[Reading files from sftp server] is what i am trying to do possible?
hello, in the following code:
Basically, I clone the repo to my stfp server and thats all good so the next step is to analyze the solution. However, since all of the files are remote and not local, I cant use
workspace.OpenSolutionAsync
, because that method takes a local path. I don't have any way to say hey look at my remote server and then create a new workspace and solution there like how I am doing above. I was trying to see if there was a way I could run the code via ssh command, but i dont think thats possible.
Also, there is no point in trying to download any files because, if I am going to do that I might as well as just cloned it locally from the start and not use sftp. But I cant do local cause its a web app. Any advice?29 Replies
you could try mounting the path with nfs/smb and give that path to the OpenSolution
(you can enable nfs in windows from the modules in the application cpl)
you could try:
cSSH.Connect();
var response = cSSH.RunCommand("c:\test.exe");
dont really know if this stil works.
It's possible, but you need to understand that it's an OS level thing. MSBuildWorkspace is going to be using System.IO.File to read and write files. And that goes directly to the OS. So you need the remote drive/directory mounted to the OS.
Nothing you do in C# to connect to SFTP is going to be relevent there.
Then I dont have any idea except a program there to do the things you want.
ok, i mounted the drive, so my code works now. I can analyze my files, and its not storing anything locally.
But my question is, this will be a web app, so other people can use the app. does that mean that, my PC always has to be running with the mounted sftp drive for my app to work? that doesnt sound right? I mean, cause if my pc is off or I unmount my drive, then that means that the solution will point to a directory that doesnt exist?
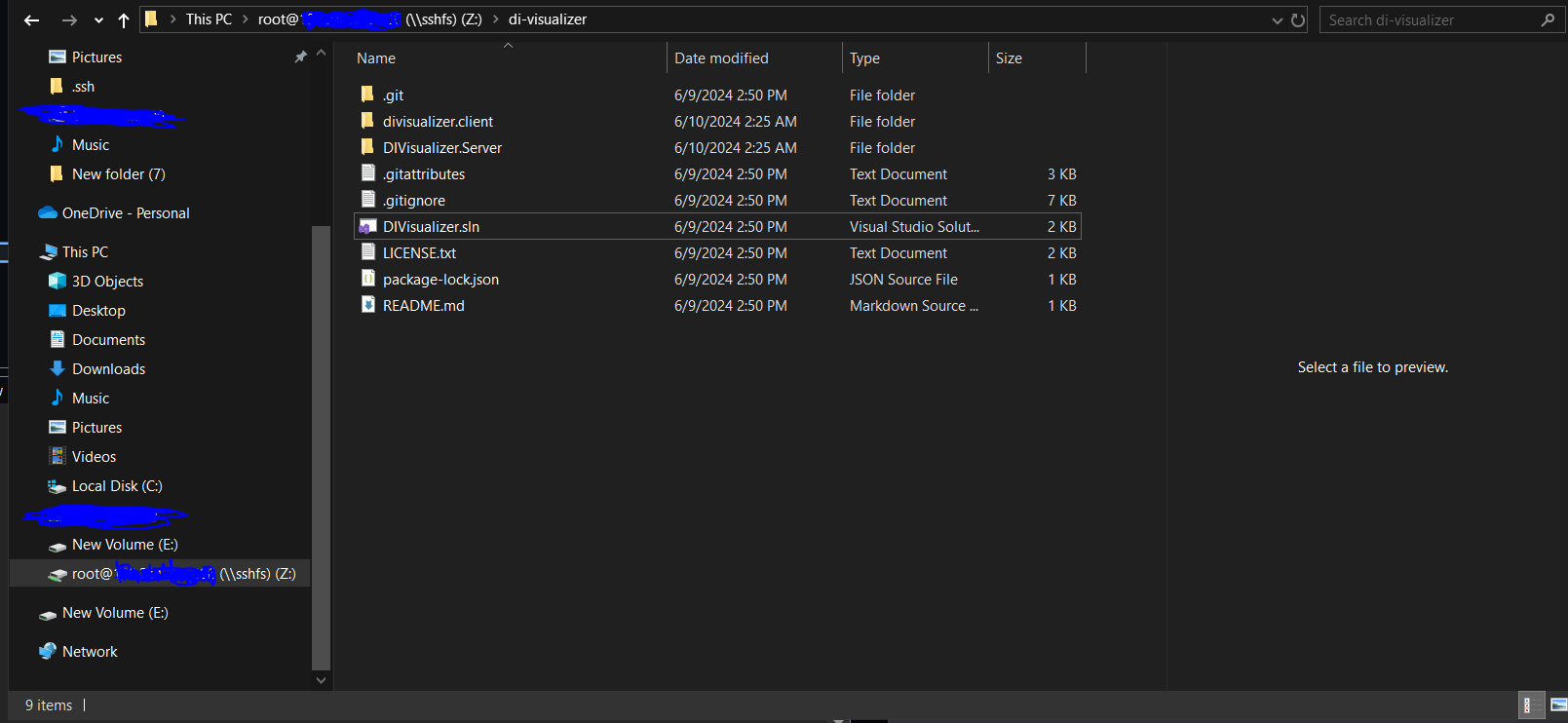
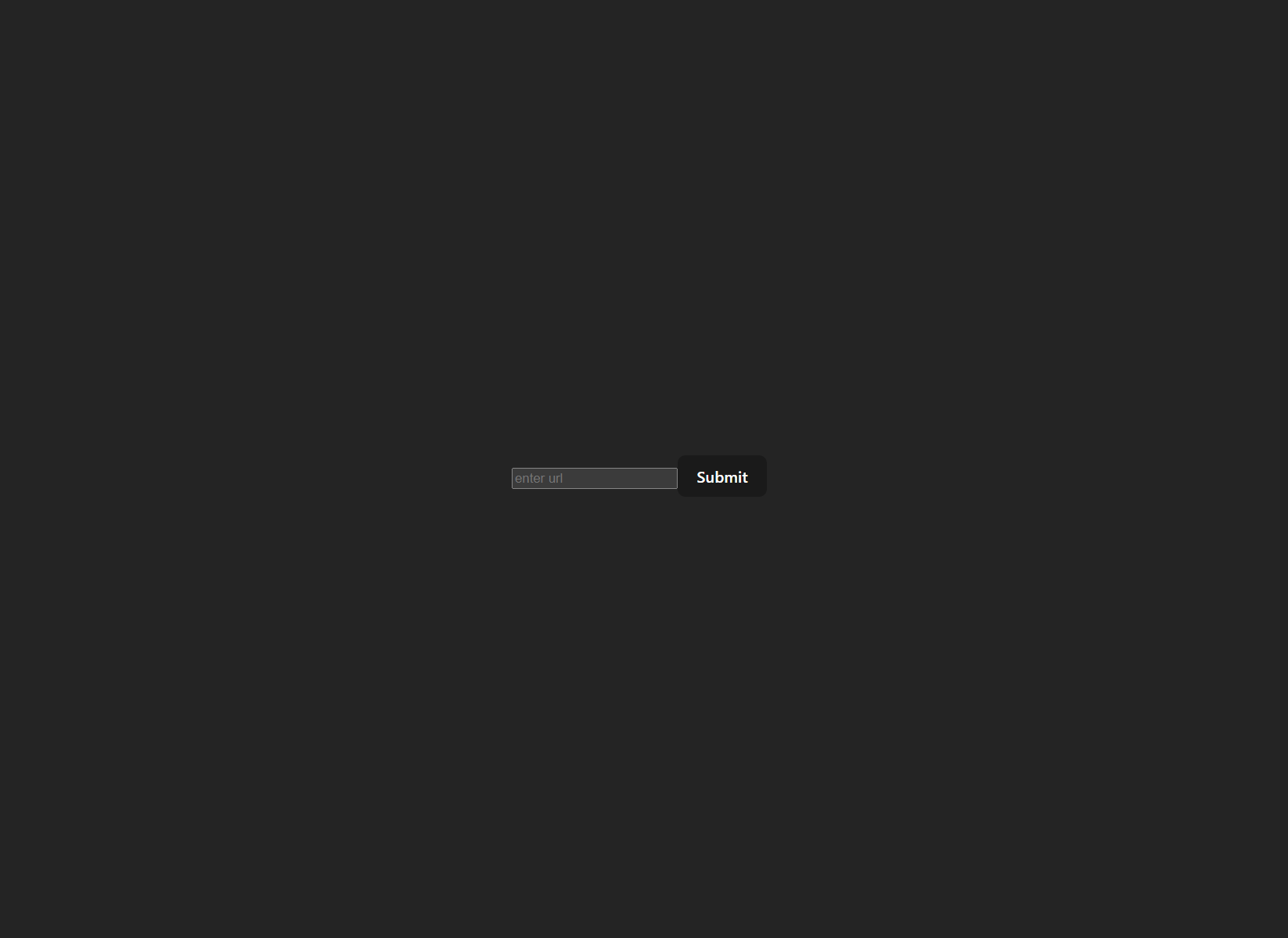
Yes. Of course.
