eventListener on click
I'm dealing with a "simple issue". Basically, i want to put my code inside a function instead of passing directly in the button, but i'm having a issue where i don't know exactly how i'm supposed to do it, so. Here's the html, very simple:
the js:
34 Replies
I do believe i'm overthinking about something more simple than it seems to solve
you're not only overthinkinging but you're misuing it
you can delete your entire javascript and just add the
required
attribute to the fields
BUT!!!!!
you have to put everything inside a form, as you should have done from the beginning
and the button should have the type explicitly set to submitoooh
Definitely what epic said, but to answer the actual question, when you're passing in a function like to an event listener, you need to omit the calling brackets where you pass the function reference.
So this
i tried without but still wasn't working
so this made me have a little mentalbreakdown for a few seconds
if was it wrong or not
since the logic is right and is just to show a simple alert
you have 2 different clicks
it was just an example of the right version, but once i do like this
doesn't work
idk
can be like bcs of the order in the script?
By adding the (), you're telling Javascript to run the function and pass the result to the listener. The event listener should do the calling, so you just pass it the reference to the function to run
like the function going after
or ?
this
Make a codepen or something then, I can't run code in my head
ok wait a sec
what jochem said is supposed to work
I'm on mobile atm though so I can't look too much further. I'll look when I can if epic hasn't solved it already 🙂
you already solved it
checkButton.addEventListener("click", checkIsEmpty)
<-- this is the solutionoh wait, i just had to actually put my function before
like before i used in the eventlistener idk why tho, it worked before the other way around
that's not strictly a function, that's an anonymous arrow function
it's important to keep that distinction because there's nuance to it
if you just did
function checkIsEmpty ...
it would workThis way wasn't working:
Like this works:
you can put the function anywhere, since javascript is weird
because you're using a constant before it is defined
yeah prob is a issue with the platform i'm using on the browser than
that's what made me got confused
i literally just had to put the function before the eventListener
weird
this works as well
no, it's just you confusing yourself over javascript's weirdness
which is normal
it's not a function, very strictly speaking: it's an arrow function assigned to a constant
yeah like why wasn't working when i put the checkIsEmpty after declaring the eventListener?
idk
it's treated completely differently in some aspects
this just makes my mind a little lost xD
i just explained why
so basically js is just weird
ok got it
well, yes and no
Defining an anonymous arrow function declared the function in place, and it's available to any code that runs after the arrow function definition.
Declaring a function anywhere in your script with the function keyword hoists that declaration to the top of the file so it's available in the entire script.
^ this
oh okay
i got it now
thanks guys
this helped a lot
you're welcome
also, be VERY careful when using arrow functions, as the
this
variable won't changetake this code as an example:
the first one will be referring to
window
the 2nd one will be referring to <button>
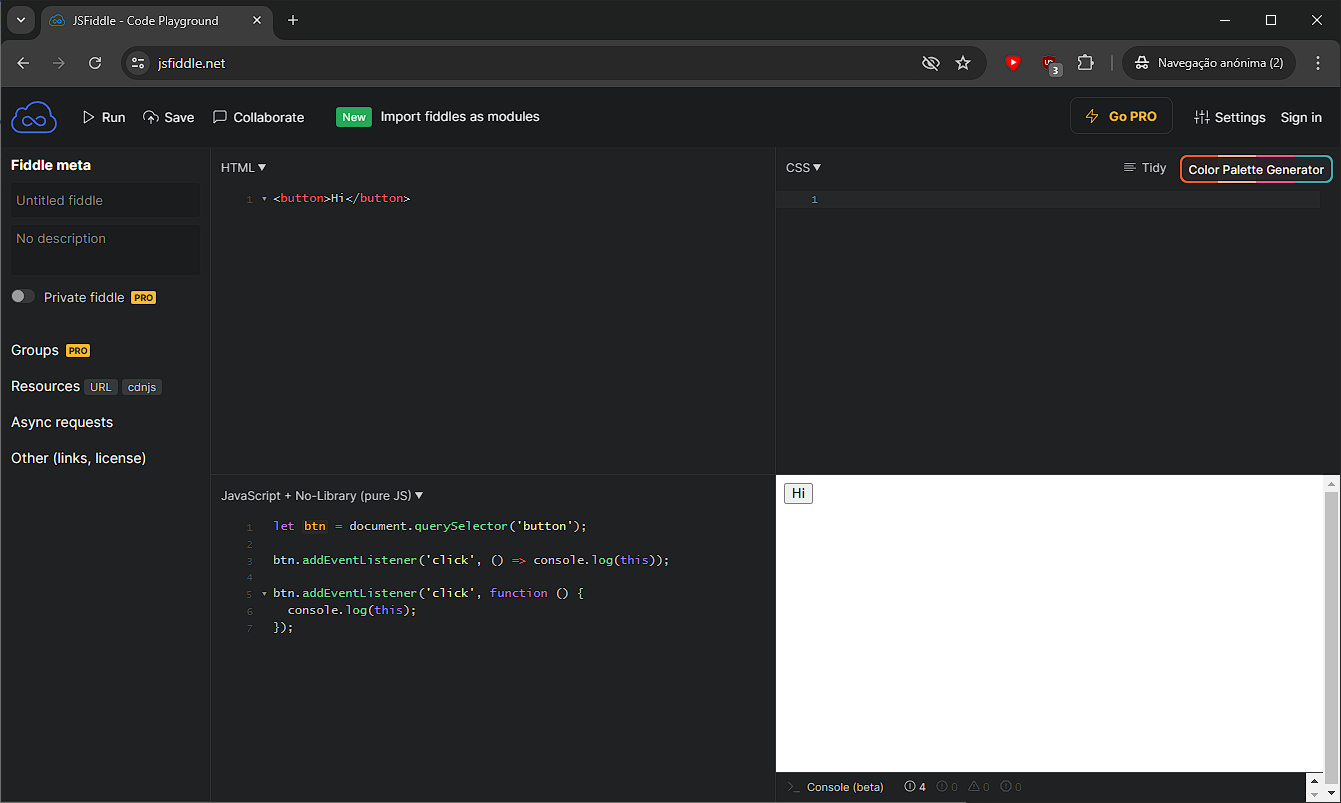
Your last line of code has an error. The second parameter of addEventListener() must be a function. What you provided is a function call and not a function. The result is that the parameter is actually the value undefined, because it calls checkIsEmpty and that function doesn't return anything. The parentheses after checkIsEmpty calls it. Those parentheses must be removed to pass the function as a callback.
oh yeah, i put bcs wasn't working when only passing without the () but i just had to move the const above
and it worked
without the () ofc
thx for the help still, is always good to learn