Problems with Form POST in Safari and IOS
I have this simple router, relying on a simple HTML form.
Using chrome, on my computer, i have no problems whatsoever
Using Safari on the computer, or safari or chrome from my iphone, or safari on the iPhone simulator, it doesn't work.
I get the message on my server:
TypeError: Request aborted, code: "ABORT_ERR"
I'm using Hono with Bun, like so:
any insights on why this may be happening?
Thanks29 Replies
What causes it to throw that error, just on load or submitting it?
The reason it happens on chrome on your iPhone is because it’s still the same engine as safari with just a different look
yeah, it's webkit, i know that 🙂
nothing throws, the error actually disappeared, i'm not sure why, i have been making changes
i have console.logs all the way down, and everything works as expected
but in the browser i get
So it’s working fine now?
the last console.log(3, 'token set')
is immediatly before the return c.redirect('/')
but it's also not the redirect
because i get the same result with
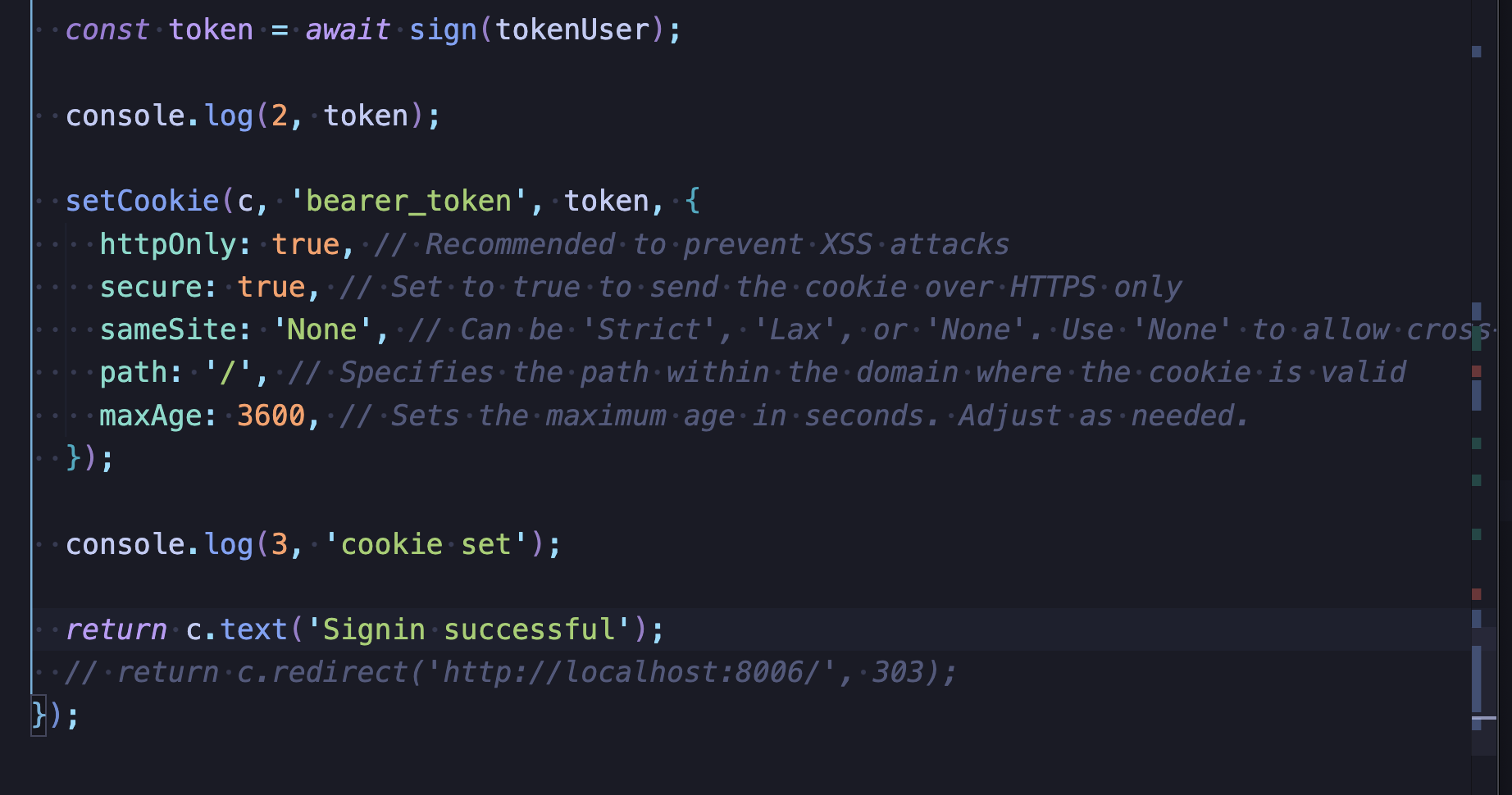
Do you have any middleware that fires before the response sends that could be messing it up
yes
hmm
Can you the commenting it out and see if it works
sure
and this works perfectly in chrome on the browser
also, from the iphone, it never even gets here
the middleware is only injected for the dashboard router
Well it server side so it would have to work in all browsers for that so it must be something else
