Mocking fails for some reason
userService.findUserById(1L) fails and returns empty optional, any idea why?
74 Replies
⌛
This post has been reserved for your question.
Hey @Koblížkáč! Please useTIP: Narrow down your issue to simple and precise questions to maximize the chance that others will reply in here./close
or theClose Post
button above when your problem is solved. Please remember to follow the help guidelines. This post will be automatically closed after 300 minutes of inactivity.
You don't need to
isNotEmpty
, the hasValue...
already checks that
Show the UserService
Also this should definitely not be a SpringBootTest💤
Post marked as dormant
This post has been inactive for over 300 minutes, thus, it has been archived.
If your question was not answered yet, feel free to re-open this post or create a new one.
In case your post is not getting any attention, you can try to use /help ping
.
Warning: abusing this will result in moderative actions taken against you.
sorry im kinda new to all this testing shit, so im just tryint to figure this out, id be really glad if you showed me the way how to do this lol
user service is:
ok, so you should inject PasswordEncoder not BCryptPasswordEncoder
You should have a config bean for PasswordEncoder that returns BCryptPasswordEncoder
So you use the same one everywhere
well, thats just a little thing, im more worried about why the test isnt passing
Remove all those annotations from the test
And just run with @ExtendWith(MockitoExtension.class)
From the test class
it doesnt let me autowire the userservice then
That doesn't make sense. This is how I always do it
Show the new test code

yes, don't autowire it
Just InjectMocks
OK, little bit of explanation you seem to be missing
You're trying to test business logic, in this case a service
So to test it, it might need dependencies, like your UserRepository. So the ONLY thing you need, is a mock for the repository, that returns the right stuff you need to test your userservice
So you don't need any other spring stuff
This isn't true for other types of tests, for example, testing the HTTP api, or the database, because they need specific spring stuff to test
And you should test those with specific test slices
https://docs.spring.io/spring-boot/appendix/test-auto-configuration/slices.html
Instead of SpringBootTest
Because that will start your entire spring application basically, for no good reason
hmm yes that really makes sense, its now almost working as expected, but its throwing NPE because passwordencoder is null for some reason
yes, you didn't mock it...
so now its loaded only what is mocked?
if i understand correctly
yes
so i suppose i should make separate configuration and load it
no
UNIT test
Just mock it
That's also why I said use PasswordEncoder
Because that's an interface
right, but how do i mock it if i dont know what will it would return?
It doesn't matter for your test what it returns
so like
when(passwordEncoder.encode("Password1")).thenReturn("hashedPassword");
is good enough?What are you doing when you do that?
You don't need hashedPAssword do you?
i placed it into the saving test
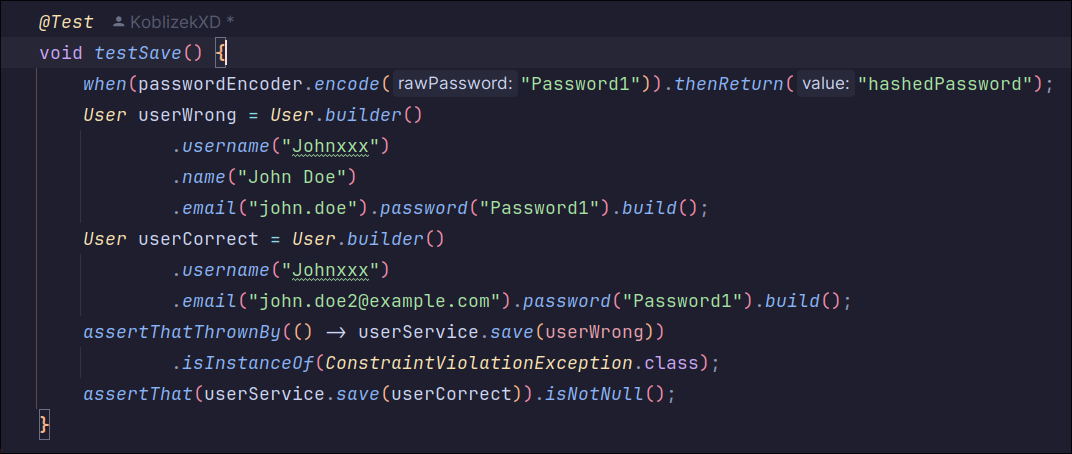
Yeah, you don't use hashedPassword
So remove the when
haha, and userWrong and userCorrect is exactly why I hate builders
Goodbye compile time safety
thats why i like kotlin
You made a builder
yes
Just don't make a builder...
Constructors exist
You're using builders as a vague way to get named parameters
... you told me to mock it, and now telling me to remove the mock?
mocks return default values
But a mock is not null
So you have a passwordencoder at that point
so your service doesn't call a method on a null object
You don't need to give it logic, unless you need to
so adding
is enough?
Yes, if your USerService takes a PAsswordEncoder as a parameter
yup
Then you declare in a Configuration class somewhere
yes i already changed that
youre true it wouldnt make sense to return a concrete class and interface is more suitable for mocking lol
Test works now?
the userWrong for some reason gets saved

