Handling Third-party lib client component that relies on ENV vars.
Hi,
I am currently trying to add search on my project, and I stubbled upon a common scenario that I don't know how to handle yet...
I am trying to use
Meilisearch
with react-instantsearch
library.
I have this snippet of code, from the doc:
- I could do this... but I prefer avoiding sensitive keys on client if possible.
- I can create the searchClient
props on the server, but I can't pass it to a client component.
- I can't use the InstantSearch
component server-side.
How am I supposed to handle this type of Third-party problems where I have to create things client-side that depends upon sensitive keys?10 Replies
with a lot of hope and not the master key
from drizzle docs, the algolia search is using a client side key
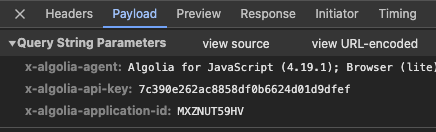
Still, isn't leaking client side key bad practice ? That could still be used to impersonate my access to a service...
is it leaking? no
it's designed that way
it's the risk of using a external source for that
other option is to proxy the request internally and securing it yourself, but you can't use the client thingy
in theory, with instant-meilisearch you can proxy it
and on
/api/my-meili-search
you make the safe request and handle the api thing
you just pay one more request per searchI just read that most of the time, you also need to actually control from where comes the request when using client side key.
The only bad design is to use them without ensuring who is actually using them.
But in environment when I don't control the components and the host (non-self-hosted content for example), I will have to make sure that this is handled correctly or find another solution.
i mean
in a perfect case, you would always use serverside and never show anything
but something you don't have a server and can only offer some kind of authentication for the data source via public key
sucks but it is what it is
I understand, I already had the sentiment it was the only way to do it...
But I did not understand why it wasn't as important with client side key.
most of them offer a read only kind of access
with meilisearch you have three keys
stripe you have two kind of keys, a private one to manage the products and more
a public one for the client to start orders and checkout stuff