standard output from Process not giving any output for certain commands
I am using C# process to run some processes. The shell is
cmd.exe
, and running several types of scripts work. But, when I try to run python idle with the command cmd.exe /C python
, it is giving no output and the process is also not exiting. It should have displayed the idle texts like it do on a normal terminal.
The task function in which I am writing the output:
Is my approach wrong? Thank you!56 Replies
Hey @Abdesol
When you run 'python' from
cmd.exe
, it typically starts Python in the interactive mode if you're in the terminal but may behave differently when started from another process like through C#. So just make sure you calling the right executable. Another option if the above doesn't work is just to redirect the input and output and potentially the error streams.
Your method for reading the output looks correct from my stance, just make sure there is output to be read thoughI think that is the problem as well.. running python.exe is not giving any output, maybe cuz it is interactive
but powershell works, but when I run wsl, I don't get the first color full part of it.. I mean the path of files is some how colorful and it is not being captured by the program
I am redirecting the error as well.. it uses this function and runs on a separate task
but it doesn't look interactive to me

Ok wait, I am not sure what your goal is here @Abdesol
I am basically building a simple terminal. I have got many things sorted out, but the problems now are:
1. python and some commands are not giving output at all
2. some commands like "git clone", which show progress on the same line of console, instead of a new line, I am not able to capture those lines
If you wanna run python scripts, you can run the a file with the script in it...if you wanna see colors then emulating a terminal will help
with files/scripts, it works well,
I know handling an interactive processes in a simple terminal project is not going to go easily
but that python doesn't look interactive..
the main problem right now is the second one here
Yeah it would, because you running python the way a user will logically speaking
yeah, there is no actual need to run the idle
It will run python code...and has it's set of memory.
If you open cmd and type python, then create variables and print them it will show output
yeah, on cmd and powershell it works, it is just not working in my code
this is my terminal and it is stuck like this when I run the python command
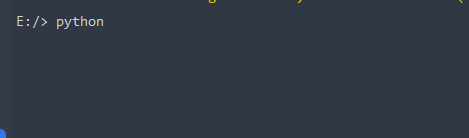
But going back to your core issues.
For interactive commands like Python, the issue is often related to the command's environment detection. Python, when launched in interactive mode, expects an interactive terminal. When it detects that it is not attached to an interactive terminal (like when standard output is redirected in a C#
Process
), it might behave differently
Try running python scripts in non-interactive modes, you can execute the scripts with predefined inputs, or using -c
command to execute a piece of python code.
Additionally you can look into 'pseudo-terminal'. It's a little complex in windows but can be partially simulated using third party libraries like wimpty
or something names 'Console Terminal' (I forgot it's name but something like that).ah, interesting, I understand the problem now.. but for the second issue, what do you have to say about it?
like when running a git clone command, it doesn't go beyond this, but it is actually doing the job and did actually clone it
it finishes just like his
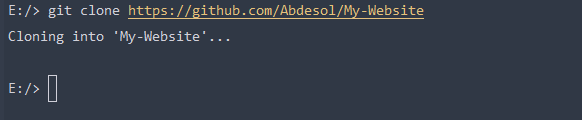
With regards to
git clone
, commands like that output progress dynamically by rewriting lines. It uses \r
as carriage returns intend of \n
and some standard output redirects may not handle that well. So you can either read raw output (which I like to do most of the time) and when you detect \r
then treat it as an instruction to overwrite the current line in your output display.
busy typing lol, I take some time give me a minute lol.hehe, thank you 😅
let me try to remove any
/r
from the output provided and see if it prints everything first
not working this wayFor the git I can give you some code as I have done this before:
So you basically checking characters and when it finds the
\r
it will then reset the line buffer.oh interesting.. let me check it
rq
@SparkyCracked even with your code, by replacing it above in my function, I faced the same issue again
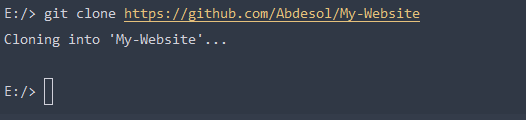
I tried adding Console.WriteLine("Here") in the if condition and it was not printing that.. I think there was no /r text ig
Yeah...tough. Are you able to get the raw outputs? Put a break and see what the variables are
okay
weird problem
after it finished the first line
i.e.
