✅Shuffling db results using LINQ.
Is there a way to shuffle db results using linq?
I've tried copying some code from online using .orderby(...) but it doesnt seem to work.
I have this code that returns all words from a db, shuffles them all then returns just the first x but it's horribly inefficient.
13 Replies
well if the shuffling is the slowpoke why not show the code of shuffle?
uh
why not let the database shuffle it?
its probably faster than doing it by LINQ
Sorry I wasn't clear. I mean the shuffle is shuffling all of the words from a category but I only need to return a few words.
I didn't realise this was a thing. Just looked at sqlite3 doc and found order by random(). I'll try to figure it out after my lunch. Thank you
yep
that'll do it
What was your original shuffle implementation out of curiosity?
The horribly inefficient one?
I just copied it from stack overflow. In the orignal quesiton I meant shuffling the whole list of words was ineffcient, I would prefer to just shuffle the amount of words I needed so its like worst case 3^2, instead of 10^2.
Ok, that's Fisher Yates which is about as efficient as you can get if you need to shuffle the entire list.
Yeah I did skim the wiki page on Fisher Yates but I haven't sat down to figure out what it's doing
Also, this seems like total premature optimization 🙂
The number of users will be less than 1 so its all premature lol
Fisher Yates shuffle of 100,000 items takes like 1 millisecond.
I will still try to figure out shuffling in the db query instead, but will do it later.
Getting close to a working single player mode now. Quite excited 🙂
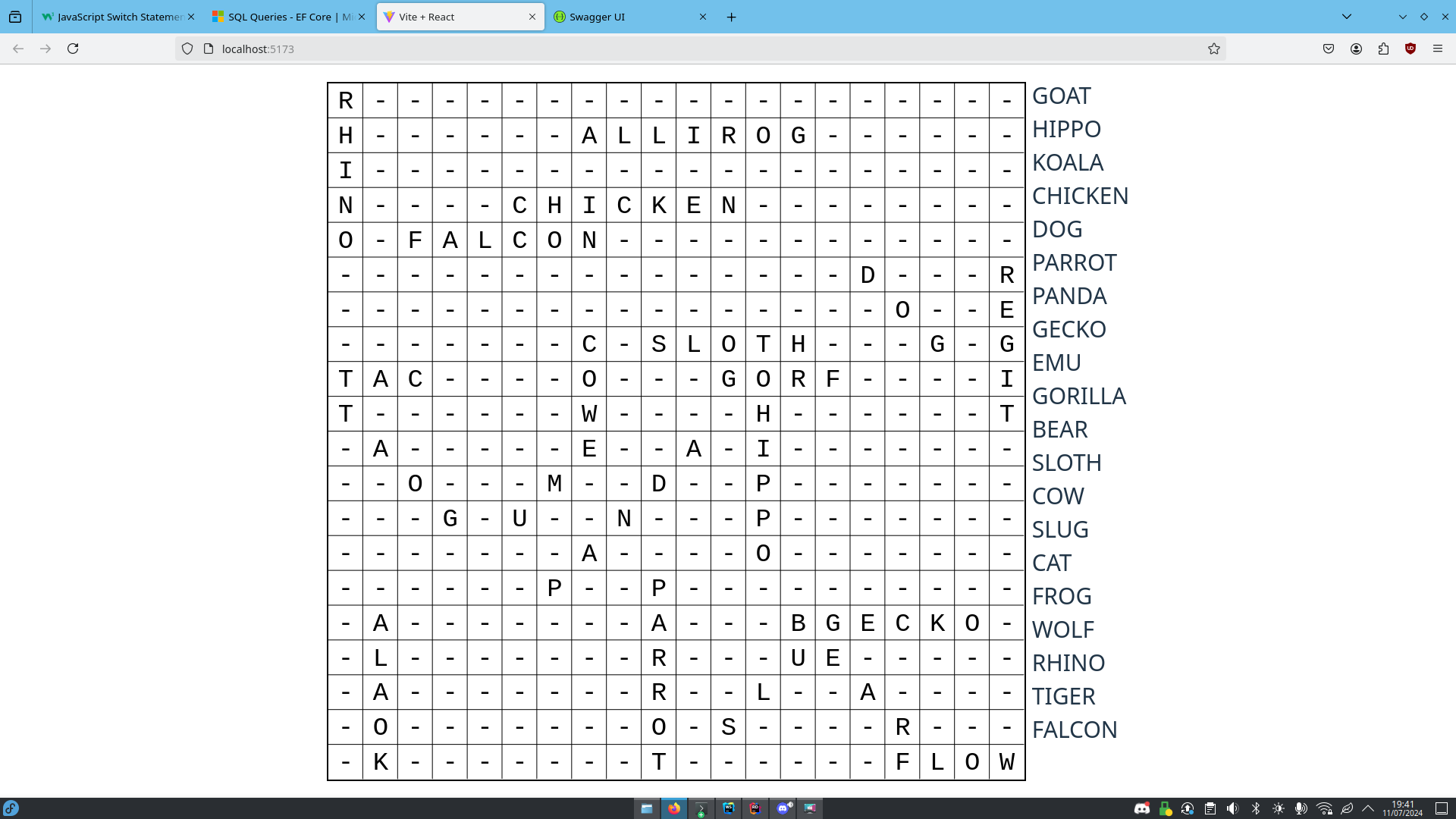