yeah, but i can't figure it out
and it keeps on working on chrome, haha
oh, i found another problem
where the
TypeError: Request aborted code: "ABORT_ERR" disappeared it comes back if i do it stopeed erroring like that, because i had hardcoded email and password for a bit
TypeError: Request aborted code: "ABORT_ERR" disappeared it comes back if i do it stopeed erroring like that, because i had hardcoded email and password for a bit
Don’t destructure it, just set it as a variable and confirm your getting the email and password
Just for debugging I mean
that's what i did before
i commented it
and just had
const email = 'email';
const password = 'password'
so it did pass that obstacle, but in the end, the result was the same, nothing goes to the browser
But I’m saying confirm that you are getting a username and password in parseBody
oh i'll try that then
If not JSX might not set the form type automatically
*data type
<form class="mt-5 grid gap-2 lg:gap-8" method="POST">
and the headers come correctly
"application/x-www-form-urlencoded"
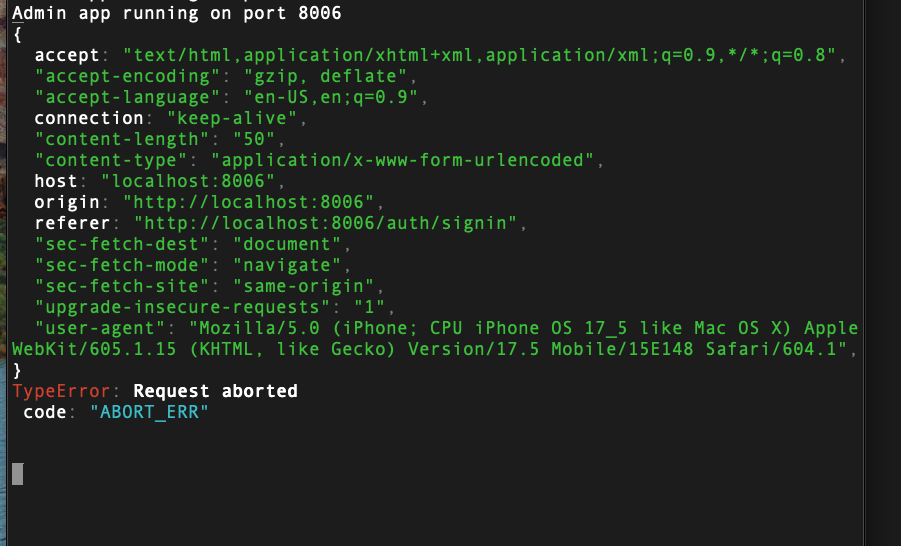
You are posting directly with the form correct, you’re not sending it with a fetch
posting directly
but i also tried with a fetch (using HTMX) and the result is the same
Would you mind sharing the whole route
i'm going to do something better
give me a sec
this is the simplest form, and it is working on safari also
with this, still working:
this is also working...
