✅ attempting to encrypt with ECB
hello. i am trying to encrypt some data however i get different results from what im doing in python as a test
here is the python code
and here is my recreated c# code
here is my starting bytes
98AD3BBBBE513C6C350912819A7A6FE4A46232396461383831623535303762343965306431393935373830356435376563A431A433353939363839A437A40000
after encryption in python
376bb7559e97f260b449928cac9a73b9c73597a4fa55a392d77218f357078073a3cc72635d3d58656203b4df436b0fe41c8a962418f61e3ab2d9b1179cff0f727c469775a7e6c17e780afc9a7ab3eb44
after encryption in c#
B9161861D4B8C6BFFCF574172E570426A3CC72635D3D58656203B4DF436B0FE41C8A962418F61E3AB2D9B1179CFF0F727C469775A7E6C17E780AFC9A7AB3EB443D0D871C865D5449AB015558F784802F
i know the python implementation is correct.59 Replies
You seem to be adding a padding in the C# version but not the Py version
iirc python auto pads i think
But what mode
not sure
Everything must match in order to get the same result
I assume it's the padding
If your AES is hashed in a third party lib maybe consider switching to a built in class
aes needs padding of / 16 bytes right? my source bytes should match that
I'm not sure
The iirc AES can have a variable prefix and key length as long as they match a specific pattern and length guideline
Ill have to look later as its 4am rn and ive been working on this since 1am
Aight
Are you encrypting strings? Maybe the encoding is different
What key are you using for your test, so we can try and replicate?
It also looks like the Python key is a string? (From the
.encode()
). You don't show how you're turning the C# string into a byte arrayThe previous function already returns a byte array
What's "the previous function"?
Im calculating a byte array given some other data
In that other function
OK, and do you want to share that function with us, so that we can see if it does the same as the Python code does?
The key being different is one possible cause of your problem
Also:
What key are you using for your test, so we can try and replicate?
Ive already verified the bytearrays are the same. I will get the key soon but i havent been able to get to my pc yet
I think you meant achieve deterministic AES encryption?
I've no idea what that means...
You need to use specific modes which allow IV inputs
ECB doesn't use an IV
yes that's the issue
Is it?
well if he's trying to achieve deteministic encryption
Comparing output from AES encryption is useless imo
Isn't encryption supposed to be getting random data in the first place?
AES is always deterministic. The fact that he hasn't set the IV shouldn't be an issue, as ECB doesn't use the IV
No. If you encrypt the same thing with the same key and same parameters, you should get identical output
but are the modes same and those parameters?
Obviously something is different, as the output is different
the key is "jo6aey6haid2Teih"
and both implementations use the same key?
yeah
SharpLab
C#/VB/F# compiler playground.
SharpLab
C#/VB/F# compiler playground.
@literally a cat Is that how you're turning the key string into bytes in your "other function"?
im using
Encoding.UTF8.GetBytes("jo6aey6haid2Teih")
for the key
ok so by default python's ecb mode does not apply padding
wait a sec
i think my previous function might be incorrect
here was the python source
c# rewrite
wait its too long for discordC# Online Compiler | .NET Fiddle
Test your C# code online with .NET Fiddle code editor.
