How to display value or data in HTML with Javascript
I'm currently struggling with displaying values in HTML. I'm recreating the tip calculator as seen in the image linked below, i've marked the parts to show which labels/inputs im talking about. For now im not using the tip buttons since im mostly focussed on learning how to use the DOM and displaying/working with values that way. This also means the totalAmount is empty since its the same as the bill as there are no tips calculated.
1 The input label called "Bill"
2 The input label called "amountOfPeople"
3 the div was which has <div> 0, 00 </div> in it, which is called "totalPerPerson"
4 the div was which has <div> 0, 00 </div> in it, which is called "totalAmount"
Planning/Flowcharting
1 Put a value in the input label bill
2 obtain/store this value
3 Put a value in the input label amountOfPeople
4 obtain/store this value
5 calculate the formula by adding bill + amountOfPeople
6 store the result of the formula
7 display the formula in the totalPerPerson div, replacing the 0,00
8 display the formula in the totalAmount div, replacing the 0,00
Code
My question is, how can i make it work in the way that if i type the price and amount in both input fields, it automatically shows up in the div without having to use buttons to submit or statically display the value. it not about making the calculator work but more so about the syntax/ways to display or work with the data
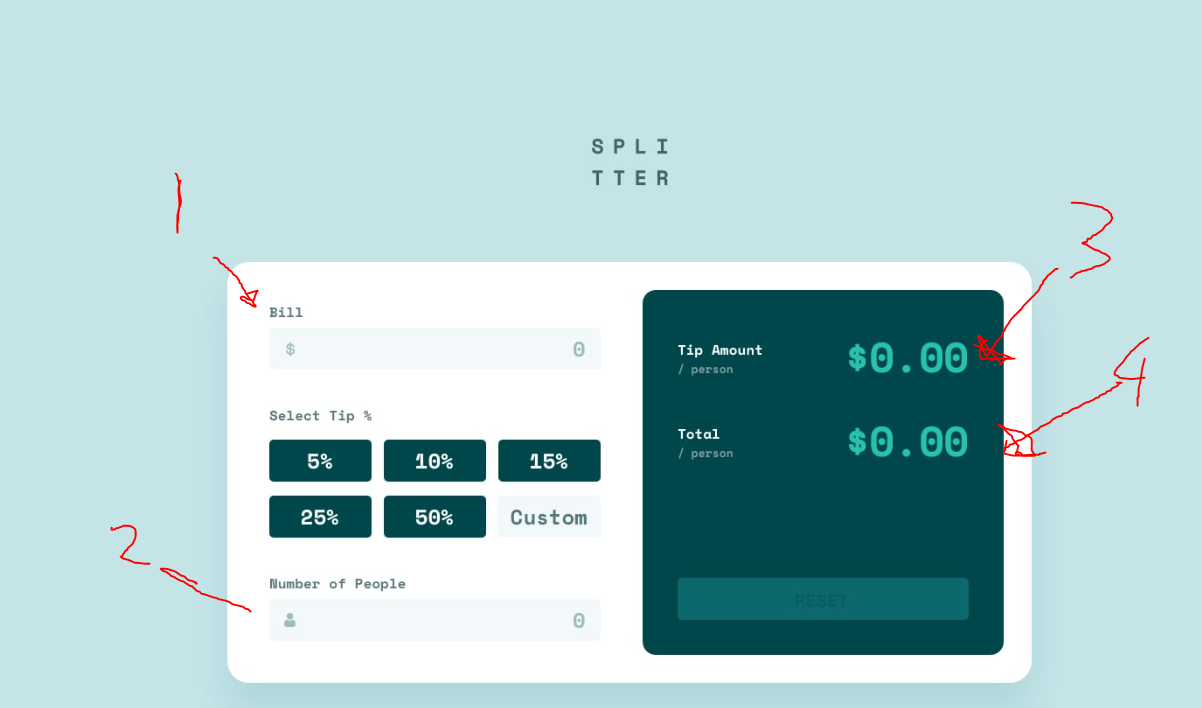
25 Replies
you can tie a
input
event to the inputs, then run the same code that would update the fields as when you press the button
(so that's elem.addEventListener('input', () => {});
)
(updated the messages, keypress is apparently deprecated)
it fires every time an input/select/textarea changes through direct user interactionso that would be like this? does that mean id have to do it twice, once for bill and one for amountOfPeople?
`
assuming
billPerPerson
is a global variable with the value you want, yeah. Though you can consolidate that:
or alternatively stick the listener on the parent, that should work too:
Looking at your code and wondered something, is my initial code good enough structure wise or am i missing out on things? aside from your solution
`
hmm, the main thing I'd notice now is that
let billPerPerson = bill / amountOfPeople;
will run only once, so it won't update unless you update it yourself
so like this
ya thats a hunch i had, i wasnt sure how to create formula's and use them multiple times
actually, gimme a sec, I'll rewrite some stuff with some comments
damm i was quite close, i think my flowchart was quite solid. its just that i lacked some parts like you showed
i googled it a lot and couldnt find this solution so its frustrating to be stuck
i honestly think i formulated it wrong and didnt get the right search results
it's a very common issue people have starting out, that they think that defining a variable will keep that variable updated as its components change 🙂
in reality, variables are (almost always) just postits that you write stuff on once and then can reuse places. Kinda like how the clipboard works on windows too, you copy a section of text and then change the original text, but the clipboard still has the old value
does putting it in a function automaticly keep updating it? since the code is the same right
well, the function runs multiple times. Every time the event is called, the code in the function executes
that includes updating the value of the variable
ah right, otherwise you'd have to repeat it multiple times, now you can just call the function
yeah
il implement it later today and report back if i run into any problems, thanks for the help
no worries, glad to help!
It works in some way? it starts at NaN and then when i type it shows the number i put in. it often doesnt calculate and just gets stuck on NaN. its quire hard to replicate but it works in the end
oh, that's a good point! Change this line:
to
thank you, if i'd want to implement the reset button. i can just do a onclick function that resets everything to 0 right?
`
if your values are in a form, you can just have a
<button type="reset">
in there. Or have an onclick that resets things manually, yeahWhats the difference between using () on updatetotal and to leaving them out as you did in the example code
`
vs
`
in the first example, you're passing a reference to the function to serve as the eventlistener, which will then be called each time the event fires
in the second example, you're calling the function and passing the return value of that function to serve as the event listener. updateTotal doesn't return anything callable though, so the event listener will fail
that makes sense, thanks!
it works and shows a 0.00 now, it often doesn't register/show the results while the labels are filled and other times it does. not sure why but it works so I'll put on solved
if you make a codepen, I can look at it though it might end up being tomorrow
its fine, the most important thing is that the DOM stuff works, il solve the small bugs later
kk 🙂