad
i will fix it when i have time
👍
yes
i did research about shallow copy
and more importantly about pass by value and pass by reference terms
Javascript is a pass by value language so, when i pass an array as an argument it holds a reference VALUE
In my 2D array, forEach go over arrays. So, arr parameter holds a reference to that nested array.
If i want to change it with its index like arr[index], i can do it because the variables inside that array empty strings which is primitive type
but if i want to change the arr itself which holds a reference as a VALUE, i lost that reference.
But if Javascript would be a pass by reference language my statement would be true
yeah, if it was an actual pointer, yes
like in c
but it's not like it
Yes, primivite values have pointer too but JS handles it for us. I will remove the pointer thing from my answer. True is just "reference"
yes, reference is better
How are you my friend
im okay
nice of you