How do you understand this code, BinarySearch Delete
Is my guess correct?
6 Replies
⌛
This post has been reserved for your question.
Hey @Rag...JN 🌌 🦡 👽 💰! Please useTIP: Narrow down your issue to simple and precise questions to maximize the chance that others will reply in here./close
or theClose Post
button above when your problem is solved. Please remember to follow the help guidelines. This post will be automatically closed after 300 minutes of inactivity.
So if delete 50, the new root will be 60?
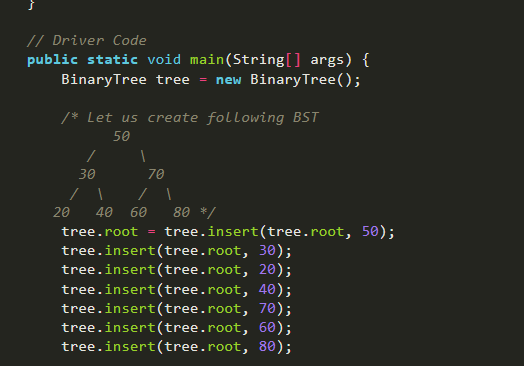
Aight
I figured it out
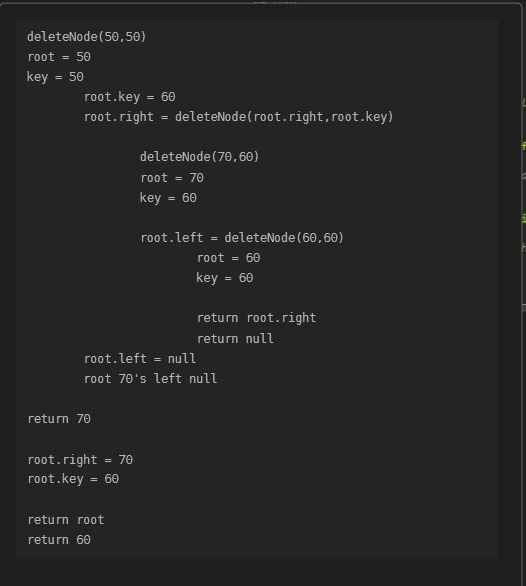
💤
Post marked as dormant
This post has been inactive for over 300 minutes, thus, it has been archived.
If your question was not answered yet, feel free to re-open this post or create a new one.
In case your post is not getting any attention, you can try to use /help ping
.
Warning: abusing this will result in moderative actions taken against you.