Unable to get the FieldInfo's value
The following method should find the fields of type
T
in the class.
When having these strings
, it only returns a List
of 2 empty ones.
5 Replies
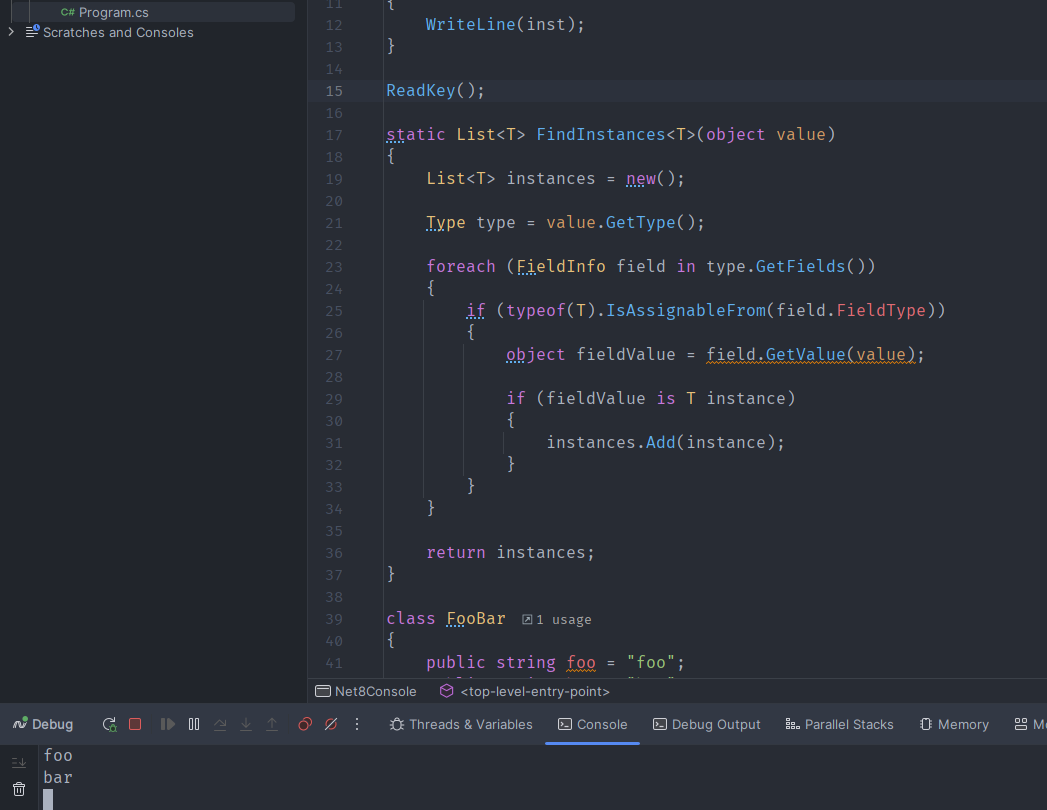
very much a "works on my machine" moment
Just run it in a console app and this works fine there. Seems like a Unity problem, since that's where I initially run it.
might have to use
type.GetFields(BindingFlags.Instance | BindingFlags.Public)
but I'm not 100%, I try to avoid reflection in Unity
but if you know for sure it's a Unity problem it might be a bit easier to find a solution with google at leastThe fields are found properly, and the value's type is checked properly too, it's just that
GetValue
returns the empty string
in Unity. I'll try to research this thing. Thank you a lot for your help!