array doesn't want to go away
i want array values that are numbers to be deleted but it doesn't go away and it still shows as ""
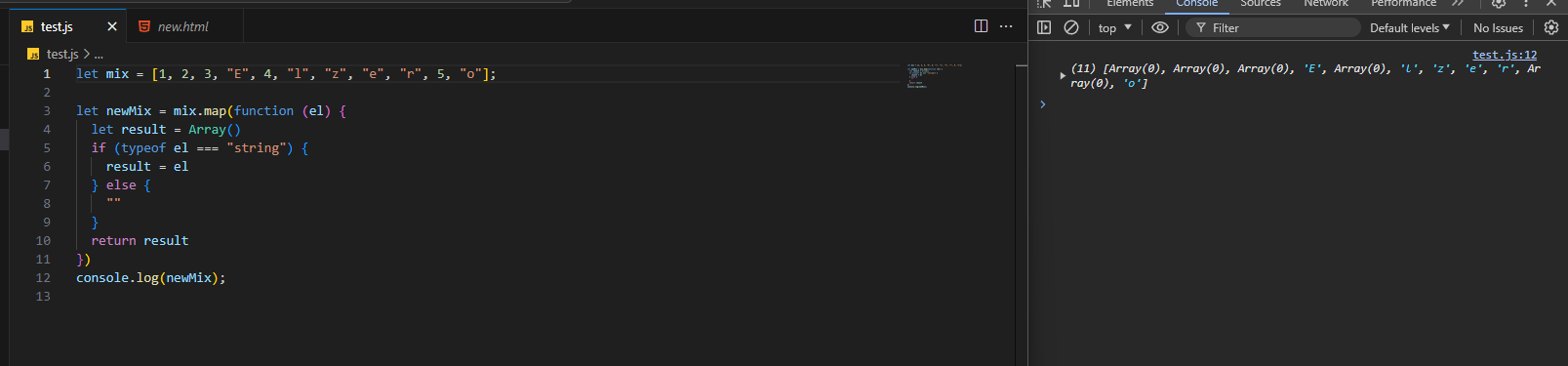
16 Replies
It's because you're using the
.map()
function, which takes in an array of X items and returns an array of the same number of items. Also, you're creating a new array in each loop for no reason—just return el
instead.
What you should be using is the .filter() function, which takes in an array of X items and returns an array of that many or less.
Which can be made even more succient by:
Lastly, please use code blocks (putting code between ``` ) so we can copy/paste your code. Going off of a screenshot isn't very helpful.thanks
but i have to use map because it's a homework
and i have to do it like this
.map()
won't change the array length, though. It'll always return an array of 11 items (as your initial array has that many items)so there's no way right
Not with
.map()
, nook what about .reduce()
.reduce()
? Yes
Both .map()
and .filter()
can be done using .reduce()
that's great
https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/reduce
The
reduce()
function's first parameter is a function that has two parameter: the accumulator
(which is the current value passed in from all prior loops) and the value
(which is the current value at the index being looped over). You then pass an empty array as the initialValue
so you have a new, empty, array to work with.
On each loop, you want to check if the value
is a string, if it is you add it to the accumulator
. If not, you do nothing. Be sure to return the accumulator
in the callback function so it can be used in the next loop.thank you very much
really appreciate it
Sure thing! Hope it helps
const newMix = mix.filter((x) => (typeof(x) == "string") ? true : false)
I think you mean: const newMix = mix.filter((x) => typeof(x) === 'string')
😸That works, too, yeah. I like the ternary operator, what can I say? 😉
In general,
statement ? true : false
is an antipattern since your ternary does nothing but complicate reading the logic.you guys are smart you need to 1v1 each other
Nah. We’ll tag-team a codebase instead :p