Singleton - has no index
Hi, i'm working with twill and i need to create a singleton, but i navigate to the link i get this "Blog has no index" ,i have also done the seeded command, this is my code
<?php
namespace App\Http\Controllers\Twill;
use A17\Twill\Http\Controllers\Admin\SingletonModuleController as BaseModuleController;
class BlogController extends BaseModuleController
{
protected $moduleName = 'blogs';
protected $indexOptions = [
'permalink' => false,
];
}
15 Replies
Sounds like you are defining the routes with
::module('blogs')
instead of ::singleton('blog')
, or using forModule
instead of forSingleton
in your Twill navigation.this is how i have done it
Route::name('blogs.posts')->get('blogs/posts');
Route::group(['prefix' => 'blogs'], function () {
TwillRoutes::singleton('blog');
TwillRoutes::module('posts');
TwillRoutes::module('categories');
});
TwillNavigation::addLink(
NavigationLink::make()
->title('Blogs')
->forRoute('twill.blogs.posts.index')
->doNotAddSelfAsFirstChild()
->setChildren([
NavigationLink::make()->forSingleton('blog')->title('Blog'),
NavigationLink::make()->forModule('posts')->title('Posts'),
NavigationLink::make()->forModule('categories')->title('Categories'),
]),
);
What's the url where you are getting the error? /blogs/blog?
yes
Did you try putting the singleton outside of the blogs prefix?
i tried it now and i get this Attempt to read property "active" on string
that's likely unrelated, and I'll need more info about the error to help you, please try to be more explicit. Did you generate this singleton using the CLI?
yes that's how i generated , this is a image of the error, it shows when i'm at admin/blog
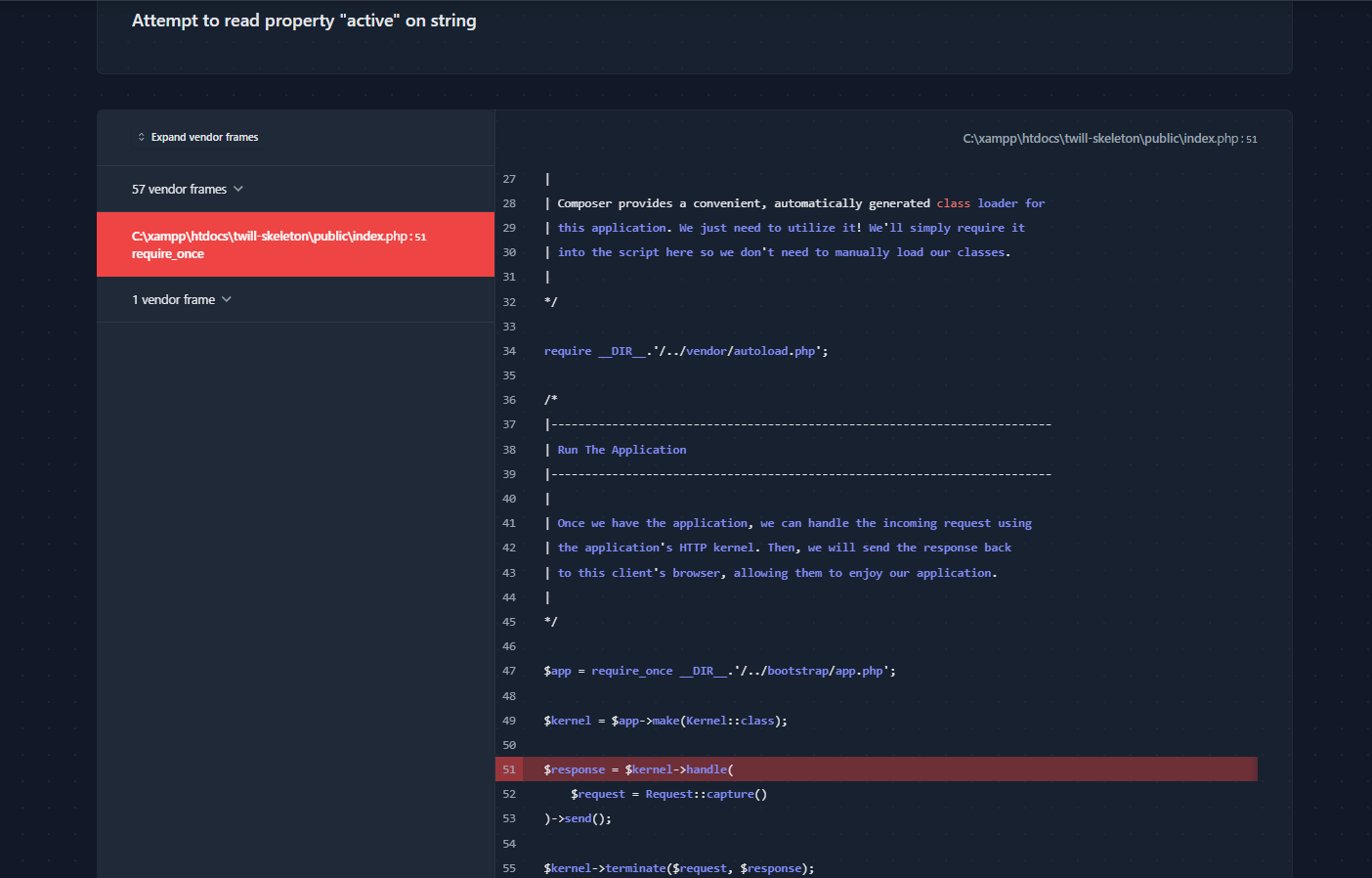
Is the singleton using HasTranslations?
yes
Ok, please show me your model and repository classes for that singleton
this is the model
