Problem while "dotnet ef migrations add Init"
When I'm trying to add data base migration i get error "The seed entity for entity type 'Contract' cannot be added because a non-zero value is required for property 'ContractId'. Consider providing a negative value to avoid collisions with non-seed data."
table (look screenshot)
insert :
table has autoincrementation:
table code:

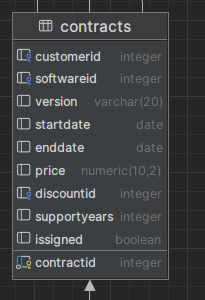
32 Replies
You don't need this code :
EfCore knows that
ContractId
is the PK of your table so you don't need to specify it manually. Remove it , delete the previous migrations and then add a fresh migrationstill same problem
my migration doesn't even create so i cant delete anything
can you show the entire db context class and the full error you get while migration ?
sure
wait let me
add it to github
sure
GitHub
GitHub - Juks0/CompanyAPI at master
Contribute to Juks0/CompanyAPI development by creating an account on GitHub.
this code is also redundant :
deleted it, still the same
why manually passing the
CustomerId
?oh there are 2 customer insterts
my bad
clean up your DB Context class...and see if it works. Overall code seems fine to me other than manually specifying the PK etc
i made Insert for companyCustomer, i get diffrent error withnot specyfying pk
exactly
i need to specify primary ket at the top of class in Models? and every goreign key like here?
does the migration work when you manually add the PK while seeding the data ?
wym manually
no you don't need that
so how should i do it then?
I mean when you include the PK in the object while seeding
let me check
sure, include the PK in the objects and then see
yh it did work
so if i dont provide anything just leave this blind it will know it need to autoincrement ID?
no it wont
when i didnt provide pk manually i get the same error
The seed entity for entity type 'Customer' cannot be added because a non-zero value is required for property 'CustomerId'. Consider providing a negative value to avoid collisions with non-seed data.
You need to provide the PK while seeding but not in the application while inserting
You will see when you Will add a new record using EF Core without providing PK , it'll work
seeding means where?
In Db Context
yeah i know that, but could you provide me an example, i have no idea where to specify a PK
well listen.
let's say your model is called
Book
, it'll look like this :
So the Id
here is the PK and EFCore knows it . So while seeding the data you need to provide this PK which is Id
in this case but in the application while adding something to the schema/database you don't need to specify this. For example you'd just do :
https://learn.microsoft.com/en-us/ef/core/modeling/keysExactly, so i provided class:
my insert looks like this:
do i need to provide this part of code? I dont think thats the reason
In link you send there is a Example where we specify PK in model class
should'nt i do it like that?
and btw thanks for your help you're legend
Oh no! As I said while seeding you do need to provide the PK i.e
yh, but how to do it when i dont wan't to provide it
this part is for the EfCore queries not for the seeding data and is not relevant in the db context class. You'll use EfCore throughout your application .
You'd need to provide it while seeding
so to make it clear as i understand it
this is only way to do it because i always need to provide customer ID while seeding, you can't use auto incrementation here?
yes while
seeding
you'd need to provide itthanks, ill try to make it now
got it
thanks a lot my man