this, oddly, still works
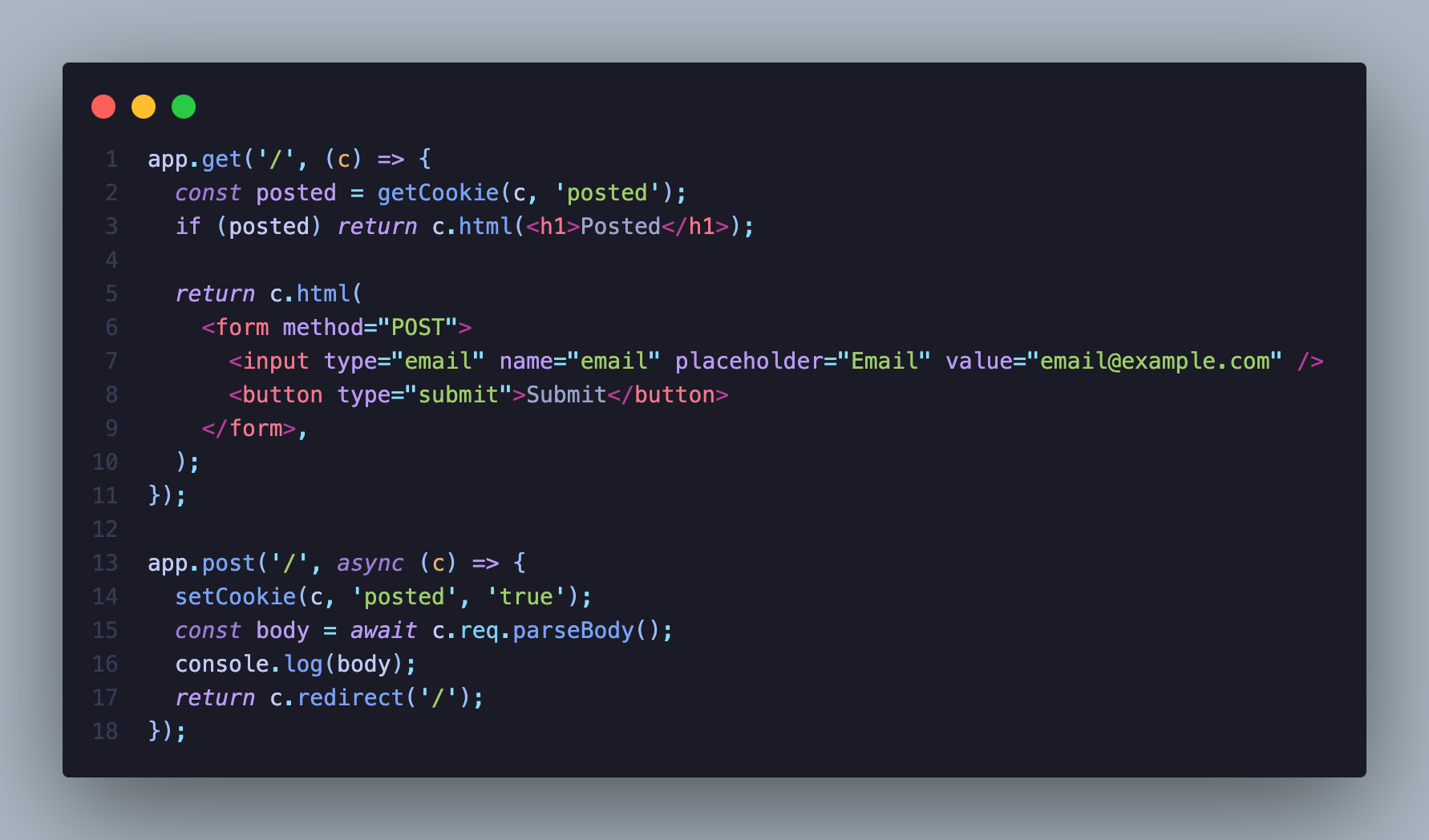
this still