<?php
namespace App\Models;
use A17\Twill\Models\Behaviors\HasBlocks;
use A17\Twill\Models\Behaviors\HasTranslation;
use A17\Twill\Models\Behaviors\HasMedias;
use A17\Twill\Models\Behaviors\HasRevisions;
use A17\Twill\Models\Model;
use CwsDigital\TwillMetadata\Models\Behaviours\HasMetadata;
class Blog extends Model
{
use HasBlocks, HasTranslation, HasMedias, HasRevisions, HasMetadata;
protected $fillable = [
'published',
'title',
'description',
];
public $translatedAttributes = [
'title',
'description',
'active',
];
public $metadataFallbacks = [
'title' => 'title',
'description' => 'description',
];
public $slugs = [
'en' => 'news',
'it' => 'news',
];
public $mediasParams = [
'cover' => [
'default' => [
[
'name' => 'default',
'ratio' => 16 / 9,
],
],
'mobile' => [
[
'name' => 'mobile',
'ratio' => 1,
],
],
'flexible' => [
[
'name' => 'free',
'ratio' => 0,
],
[
'name' => 'landscape',
'ratio' => 16 / 9,
],
[
'name' => 'portrait',
'ratio' => 3 / 5,
],
],
],
];
public function getSlugs() {
return $this->slugs;
}
}
this is the repositery
<?php
namespace App\Repositories;
use A17\Twill\Repositories\Behaviors\HandleBlocks;
use A17\Twill\Repositories\Behaviors\HandleTranslations;
use A17\Twill\Repositories\Behaviors\HandleSlugs;
use A17\Twill\Repositories\Behaviors\HandleMedias;
use A17\Twill\Repositories\Behaviors\HandleRevisions;
use A17\Twill\Repositories\ModuleRepository;
use App\Models\Blog;
use CwsDigital\TwillMetadata\Repositories\Behaviours\HandleMetadata;
class BlogRepository extends ModuleRepository
{
use HandleBlocks, HandleTranslations, HandleSlugs, HandleMedias, HandleRevisions, HandleMetadata;
public function __construct(Blog $model)
{
$this->model = $model;
}
}
Remove HandleSlugs from the repository
since you are not using HasSlug in the model
yeah, i did that, it works now.Thank you very much!