Connections are over connection limit
I deployed my NextJS + Prisma on a dedicated server then I noticed that the connections on my Postgres database is higher than the connection limit.
I set my connection limit to 30 (
?connection_limit=30
) but the connections go to around 70 - 80. You can see it from the graph. I already made sure if the data from the graph is correct by using this query.
And, here is how I initiate the Prisma client.
Another weird thing is that I extracted metric from the Prisma to Statsd but The data doesn't match with data from Postgres database. The Prisma idle connection is less than data from Postgres. You can see from the graph.
So, all I want to know is
1. How can connections go over limit?
2. Why are Prisma metric and Postgres data different?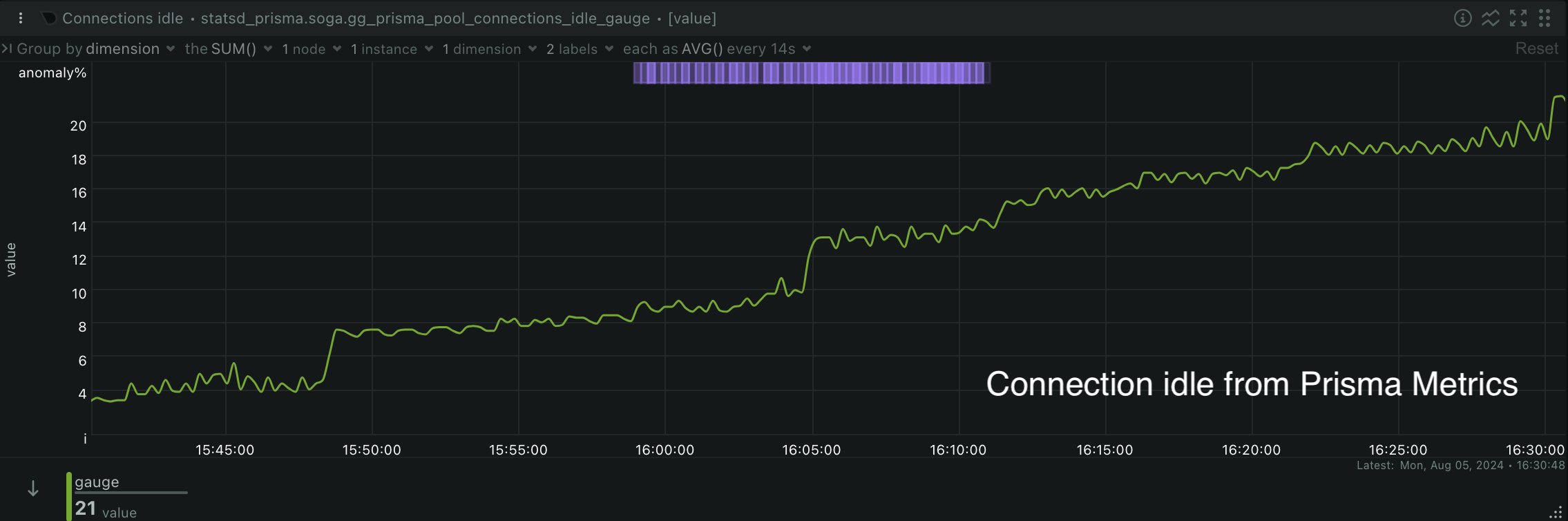