works, getting weirder
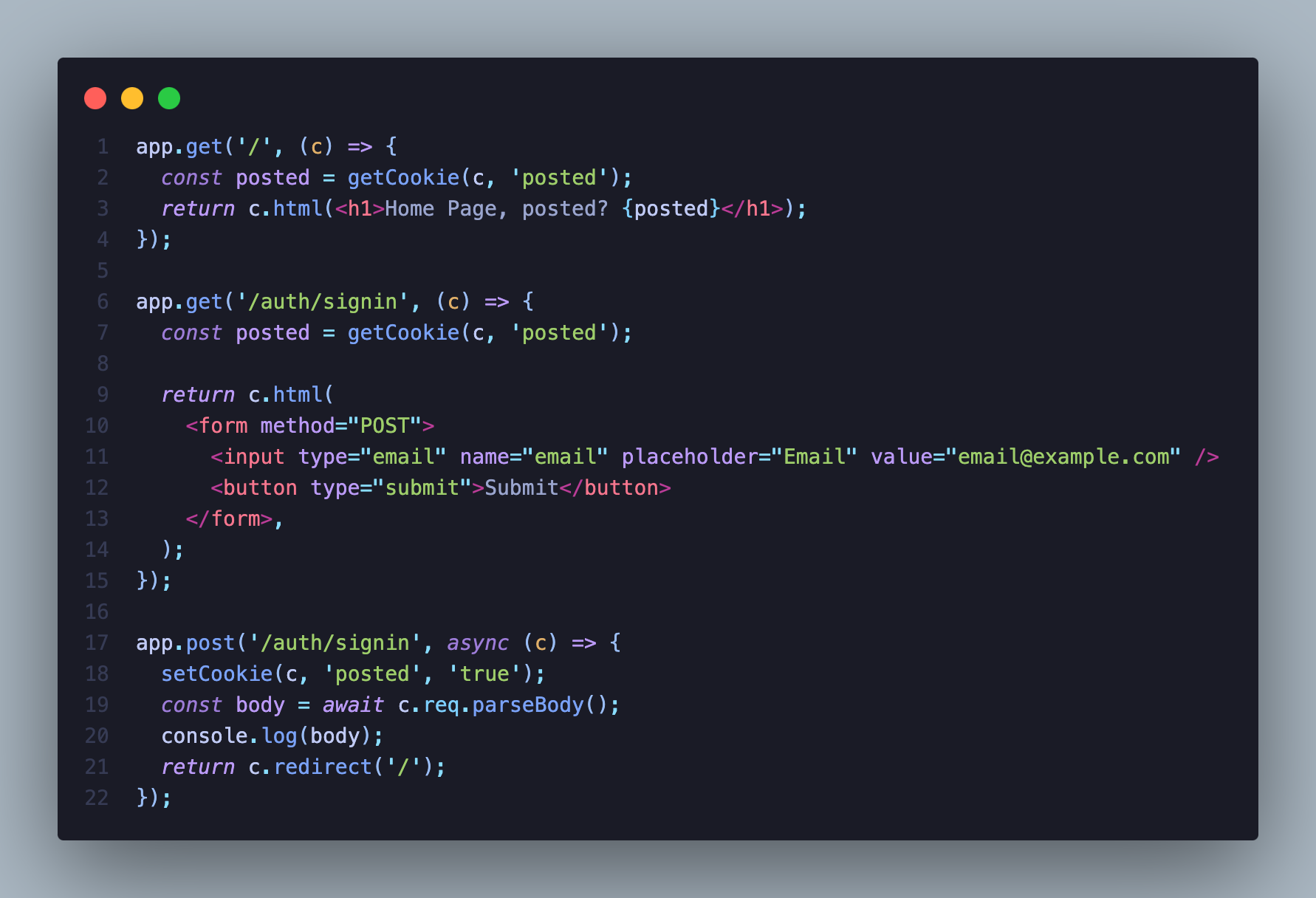
still working...