Clonning into 'My-Website'...
It just ended?
EndOfStream got to tru
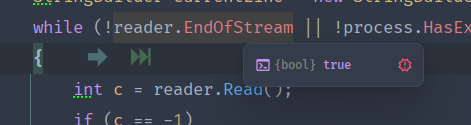
yep
this is the function where I am dealing with the process and the tasks.. is there anything I am missing here?
It looks fine from my side, I don't see any issues
for windows no suffix, and the prefix is
/C
and shell is cmd.exe
There might be a potential race condition where the process exits before all output has been read. To mitigate this, consider awaiting the I/O tasks before awaiting
process.WaitForExitAsync()
. This ensures that all output is captured even if the process exits quickly:
oh, interesting.. let me check rq
Here is some documentation on race conditions
Race conditions and deadlocks - Visual Basic
This article describes that Visual Basic offers the ability to use threads in Visual Basic applications for the first time. Threads introduce debugging issues such as race conditions and deadlocks.
I've recently been diving deep into tasks and threading so yeah
okay will check it out
I tried putting a console write line outside the while loop and the problem is still around
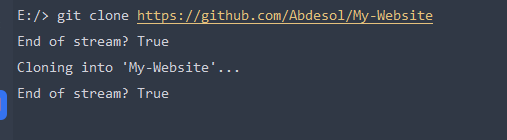
the first one is from the error output, and the second one is from the actual output
actually, I just checked and the error task is the one responsible for the git clone output
For me I want to see if you ever have more than that one in your code at any point. So when you step in your code, once you print out 'Cloning into...' hover over the variable and see what it's contents are
may I share the code? it is just a one file code
Ohh fair, if you comfortable with that...
yeah, I will probably make this simple terminal public if it gets to work well
what was the way to share it? binpath?
could you share the link
$code
To post C# code type the following:
```cs
// code here
```
Get an example by typing
$codegif
in chat
For longer snippets, use: https://paste.mod.gg/You can use the above link
BlazeBin - bzkdrnmwuvvs
A tool for sharing your source code with the world!
@SparkyCracked
sorry not this one ig
BlazeBin - mxifcyrdlkeu
A tool for sharing your source code with the world!
that is the old one
Ok cool
btw, you have to click enter to get back to the path part
that is an issue for later
@Abdesol Some progress...
I've located a potential issue where the output capturing from the process in C# is not handling the asynchronous nature of the
git clone
operation correctly.
It's super weird so I am gonna try see if I can read the outputs properly and prevent early termination of the process (I mean git still clones cause my massive project managed to clone so it works which is good)great!
and yeah, it does actually clone, just doesn't show the progress bar and the lines that come after that
Ok @Abdesol
I can't lie, it may take me some time to figure this out. I managed to get it to the point where it exits the cloning process when done and waits for input again by itself but the actual printing of output is a little tougher. I don't understand the reason it is bombing out as of yet
ah, I really appreciate your cooperation and absolutely no rush
@SparkyCracked Sup, any progress?
Yoo wassup
i capped myself to 45 min yesterday on working on it, still at the same place. Will look into it today again.
okay, tnx
@SparkyCracked Hello, sorry for the long offline. I was also looking for solutions from my side, but this issue seems like no one ever faced it lol
Yo wassup man. Yeah it's such a nieche issue. I honestly haven't even played around with this recently. I've been so busy it's crazy
I'll def look into it as soon as I have some time (my git profile is even looking horrendous lmao)
hehe.. no worries
if you want, you can take it as a challenge 😅 .. but I have to let you know that I have already given up on the project lol