Can you send an ephemeral response from a public slash command?
^
Solution:Jump to solution
```kotlin
check {
val user = userFor(event)!!
val usage = usageService.checkUsage(user.id)
event.extraData["usageResponse"] = usage...
63 Replies
the discord API accepts that and it's exposed via
respondOpposite
, but it probably won't actually be sent as ephemeral
what's your use-case?It's a public slash command, public response is fine, but if there is an error, then I want just the user who sent the command to see it.
what kind of error did you have in mind?

could you do that check in a check instead?
wdym?
using the checks system
Kord Extensions Help
Checks | Kord Extensions
just write a check that fails with an error message
Okay I'll try that thanks
checks don't have access to command arguments
if you need that then you'll need to do something else
Ugh that causes problems, because I'd need to hit my database twice
that's what the event context is for
Because I output the remaining quota in the message too
https://docs.kordex.dev/events.html#custom-context
event.extraData.set("key", yourData)
event.extraData.getOf<YourDataType>("key")
see, I think of things /lhI can't acess
user.id
within the checkyou can get it from the event object, or use
userFor(event)
that function is also detailed on the checks pageSolution
lgtm
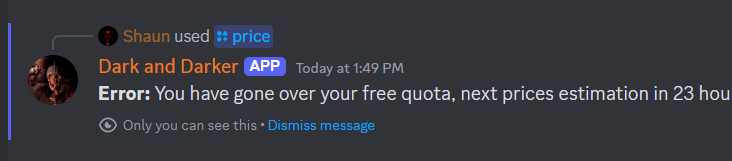
Can you dm slash commands to the bot?
yeah, if they're global
I need to reopen this as I'm back on this problem again.
I need to send an ephemeral response to a command in some case, but the response needs to be customized and have some components on it.
Othertimes the response will be public
Would be neat if it were something like
This works but the extemsion already responds so it errors
the unsafe module provides a command type that allows you to handle responding to the interaction yourself
it's not documented (yet) but it's not that complex to use
How do I stop kordex from responding
you have to use the unsafe commands
In the extension?
within the action?
what?
are you already using the unsafe module?
No
then you need to use it
Any docs/examples ?
it'll give you an
unsafeSlashCommand
which allows you to set (or disable) the initial response
well you need to add the dependencyIs it your dep?
yeah, it's the same dep but the artifact ID is
unsafe
you need bothUnsafe feels such a strong word here 😂
manualSlashCommand
it's the correct word
how do I do this with toml
add this?
yep
Dependency alias 'kord-unsafe' is not used in build scripts
you do need to actually use the alias in your build script yes
I really don't see the point in the toml file
libs.versions.kord.unsafe
in your case I think
it's more useful for multi-module projects
and also for linters and dependency checkers
eg dependabot on githubShould I be excluding dev.kord from unsafe too, while I use the newer kord version
checking
no, it should be fine
I'm not using API scoped dependencies in that module
Okay so how do I respond with an unsafe slash command?
I see respondEphemeral and respondPublic, I'm guessing its those
no
first of all, you need to set
initialResponse
in the command builder
to InitialSlashCommandResponse.None
in your case presumably
in the action
, you'll need to use one of the ack
functionsIf I used PublicAck then would the bot say its thinking, then I wouldn't need to acknlowedge further down
it uses deferPublicResponseUnsafe, I forget whether that shows the thinking message or not
you can always test it
then how do I respond
you need to ack first
the ack function also allows you to set an inital response content
in the builder
after you ack, you use the corresponding response function
initialResponse = InitialSlashCommandResponse.PublicAck
doesn't ack?it does, but if you use that then you may as well use a normal public command
It seems to work
the point of unsafe commands is to make it easier to change the response type dynamically
but in doing so, you are accepting that things might not work how you expect them to if you don't do what discord expects
I want to always public ackknowledge and then respond privately/publicly
Which is different to the publicSlashCommand beacuse it tries to ack twice
you simply can't do that
It works?
for now
haha
the ack types do matter to discord
if you start with a public ack and then send an ephemeral response, that's undefined behaviour
the API may break it
How do I set a command argument to be optional and set a default value?
defaultingXyz {
And what goes in the body
read the converters page in the docs
Kord Extensions Help
Converters | Kord Extensions
there's even an example in there