full stack Nextjs on pages with hono | Sometimes Work, Sometimes Not
I am getting some weird error, once there is some error in my useEffect hook, i get 500 error back in reponse. Then if i again reload the page the whole website with all routes whichever i visit shows 500 error page.
It sometimes work,sometimes not
this is how my useEffect looks like:
Here is my /api file
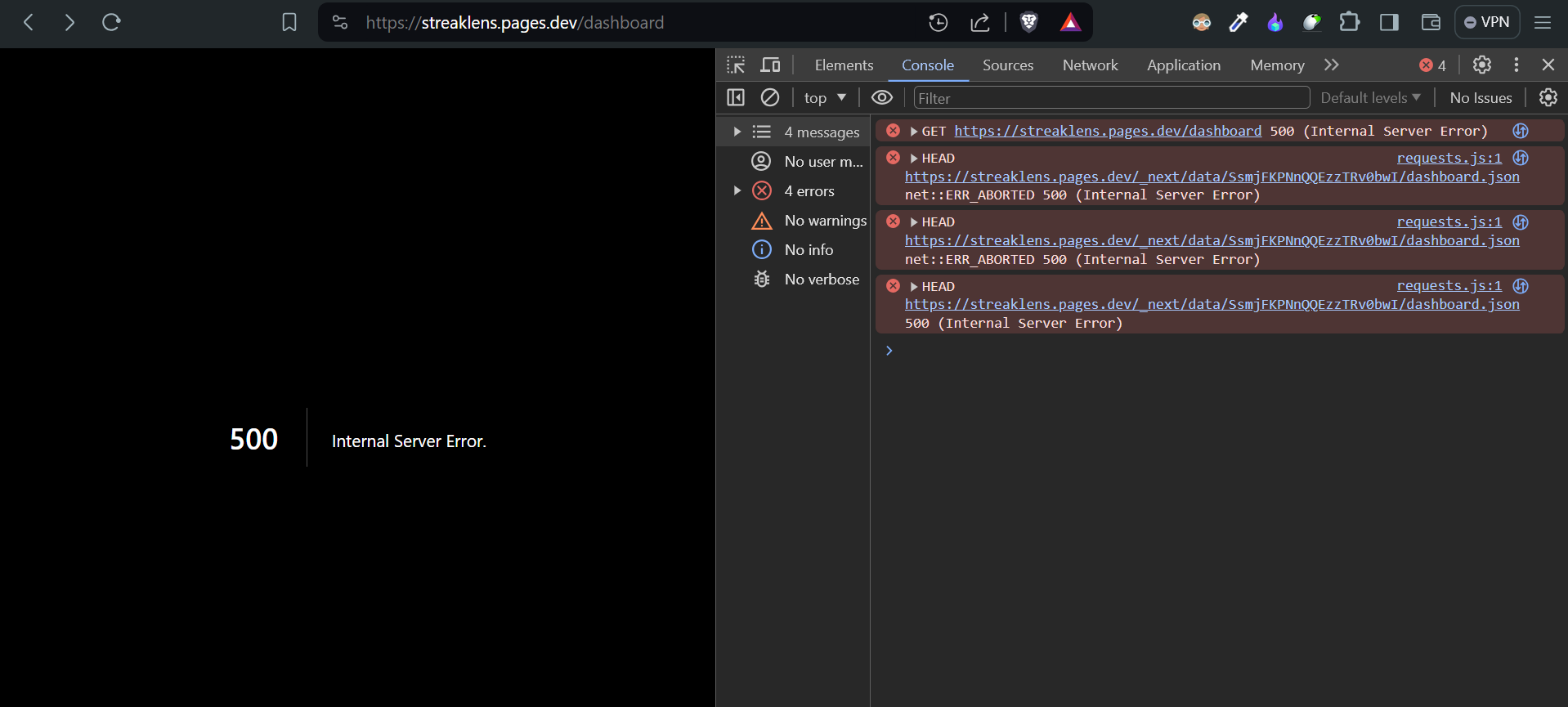
8 Replies
Are you sure this is related to the /api/news/top request? UseEffect is a client side hook, so should be executed on the server and shouldn't be responsible for this internal server error
Are you using Next on pages?
yes I am using next on pages.
but ig problem is with KV, i also tried using KV api, its working in localhost but not in production.
Also when some internal server error happens ,and that default black 500 page comes,the whole website becomes unavailable for that browser,, every route then starts showing that black page
Might be something related to your configuration of your env variables in production? If it's working locally, the KV seems to be accessible, so are you sure you can get the right ctx from your API handler?
I tested this also but nothing worked
i think the api routes dont work sometimes in next on pages
Hmm yep, seems Next.js related, any chance you can provide a repro? I'm not as familiar with Next-on-pages intricacies, might be more helpful for @Greg Brimble | Cloudflare and/or to submit to https://github.com/cloudflare/next-on-pages
GitHub
GitHub - cloudflare/next-on-pages: CLI to build and develop Next.js...
CLI to build and develop Next.js apps for Cloudflare Pages - cloudflare/next-on-pages
Repo link: https://github.com/Mrinank-Bhowmick/streaklens
Live URL : https://streaklens.mrinank.me (frontend: vercel, backend: worker)
Actually sometimes its working, sometimes its not. Thats the main problem, after too much trial and error i guess
sometimes internal api routes calling dont work like this:
But fetching outside URL always works:
and one more wired thing I observed that if in any case the user gets 500 internal server error , the whole website with all the routes starts showing 500 internal server error
@Better James
Repo link: https://github.com/Mrinank-Bhowmick/streaklens
Live URL : https://streaklens.mrinank.me/ (frontend: vercel, backend: worker)
GitHub
GitHub - Mrinank-Bhowmick/streaklens: AI Platform for Content Creators
AI Platform for Content Creators. Contribute to Mrinank-Bhowmick/streaklens development by creating an account on GitHub.
StreakLens
AI Platform for Content Creators
@Mrinank Hey i had a similar problem and fixed it by downgrading next to version 14.1.3.
Let me know if that fixed it for you