Why in the world are you trying to do this anyways?
i dont know just for practice, i didnt think i want to make another crud app
I mean SFTP. Why?
Real people deploy built apps to web servers for hosting.
i mean i bought a vps droplet with digital ocean, so i would have a virtual computer to clone repos to, and then that is always running so thats good. but now I arrived at the situation where, I need to access the solution in my code, and i cant do that without mounting the drive it seems
my plan was to just always have that digital ocean server running, and the purpose of sftp was to read/write/edit files from my code to the digitial ocean vps, but it seems to be limited in that regard
Teh solution should be on your computer.
Not on some remote machine.
YOu build the app, and deply the RESULT to the server.
That is after all what the point of building in the first place is. Source is turned into binaries. Binaries get deployed.
"You can clone the repository to your VPS and do the analysis server-side then present the results to the user" <-- this is what I was trying to do basically. Is this unreasonable?
this is why I used sftp, so i can communicate with the vps
Running a program on your computer that analyzes a remote program, is running the analysis locally.
and do the analysis server-side
But I also have no idea what software you're using. Doesn't look like you ever mentioned it.
im using SSH.NET https://github.com/sshnet/SSH.NET
GitHub
GitHub - sshnet/SSH.NET: SSH.NET is a Secure Shell (SSH) library fo...
SSH.NET is a Secure Shell (SSH) library for .NET, optimized for parallelism. - sshnet/SSH.NET
I mean for analysis. I do not know what you are quoting.
Kouhai
You can clone the repository to your VPS and do the analysis server-side then present the results to the user
Quoted by
<@326800686845263872> from #how difficult would this be to do? (click here)
React with ❌ to remove this embed.
I am aware that I would have to host my backend somewhere, and then that backend would handle the processing. cause right now i am doing it locally
but then my server would be trying to read from my local mount which doesnt make sense i think?
I see. So you are not doing the analysis server side as suggested by the quote.
The person you quoted said to clone the code on the server, and then run the analysis on the server. So there would be no mounting involved at all.
Both parts would take place on the same machine.
yeah
So again, why SFTP? Heh.
but the problem i was having with that is that
OpenSolutionAsync()
looks for local files only. so it breaks the code basically. and I dont think there are any ssh commands I could use to mimmick what it doesRIght. Which would work. Because when running ON THE SERVER, local means ON THE SERVER.
But that's not what you're doing. You're running the code ON YOUR DESKTOP.
but if I deploy my backend somewhere, and i say to find a path, my backend has no knowledge of any paths in my VPS.
The backend would be on the VPS presumably.
the digital ocean vps is basically another computer somewhere else, so I would have to deploy my backend on that same computer then basically?
Uh huh
ok, so basically, my vps needs to have my server on it that is running locally to that vps, and then its code will have access to all the paths that i want to access that are in the vps
Right, so, exactly what the other poster you were quoting said. You write a program that clones the repo, and then analyses it. Wherever you deploy that (VPS or not) it will do that.
ok
that make sense so, i think my next step is to figure out how to get my backend running on my vps. i can move the files there easily but to actually run it, maybe i can do that from the cmd line. I think you can run solutions that way. but i will look into it. and then after that I should not have issues with the path
and then the backend would have to be listening for requests too, so it cant be a localhost url i think.