Unable to catch the disalowed intent
I constantly fail to catch the missing intents. How can I catch this error?
17 Replies
- What's your exact discord.js
npm list discord.js
and node node -v
version?
- Not a discord.js issue? Check out #other-js-ts.
- Consider reading #how-to-get-help to improve your question!
- Explain what exactly your issue is.
- Post the full error stack trace, not just the top part!
- Show your code!
- Issue solved? Press the button!error:
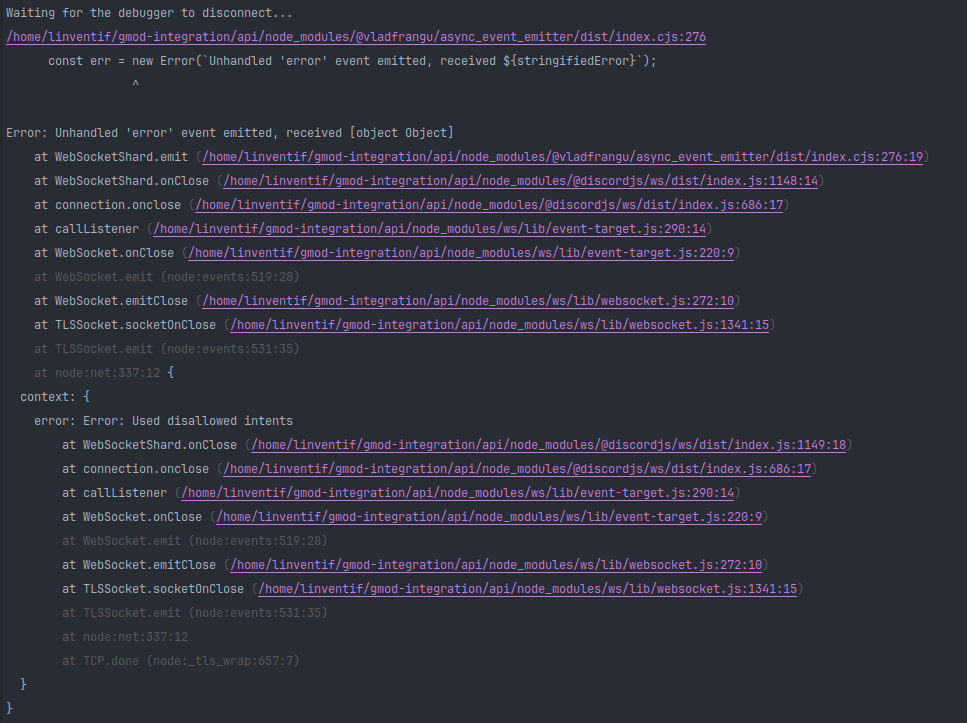
-
Error [DisallowedIntents]: Privileged intent provided is not enabled or whitelisted.
- Error: Used disallowed intents
If you are using the GuildMembers
, GuildPresences
, or MessageContent
intents, you need to enable them via Developer Portal > Your app > Bot > Privileged Gateway Intentsyup I know
but I want to catch the error
actually it just block my code
GitHub
Regression starting from v14.10.0: "disallowed intents" is not catc...
Which package is this bug report for? discord.js Issue description If you run client.login() specifying privileged intents (like GuildMembers) while the bot doesn't have permission for that, No...
Perfect example of why attempting a global anti-crash is bad
so no way to catch it ?
Not currently
ok I think I have a idea with child process than
yup I did it
Why exactly do you require this solution?
custom bot for a saas, the other solution will be docker with dynamic nginx config
So they'll be providing their own token, and you're building error handling around it being set up incorrectly?
👍
I'd probably simply refer them to setting it up according to documentation, but I guess that makes sense
yup but I can't let 1 user kill a multi user process