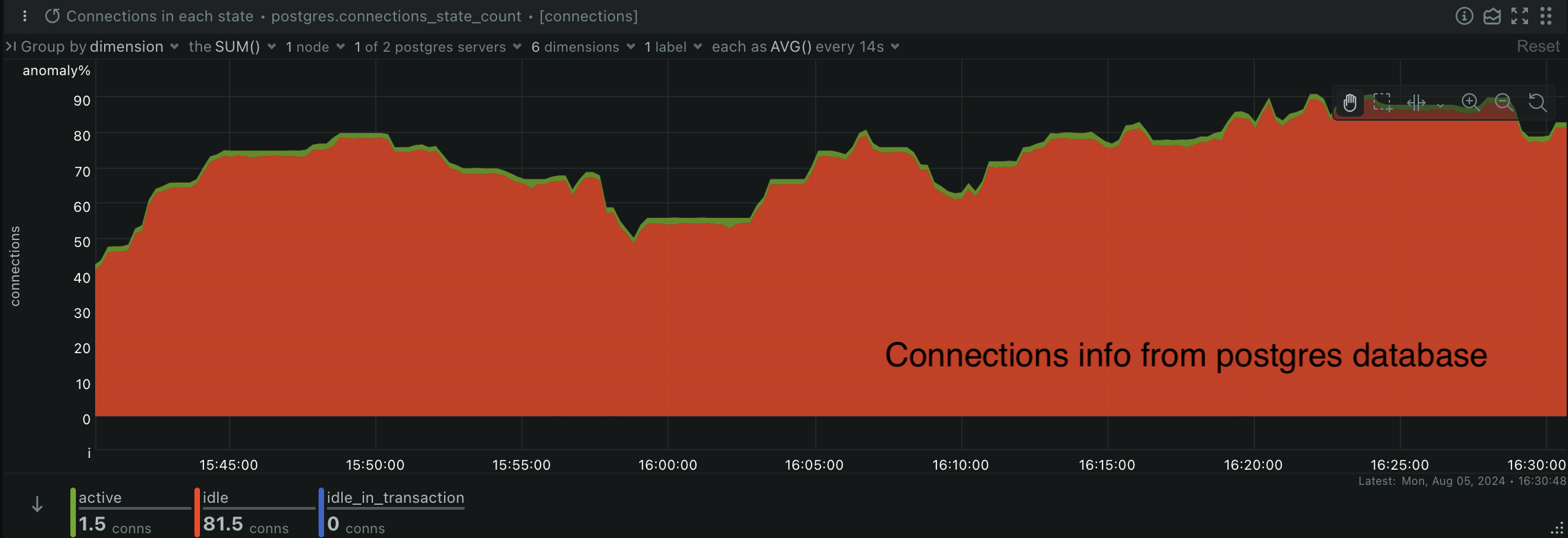
Solution:Jump to solution
In the end, it's not problem with Pisma. It's problem with our PM2 config. We set instances to "max" which is too much. I reduced the instances number and it works now. Thank you for support. @Nurul (Prisma)
13 Replies
Here is how I send data to Statsd
I use
node-statsd
to send dataHey 👋
Can you share the output you get when running this SQL Query?
Are there any other external services connected to your database or is prisma the only one?
Here the query result @Nurul (Prisma)
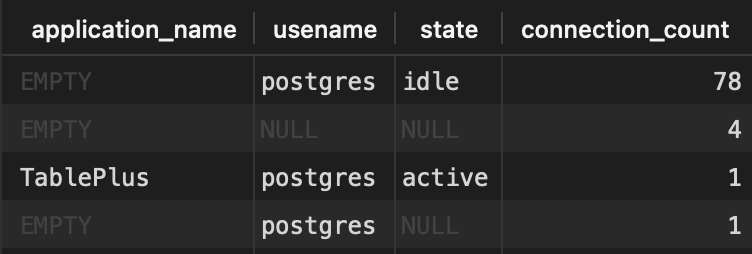
The result from my query
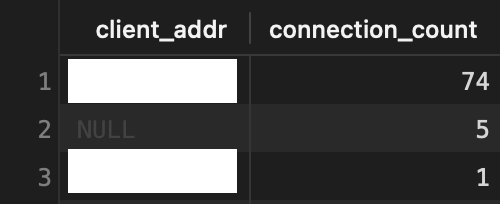
I also checked each activity one by one to see if idle activities really have the sql statements from server too.