I like doing it this way btw
But up to you
ah yes, because who checks the validity?
im pretty sure annotating the component with @Validated and @Valid on method parameter is enough to check the validation automatically
atleast i read it on docs
somewhere
for sure
You shouldn't validate on the service btw
That's the controller's job
yeah, you shouldn't do that in general?
Unless this is like a new thing a lot of people do
But Controllers handle the IO, they convert your web request from json or whatever to an object, that get's validated and passed to your service
So put a @Valid on the @RequestBody in the controller
ah okay
so i shouldnt validate in services
good to know
no
okay i removed the validation
but now the saving fails
aha

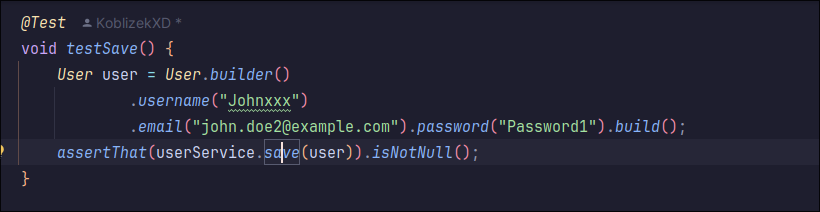
with?
means your save method doesn't return the value
Because the repository save method doesn't have an implementation in your mock
you can have it return the first parameter with thenAnswer for example, or just verify that you call the save method
I would also verify the passwordEncoder method is called
it is but returns null...
therefore i will need to mock it
indeed
Unknown User•10mo ago
Message Not Public
Sign In & Join Server To View
sorry i already solved it but i kinda have a dilema over these 2 things, which should i choose:



Unknown User•10mo ago
Message Not Public
Sign In & Join Server To View
because maybe i want to pass some default permission to the user?
@tjoener im tryint to test this, but its not working again, any idea why is that so?
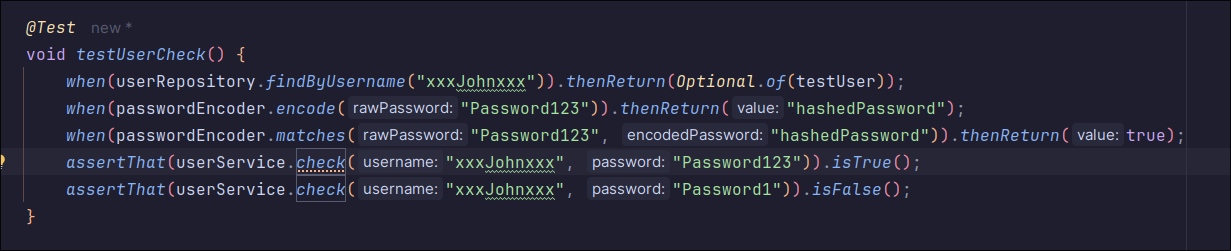
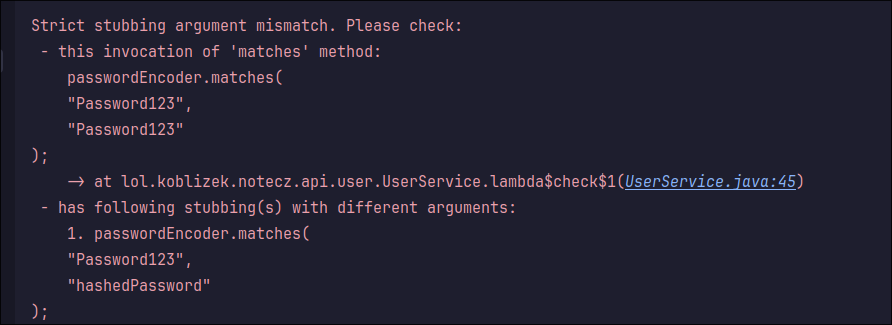

Unknown User•10mo ago
Message Not Public
Sign In & Join Server To View
?
Unknown User•10mo ago
Message Not Public
Sign In & Join Server To View
no
Unknown User•10mo ago
Message Not Public
Sign In & Join Server To View
i dont want to spend like 10 hours watching guides on how to create tests bruh
Unknown User•10mo ago
Message Not Public
Sign In & Join Server To View
thats true
Unknown User•10mo ago
Message Not Public
Sign In & Join Server To View
it makes sense though in my head, i mock whats supposed to be mocked, and then i test the service layer
Unknown User•10mo ago
Message Not Public
Sign In & Join Server To View
i mock repository and pwd encoder
Unknown User•10mo ago
Message Not Public
Sign In & Join Server To View
im leaving for a wekk tommorow, so i might check out some guides while bored i guess
@Agit Rubar Demir any guide you would recommend?
Unknown User•10mo ago
Message Not Public
Sign In & Join Server To View
well during that time i wont have laptop with me
i meant rather like watch only things
for like theory or smth
so atleast i know what im writing
Unknown User•10mo ago
Message Not Public
Sign In & Join Server To View
Wait who's helping now
Always try to return immutable collections (Set.of in this case). Having immutable types makes it easier to reason about your program
💤
Post marked as dormant
This post has been inactive for over 300 minutes, thus, it has been archived.
If your question was not answered yet, feel free to re-open this post or create a new one.
In case your post is not getting any attention, you can try to use /help ping
.
Warning: abusing this will result in moderative actions taken against you.