"Duplicate property" Entity Framework 8 Error
I've got this error popping up on OnModelCreating method:
this is how I'm setting up AlternativeShift there:
8 Replies
this is my AlternativeShift class:
and this is my AlternativeShift class:
is probably confusing it, you don't need that attribute
Also I personally would name that column RotationId anyways.
well, it's named as Rotation in the DB, which is why I'm adding that.
The deal is that it was a legacy website which I started to update to .net 8
But eitherway even removing the Column annotation the same error pops up!
if you have an existing database, is there a reason you aren't using scaffolding / reverse-engineering?
I did, thats how that class AlternativeShift was created initially.
I simply added the column data annotations. Used to work before!
Ahh I see
looking further into the modelBuilder, I can see there's two RotationIds, one being a shadow property. not sure why this would get created as I already have RotationId field
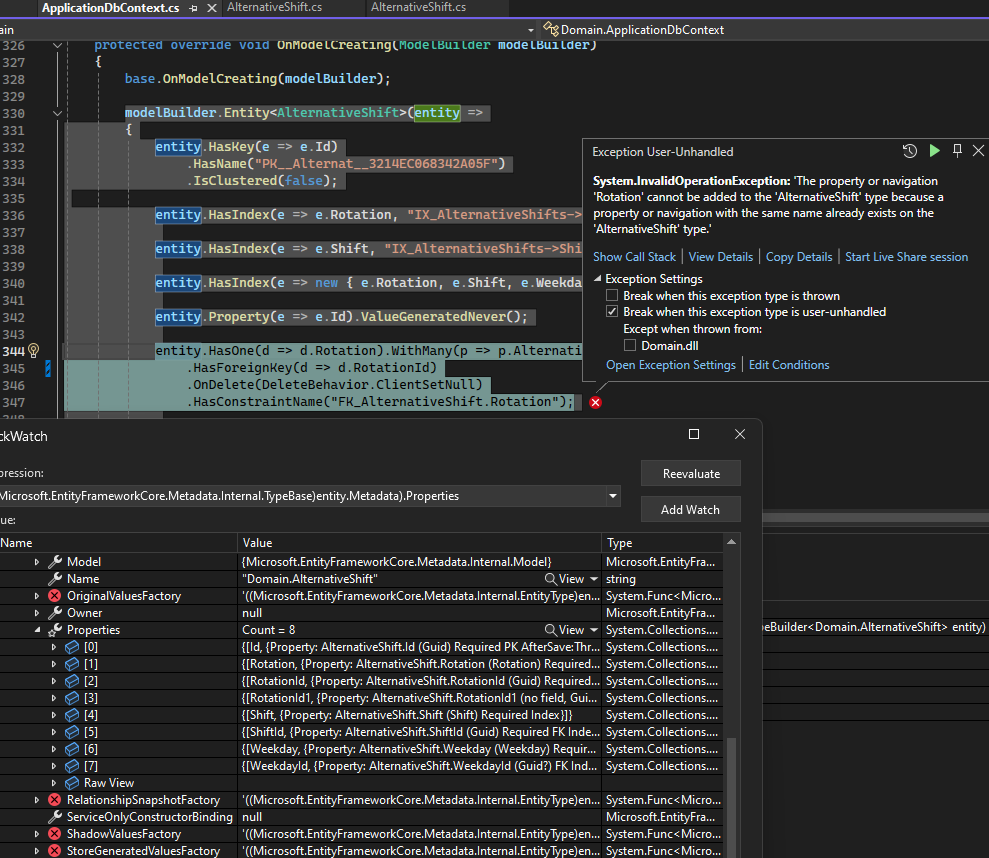