Modal Submit not Working
Can someone help me, I can't submit a Modal
I can open it, but it won't even get to the
submit
function for some reason
packages :
:djs: @discordjs/builders: 1.8.2
:djs: discord.js: 14.15.3
command :
6 Replies
- What's your exact discord.js
npm list discord.js
and node node -v
version?
- Not a discord.js issue? Check out #other-js-ts.
- Consider reading #how-to-get-help to improve your question!
- Explain what exactly your issue is.
- Post the full error stack trace, not just the top part!
- Show your code!
- Issue solved? Press the button!
- ✅
Marked as resolved by OPevent:
Also it prints an 'undefined' on Modal Submit
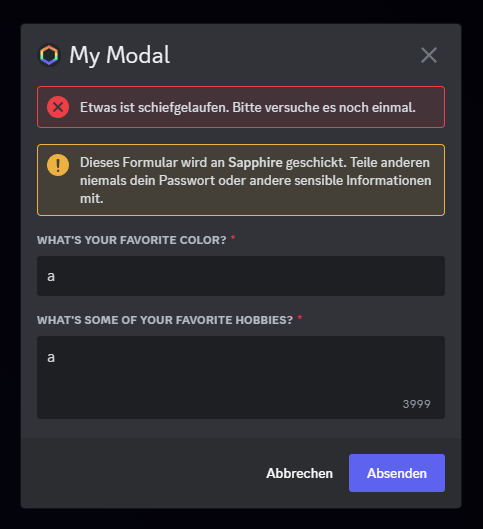

Your event is going to immediately ignore anything thats not a command
!client.commands.has(interaction.commandName)
You should be doing somrthing like
or, handle the modal in the commandi see now its working thanks!