✅ Waiting on a task more efficiently
I've made this class for my application that needs to process some things in the background using a queue:
Is there anything to do to better optimize the while loop when no jobs are in the queue? I've done a simple 1s delay but I'd bet there's a better way of doing it..?
7 Replies
Actually I thought of using a semaphore to do it:
If you have no further questions, please use /close to mark the forum thread as answered
$close
If you have no further questions, please use /close to mark the forum thread as answered
rip
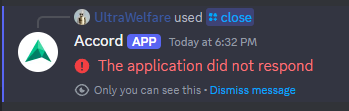
lmao xD
well at least its clear now for others that this is already resolved ;p