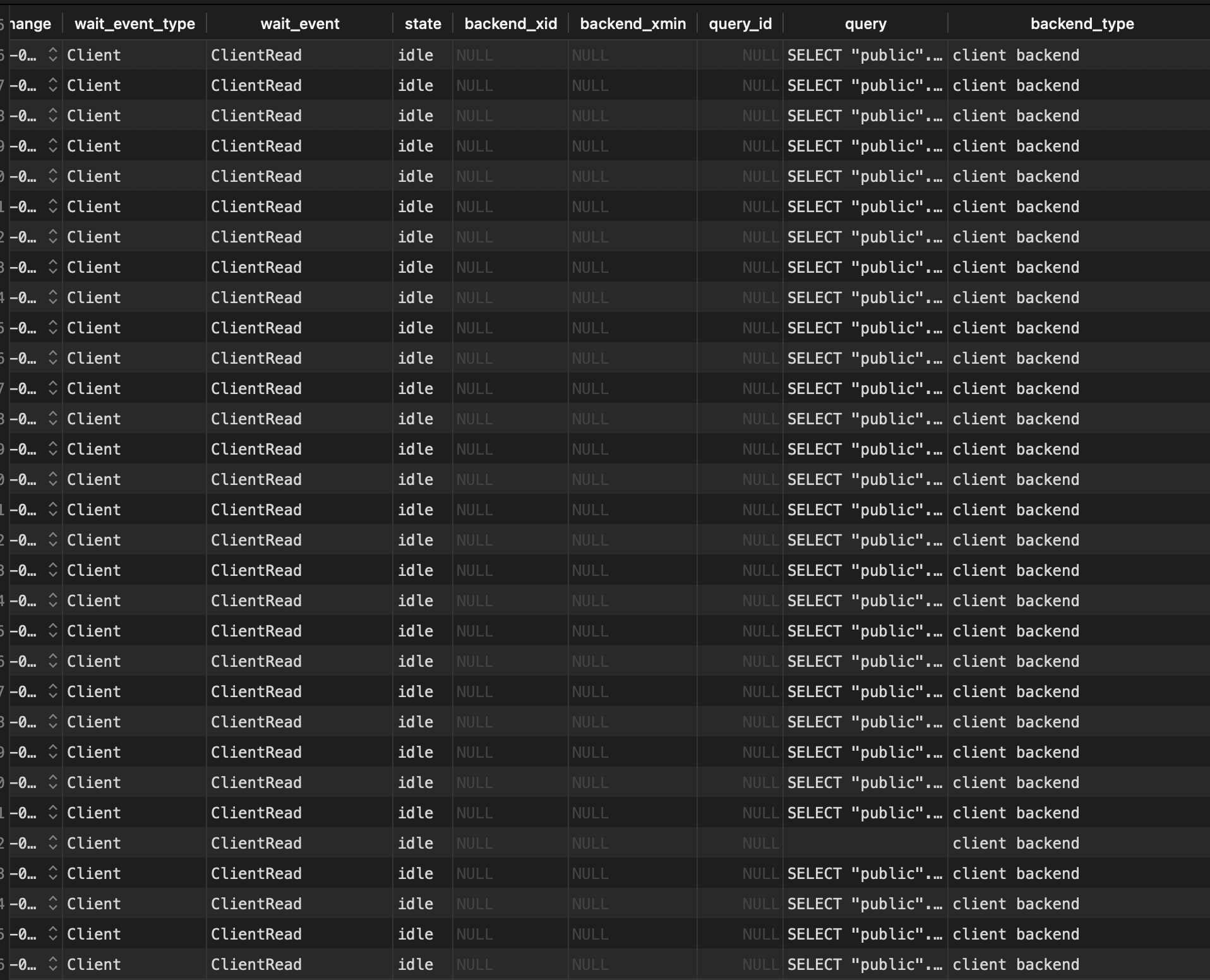
I only have a server container connect to the database.
Thank you for running the query and sharing the output with me.
To confirm, there is only a single PrismaClient instance in your application, right? You are not instantiating Prisma Client twice.
Ideally, this shouldn't happen. Let me ask the ORM team to get additional insights
@Nurul (Prisma) Thank you very much. I will try adding counter in the prismaClientSingleton function to make sure that the code didn't run more than one time too then.
