Resolving "No EtherCAT Slaves Found" Error on BeagleBone AI with Embedded Linux
hey guys, am developing a real-time industrial control system using a BeagleBone AI with Embedded Linux. The system should read data from an EtherCAT torque sensor. I have verified the
EtherCAT slave
connection and settings, checked the network interface and cable connections, made sure the EtherCAT library is correctly installed and configured. But am getting the error No EtherCAT slaves found
.
this is my code
@Middleware & OS13 Replies
Hi @Boss lady make sure that the eth0 interface is up and configured correctly. You can check this using the
ifconfig
command. Ensure the interface has the right IP settings for EtherCAT
communication. Also, verify that correct drivers for your EtherCAT
master are loaded. Some configurations may require specific kernel modules, you can check which modules are loaded with the lsmod
command.@Boss lady You should use the ethercat command-line tool to debug the EtherCAT network. This tool can provide more insights into the state of your EtherCAT network. Run the following to check for connected slaves:
Thanks @RED HAT @Enthernet Code I confirmed that
eth0
is up and configured correctly. I also checked the loaded modules, and everything seems fine. But running the application with sudo
didn’t change the outcome.It seems like the problem might be with the network configuration or the way the EtherCAT library is initialized. Make sure your network configuration does not interfere with EtherCAT communication. Also, ensure the
ec_init
function is correctly initializing the network interface and you could add some debug prints in your code to ensure that the initialization steps are executed as expected. Modify your code like this to get more details:
I added the debug prints and found that the initialization of the EtherCAT interface is successful, but still no slaves are found. It seems like the
ec_config_init
function is not able to detect the slaves.@Boss lady If the initialization is successful, but slaves are not detected, it might be an issue with the slave configuration or compatibility. see that your
EtherCAT
slaves are powered on and properly configured to communicate with the master and check the slave compatibility with the EtherCAT
library you are using. Some slaves may require specific configuration or firmware versions to work correctly with the master.@RED HAT I have checked the slave configuration and compatibility, and everything seems correct. But still no success.
@Boss lady Since all seems fine, it might be useful to try an alternative
EtherCAT
library or tool to cross-verify
the hardware setup. try using a different EtherCAT master stack
to see if it detects the slaves and let me know the outcome with the different EtherCAT master stack
. It can help pinpoint whether the issue is with the current library or the hardware setup
.Okay
@RED HAT I tried using a different EtherCAT master stack
(IgH EtherCAT Master)
, and it detected the slaves without any issues. It seems like the problem is with the current EtherCAT library(Simple Open EntherCAT Master)
I was using.That’s good, At least you know the hardware setup is fine, you can either continue with the new master stack or try to troubleshoot the original library further. If you decide to stick with the original library, consider reaching out to their support or community for any known issues or patches. Otherwise, the new stack should serve you well for your project.
Thanks @RED HAT I’ll proceed with the new master stack for now and reach out to the original library’s support for any known issues. I appreciate all the help
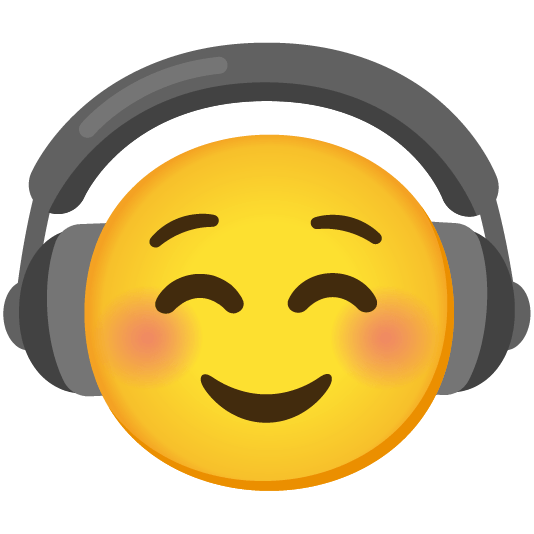
Good luck with your project and feel free to ask if you run into any more issues. Happy coding!