Same Tree LeetCode
What am I doing wrong here
17 Replies
⌛
This post has been reserved for your question.
Hey @Rag...JN 🌌 🦡 👽 💰! Please useTIP: Narrow down your issue to simple and precise questions to maximize the chance that others will reply in here./close
or theClose Post
button above when your problem is solved. Please remember to follow the help guidelines. This post will be automatically closed after 300 minutes of inactivity.
Failing test case
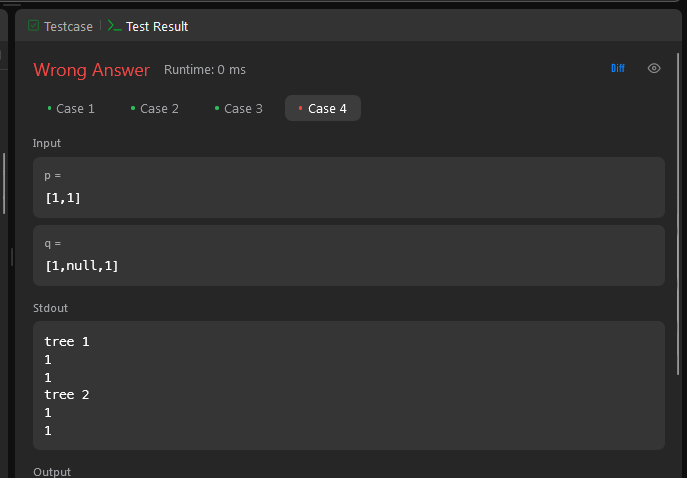
You're flattening the trees into Lists, then comparing the Lists
It could work if you ensured the List you flatten to were canonically representing the trees, but you don't. You produce the same list with a missing left node and a missing right node.
yah how to canonically represent the tree?
You could do it the same as they do in the input part
Here when there is a missing node in the middle they insert null
yah I tried that then also it generated the same tree
The same list you mean?
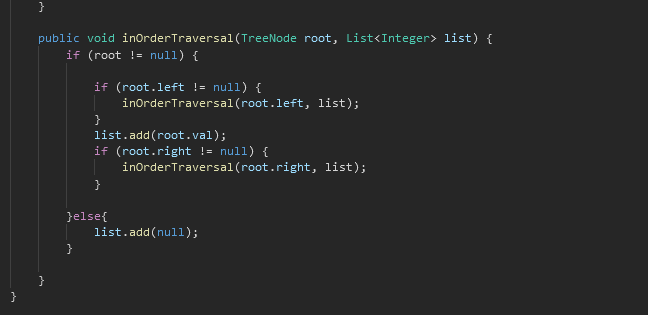
yah it turned out a same list
That's not an attempt to add nulls. You just modified where you insert the root
alright I figured it out
I just noticed your list is not like the one they put in input box. I'm not sure it will be canonical this way
is the better approach?
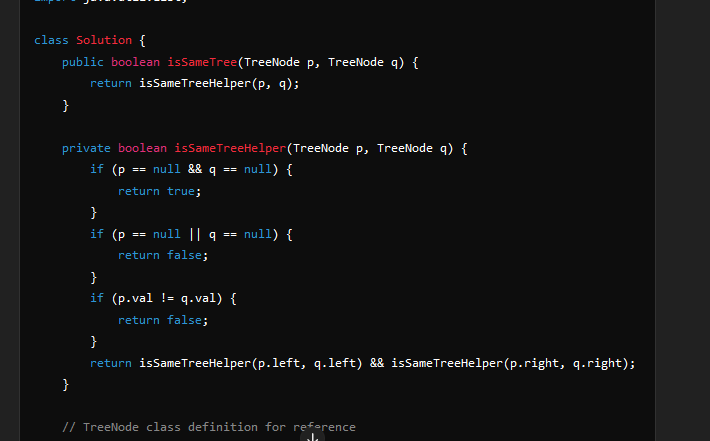
This reaches till the end of the leaf node of one of the tree right
Then it check if the values are null, one is not null or no even
I mean, it's the most direct approach. Trees are recursive structures, so it checks recursively and directly.
💤
Post marked as dormant
This post has been inactive for over 300 minutes, thus, it has been archived.
If your question was not answered yet, feel free to re-open this post or create a new one.
In case your post is not getting any attention, you can try to use /help ping
.
Warning: abusing this will result in moderative actions taken against you.