Spring app doesn't read environmental variables from .env
I have .env file in my project root folder along with pom.xml. Contents:
and in application properties I use these values:
I even added Dotenv Dependency:
and configured it:
This class should set the system properties using the values from the
Dotenv
bean:
But actually it doesn't work and I get an exception when I launch my application.
I'm on Windows 10.9 Replies
⌛
This post has been reserved for your question.
Hey @Tomasm21! Please useTIP: Narrow down your issue to simple and precise questions to maximize the chance that others will reply in here./close
or theClose Post
button above when your problem is solved. Please remember to follow the help guidelines. This post will be automatically closed after 300 minutes of inactivity.
The exception tells that my database is inaccessible:
One of ways to succesfully launch the app is to set environment variables through Eclipse
Run Configurations...
in its Environment tab: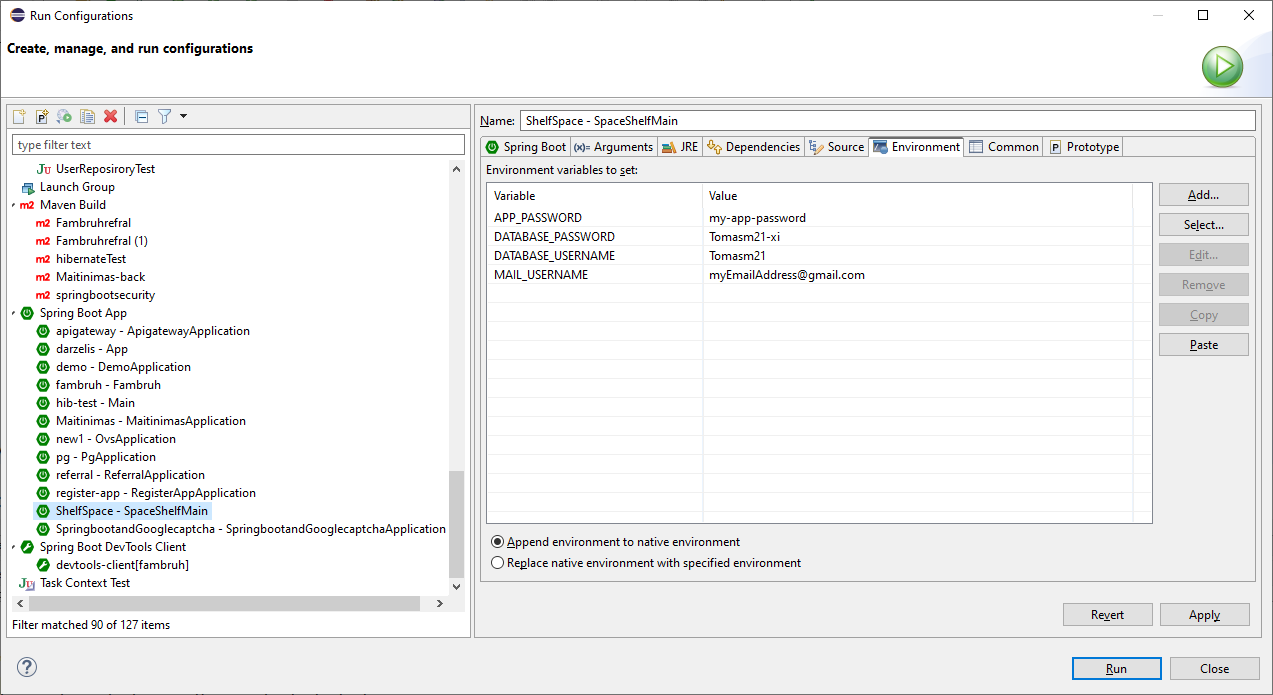
But it works only if I launch on Eclipse.
But the app fails if I launch it through CLI:
$mvn spring-boot:run
----
How to enable Spring Boot app to read my .env
and succesfully launch?
It can be launched as mvn spring-boot:run -Dspring-boot.run.arguments="--spring.datasource.username=Tomasm21,--spring.datasource.password=Tomasm21-xi,[email protected],--spring.mail.password=my-app-password"
But it does not solve reading from .env
problem.Unknown User•9mo ago
Message Not Public
Sign In & Join Server To View
well lets see
💤
Post marked as dormant
This post has been inactive for over 300 minutes, thus, it has been archived.
If your question was not answered yet, feel free to re-open this post or create a new one.
In case your post is not getting any attention, you can try to use /help ping
.
Warning: abusing this will result in moderative actions taken against you.
Unfortunately that article users answers does not solve reading environmental variables from
.env
file to the Environment specifics.
Fortunately I have found how elsewhere. And when using Spring Boot project in docker container too.Post Closed
This post has been closed by <@312509109863710732>.