Hey im having a similar issue, Id really appreciate if you guys can fix this 😢
I count the initiation. I notice that at the start of my container, Prisma instance created multiple times.
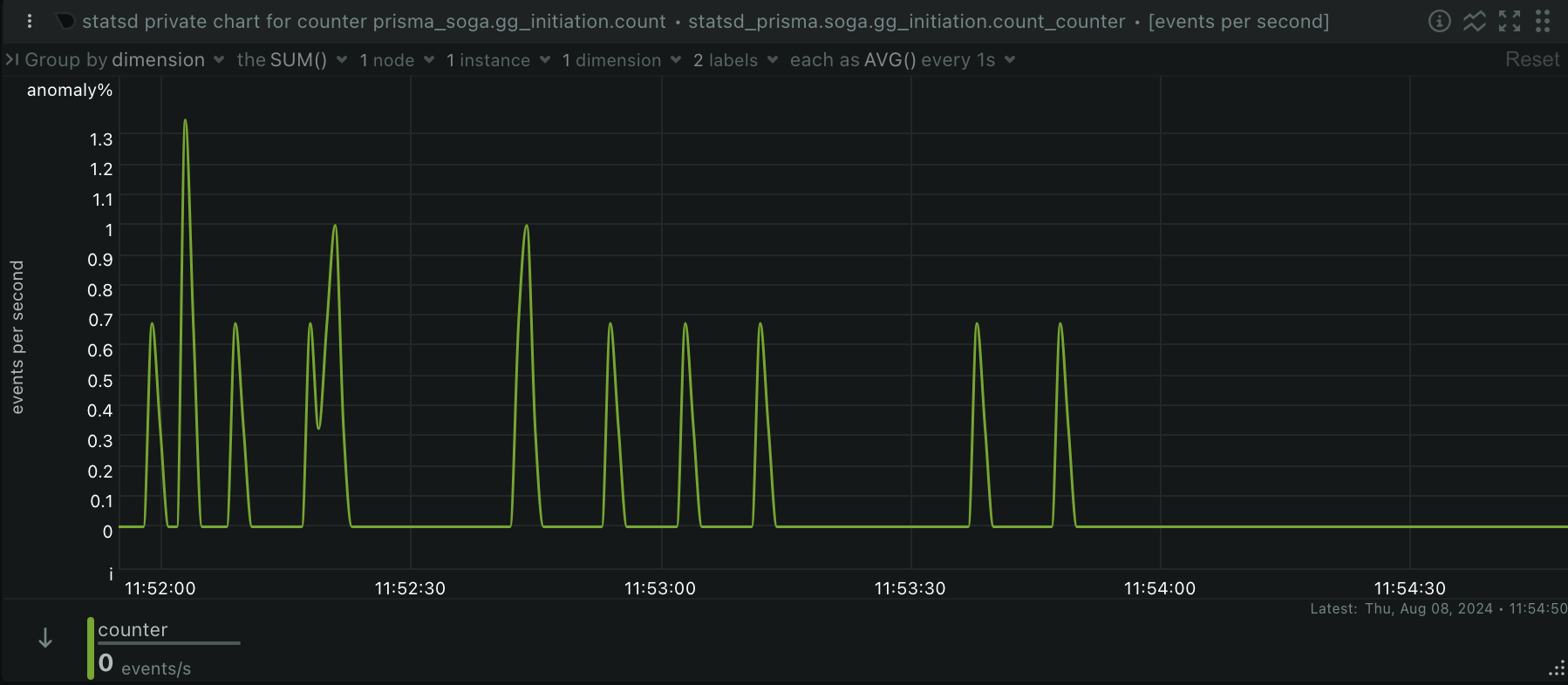
prismaClientSingleton is called multiple times and globalThis.prismaGlobal always be undefined on prod.
I tried changing the code and add more log.
global.prismaGlobal
still always be undefined on prod.
But, on local, it has value after being set.Solution
In the end, it's not problem with Pisma. It's problem with our PM2 config. We set instances to "max" which is too much. I reduced the instances number and it works now. Thank you for support. @Nurul (Prisma)