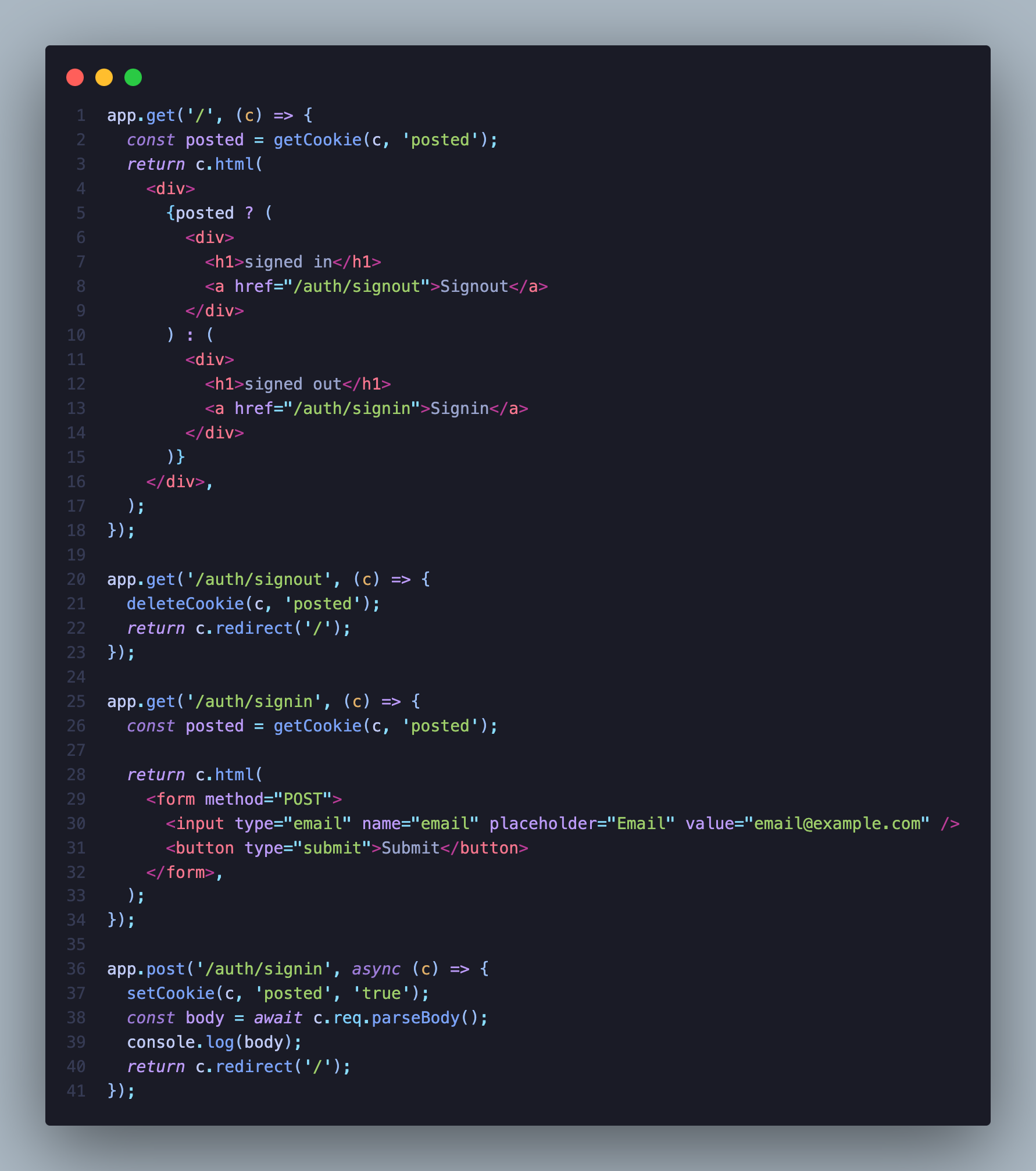
Hey I’ll come back to you I gotta step out for a bit. If we want we can hop on #voice and try and debug it
that would be great
than you so much
Ready when you are https://discord.gg/j6vbf8cZ