Get type of prisma client before it is defined
In my next.js app I have to use the following approach to prevent multiple instances of PrismaClient being created
The above approach however is problematic because it doesn't generate the correct PrismaClient type. E.g. in my project TS will complain that
db.user.example
doesn't exist.
I tried solving this with typeof new PrismaClient().$extends(extension)
but I need globalForPrisma
to be defined before the PrismaClient has been defined10 Replies
I use the following solution in a file called
prisma.ts
where I then import my client from:
Thank you! I found an alternative solution without having to use declarations (someone told me they're unsafe).
I am getting this error though
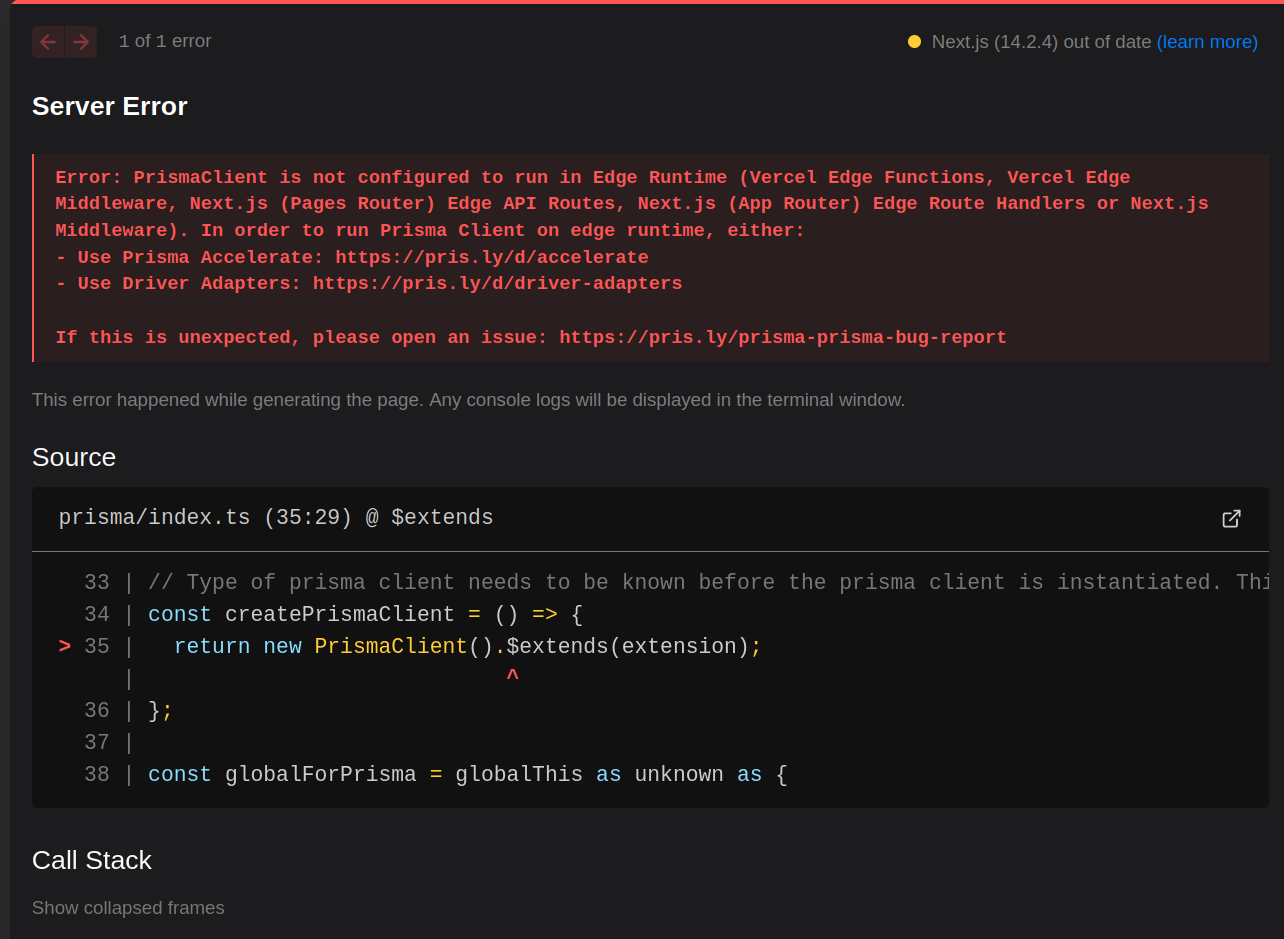
Are you trying to run prisma in the middleware? That doesn't work since the middlware is run on the edge runtime which Prisma can't do
This isn't on the middleware, it's in
prisma/index.ts
.
This error doesn't happen if I remove the createPrismaClient
function. e.g.:
No Error:
Yes Error:
Then try my code. I don't know why it would be unsafe to use it.
Your code has the same problem as mine though, since you are setting
prisma: PrismaClient
if you want your prisma client to have an extension it will be of the wrong type.
eg:
You see, we have added an extension
to PrismaClient
but the type of prisma
is still PrismaClient
, so like if our extension has a method then typescript won't know about it
Ideally we can somehow set var prisma: PrismaClientWithExtension<typeof extension>
or something like that. Not sure if it's a thing thoughYou can create a type which has all the additional typing and then do
That's a cool idea but I tried it and it doesn't work unfortunately.
My extension is like this:

What actually happens is TS thinks that
db
has db.model
which shouldn't happen