Cannot read properties of undefined (reading 'roles')
I am trying to get the user's highest role and compare it to a role they are attemping to give another user through a slash command.
The command worked perfectly until I tried to add this checker, and now it's breaking.
The error I am getting is
TypeError: Cannot read properties of undefined (reading 'roles')
The line that is erroring is const senderHR = interaction.sender.roles.highest.position;
Full code:
31 Replies
- What's your exact discord.js
npm list discord.js
and node node -v
version?
- Not a discord.js issue? Check out #other-js-ts.
- Consider reading #how-to-get-help to improve your question!
- Explain what exactly your issue is.
- Post the full error stack trace, not just the top part!
- Show your code!
- Issue solved? Press the button!Unknown User•5mo ago
Message Not Public
Sign In & Join Server To View
i just replaced it with interaction.member and it still gave me the role even though it was higher than my previous highest role
if that doesnt make sense
i had member role, ran the command to give me the owner role and it gave me the role
it isn't supposed to give me the role if my highest role is below the role i am trying to give
- Bots cannot moderate (kick/ban/nickname/...) a target with a higher or equally high highest role or the guild owner.
- Bots cannot modify (edit/add/remove) roles that are higher or equally high compared to the bot's highest role.
- The
Administrator
permission does not skip these checks.thats
not what i was saying
Unknown User•5mo ago
Message Not Public
Sign In & Join Server To View
no but im going to be using this bot in my server to give people roles like staff and things for when im lazy
but what im trying to do
is if my role is say for example the moderator role and i try to give myself the administrator role
i dont want it to work
but it does give me the role
im trying to get it so the bot won't give me the role if my highest role is below the role im trying to give
:mdn: Greater than or equal (>=)
The greater than or equal (>=) operator returns true if the left operand is greater than or equal to the right operand, and false otherwise.
:mdn: Less than or equal (<=)
The less than or equal (<=) operator returns true if the left operand is less than or equal to the right operand, and false otherwise.
Unknown User•5mo ago
Message Not Public
Sign In & Join Server To View
it is but its set up correctly and the bot still gives the role
because youre comparing your own role position
Unknown User•5mo ago
Message Not Public
Sign In & Join Server To View
not the one from the member
also .getMember exists
ik the if part is messy i tried another thing
and it still gives the role
const senderHR = interaction.member.roles.highest.position;
that's the person that uses the command
im checking if their role is higher than the role they're trying to give
if its below the role theyre trying to give then it shouldnt work
Unknown User•5mo ago
Message Not Public
Sign In & Join Server To View
how
i took my roles
and im giving myself a role above the current role i have
and its still working
use this
how do i check whether it returned positive or negative
do i do something like
if (compareRoles(role.position, senderHR) >= 1);
give the role
like this
thankss
is there a way to make it send different replies based on that
i tried using else but it just gives me a constant red line under everything
Yes, your syntax is likely incorrect if that's the result
is this how i use it
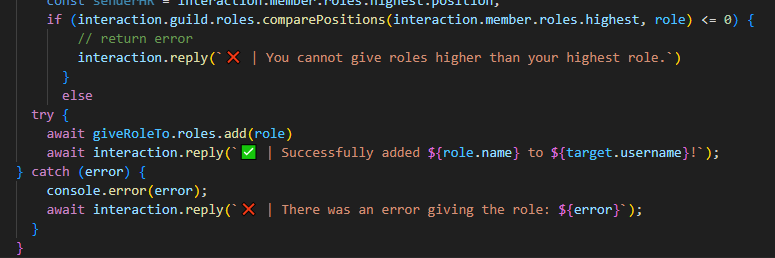
you know basic javascript right?
yes
it worked
tysm! :X_whitehearts:
add return before interaction.reply and remove else that doing nothing
i did and now its not responding when i run the command
add "else" then
it worked
thankss