72 Replies
- What's your exact discord.js
npm list discord.js
and node node -v
version?
- Not a discord.js issue? Check out #other-js-ts.
- Consider reading #how-to-get-help to improve your question!
- Explain what exactly your issue is.
- Post the full error stack trace, not just the top part!
- Show your code!
- Issue solved? Press the button!Some of this looks like v13 code, not v14
@ʎǝɹquoɯ did i fi it
The
Intents
have changed
no, nothing here is fixed
Are you using ChatGPT or something?no
i put // Replace with your Discord server ID so i know
If you dont mind me asking, where are you getting the outdated code from?
bc im still learnign and it is notes
youtube
and myself
I recommend following our guide for the most up to date code and references https://discordjs.guide/#before-you-begin
discord.js Guide
Imagine a guide... that explores the many possibilities for your discord.js bot.
i try
and i might give up bc im trying to make all this with no help
so
That is quite a lot and our guide definitely doesnt cover all of that
ik
:monkaStop:
I would quit coding discord bot and do something more useful
just saying
it is for my server
if you are a beginner those might be little too hard for you
Unknown User•5mo ago
Message Not Public
Sign In & Join Server To View
Im not discouraging him lol
You literally told him to quit
shared my opinion
Please leave this thread if you have no intention of helping people use discord.js
👍🏻
This is not a place to share your opinion
Start with the basics and tackle the easy ones, build on those skills into more complicated items
some one said py is better
tf is the new intents
Sure, the video is fine, it shows you how to start the basics for your bot
i got all that i just need the intents
The intents didn't change between v13 and v14, they just changed what name they are under
@Jô 🌈🦄 you apere out of air
ty jo
do yall have a thing on this
client.once('ready', () => {
console.log('Bot is ready!');
client.user.setPresence({
activities: [{ name: 'd', type: 'PLAYING' }],
status: 'online',
});
ok
so i made the index to were it will auto make my command
is this something you made or some package/chatgpt thing? djs doesn't have anything that automatically makes commands
no like it regesters and it is from my old bot that my frinde fix like 3 things
Unknown User•5mo ago
Message Not Public
Sign In & Join Server To View
there is something similar in the guide, you can try to use the one you have or make a new one from the guide
oh i got it - i got the
now i got to make a embed maker
witch im go do
i found it
yes no
i think i did good
just got to waait for my bot to chatch
option types aren't uppercase strings anymore, they are either enums or numbers
what
wait do i have to export it
yes, it is a type you have to import
otherwise you can use the corresponding numbers
no fo it to work do i have to export it
Please be sure that the code you're using is for the correct version. You tagged this post for v14, however
MessageEmbed
is found in v13what guide are you using ^^
you're on discord.js v14 but the code you've been providing has been v13
oh dam
im on the one you give im using some stuff from my old bot from v13
That is why I tagged how to create an embed, https://discord.com/channels/222078108977594368/1255010013815242793/1255023881052356681
i can find it
as of what it is to do what im doing
could you rephrase that maybe? I have no idea what youre asking here
ii cant find how the embed thing im making is made
what are you looking for that is different from what the guide provides?
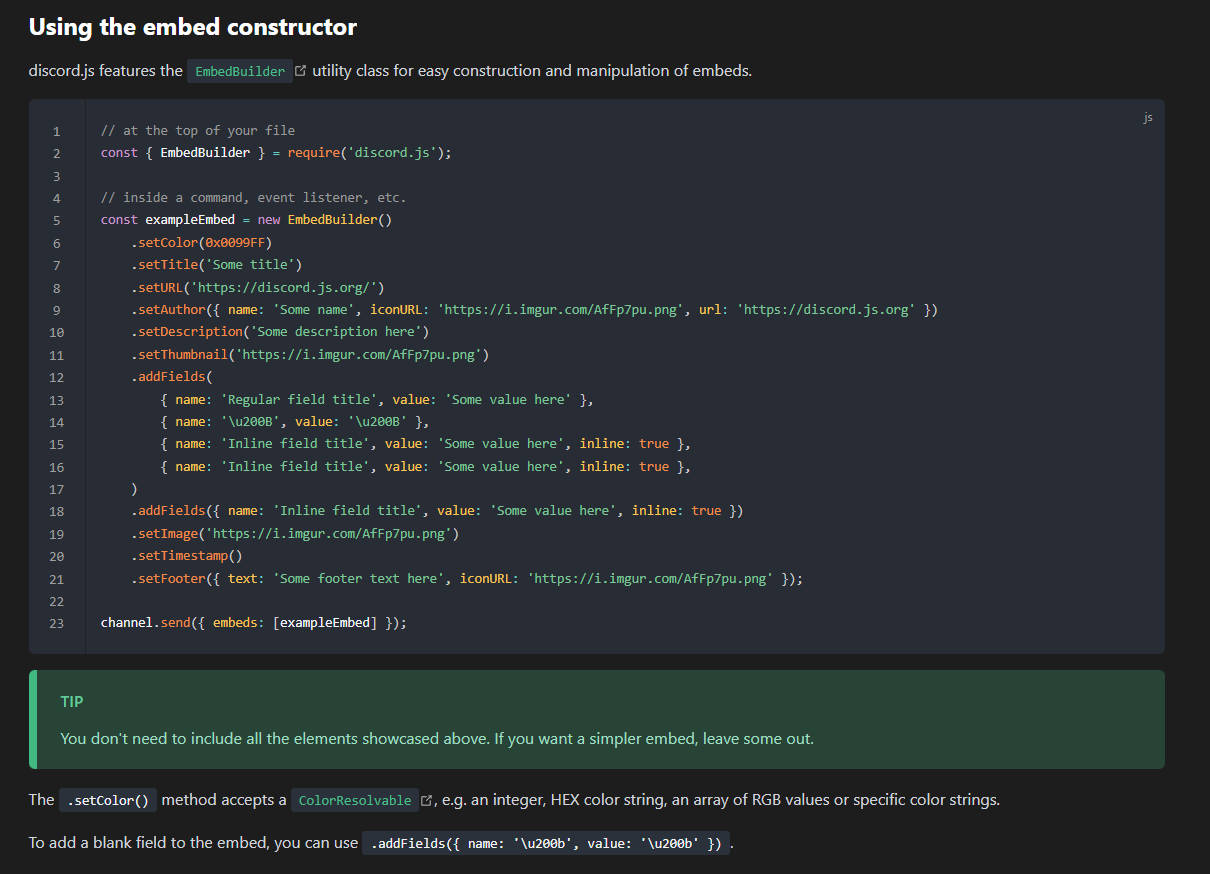
this
anddddd what does it not have?
sorry but im new to v14 and stuff
I'm confused on what you say isn't in the guide that is in your code
string
For options or the embed?
both
we'll start with options
wait did i do it right
or
bc im dumb
how are you parsing your slash commands data when updating your slash commands?
huh
when you upload slash commands to discord, what code are you using to do that
and where is your
deploy-commands.js
?
oh and
again, those types in
options
have to be either an enum or numberwhat
here if you wanna look at it all rq
https://prod.liveshare.vsengsaas.visualstudio.com/join?FCF2AFD2FB20FE0E4D9766D0B2D183681138
something like that