debug output
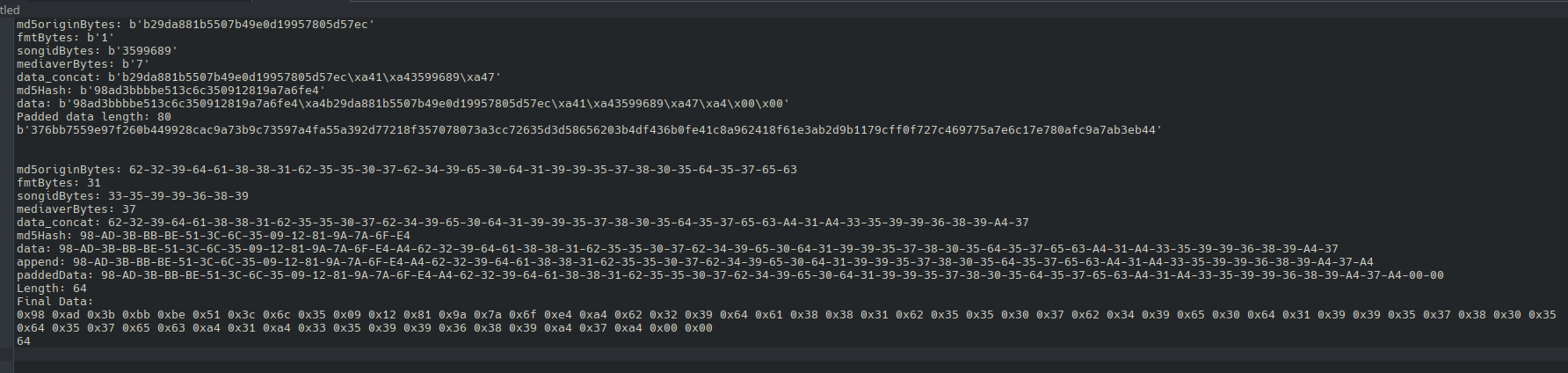
top is py bottom is c#
md5Hash and data are different between py and C# there?
Python, for some awful reason, renders bytes in a bytestring as ASCII chars if it can. So b'12' is not hex '12', it's hex '31-32'
That could just be a change in how you're printing those strings though, and since you haven't shared that code, I can't check
For python im just printing all variables with .encode and c# bitconverter.tostring
Python Bad on Gang
Could you please share your full exact code?
OK, so you genuinely do print the
data_concat
in a different way
Having a mix of str(...).encode()
and not is really quite confusing there!lemme make them all str.encode and rerun it
huh
Can you copy the C# strings as text as well, so I can compare, without having to open/close an image
What's going on there with data_concat, md5Hash, and data? The
b"b'....'"
?thats from the str(...).encode
atp i think python is giving you the wrong result :stare:
Ack, this is really frustrating, because you still aren't sharing your full code, so every time I spend time picking up on something, it's due to some code which you haven't shared
So I'm on the edge of just giving up -- I've wasted a bunch of time on this already
ok ill just share my functions and their results just straight up gimme a sec
I really need to be able to run both Python and C# side by side, and spot where in the process the difference is happening
But you keep changing what your MCVE is
ok
this outputs
https://dotnetfiddle.net/uyIoG1 is the c#
C# Online Compiler | .NET Fiddle
Test your C# code online with .NET Fiddle code editor.
Well there's one difference. In the python you do
data = b'\xa4'.join([md5hex(data_concat), data_concat]) + b'\xa4'
, but in the C# you do byte[] md5originBytes = Encoding.UTF8.GetBytes(track_hash);
Or wait, I might be confused
Ah no sorry, the C# version is md5.ComputeHash(data_concat)
So the python side takes the hex string of the md5, but the C# side takes the raw bytesshould i be doing
BitConverter.ToString(md5.ComputeHash(data_concat))
in the list then?
wait no
yup that was it
getting 376BB7559E97F260B449928CAC9A73B9C73597A4FA55A392D77218F357078073A3CC72635D3D58656203B4DF436B0FE41C8A962418F61E3AB2D9B1179CFF0F727C469775A7E6C17E780AFC9A7AB3EB44 from c# now
thank you sm and sorry for wasting your time on this lmfaoCool, glad you found it!
So the statement:
here is my starting bytes 98AD3BBBBE513C6C350912819A7A6FE4A46232396461383831623535303762343965306431393935373830356435376563A431A433353939363839A437A40000Was wrong?
looks like it was wrong
Yeah
Cool. I wasted about an hour trying to find that, based on the information you gave ><
Next time, please make sure that the question is correct!