Application commands are not updated in chat, but in "Manage Integration" are up-to-date.
Here is my code that updates commands:
When the bot starts to run, it loads all commands
After the bot is ready, it sets
client.application.commands
Here's an example of my command file:
Discord.js Version: 14.7.1
Nodejs Version: 22.2.016 Replies
- What's your exact discord.js
npm list discord.js
and node node -v
version?
- Not a discord.js issue? Check out #other-js-ts.
- Consider reading #how-to-get-help to improve your question!
- Explain what exactly your issue is.
- Post the full error stack trace, not just the top part!
- Show your code!
- Issue solved? Press the button!
- β
Marked as resolved by OP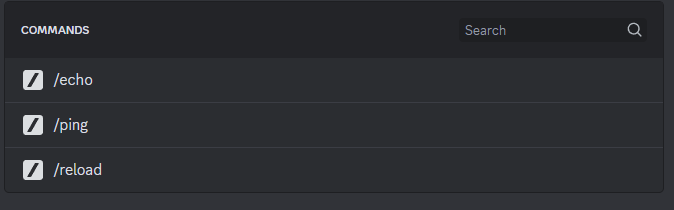
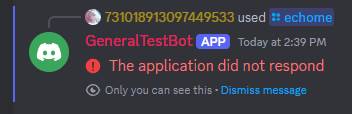
this is what I mean that commands are not "updated"
Did you try refreshing the app?
That error means itβs still a registered command tho
Yes, but when I check "Manage Integration", it does change.
"Yes" to that you tried refreshing?
ctrl + r
You are totally right.
So I should refresh the app every time I update....
For users, that will be a hassle, I suppose.
Any other way?
Or should I register commands, and it will change instantly?
I guess just refresh my discord
Thank for the response, I will note that.
hi guys do you know how to use discord.js
please say
you can try not to deploy on ready, only when you need it (like with an extra script, the guide has an example)
Isn't that registering commands?
I have no idea about the difference
you can check the guide https://discordjs.guide
discord.js Guide
Imagine a guide... that explores the many possibilities for your discord.js bot.
don't ask on random threads though, at least do it on #djs-help-v14
yes, in this context deploying/registering can mean the same thing, which is "sending" your command data to discord
oh, I see
What if I set
guild.commands
? will that be the same?that'd deploy/register your commands to only that guild
what I meant was, given commands don't change that regularly, you can try manually deploying them when you know it's needed, not on every start
Fair enough π
Thank you