Help designing classes for a game
Have to design a menu class for a game assignments I'm working in Windows form using my teachers template. I posted somthing similar today earlier but found out my code wasn't any good. I can share the actual file on GitHub if anyone is interested in helping me out or guiding me through it
https://github.com/SonicArray3/Breakout
23 Replies
Breakout begins with eight rows of bricks, with two rows each of a different color. The color order
from the bottom up is yellow, green, orange and red. Using a single ball, the player must knock down
as many bricks as possible by using the walls and/or the paddle below to hit the ball against the bricks
and eliminate them. If the player's paddle misses the ball's rebound, they will lose a turn. The player
has three turns to try to clear two screens of bricks. Yellow bricks earn one point each, green bricks
earn three points, orange bricks earn five points and the top-level red bricks score seven points each.
The paddle shrinks to one-half its size after the ball has broken through the red row and hit the upper
wall. Ball speed increases at specific intervals: after four hits, after twelve hits, and after making
contact with the orange and red rows. You are to use these rules to implement Level 1 of your game.
Additional levels can contain other colour bricks with other scoring values. You must include bricks
that have special features such as:
• Bricks that never disappear
• Bricks that require multiple hits in order to disappear (maybe change colour)
• Bricks that change the ball speed or size (speed up or slow down)
• Bricks that drop items when hit that when caught by a paddle, a paddle effect is added (such
as longer or shorter paddle, ability to fire bullets at bricks)
game description
I just want it to look like this lol
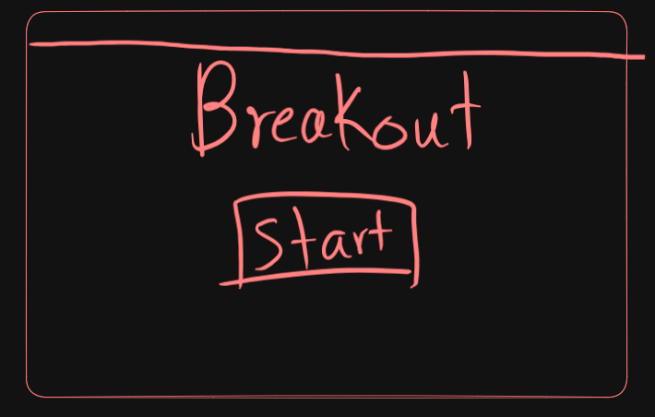
With a background n stuff, maybe a slight tutorial
The repo is private
Dang
I added you to it
Can't you make it public?
First thing -- learn about gitignore and how to commit to git
How to use git locally
Second thing -- use modern project files
They support winforms
Sorry if this is a silly question but what does it mean to commit to git? This is my last assignment of the school year and my teacher wants us to just use the template he has given
Thanks for this suggestion - alot of the time I ask this in the server people tell me similarly
But since my teacher usually provides the templates I can’t really adjust it
Any tips on the actual code I can implement
Read on git. Not github. Git specifically. Git basics. Then you'll understand.
This is one of the first things you should learn and it should only take you a couple hours.
https://github.com/SonicArray3/Breakout/blob/9d912288327dc6109cb9ca8a50375dd0c3673f11/Breakout%20-%20Student-20240603T140721Z-001/Breakout%20-%20Student/Bricks.cs#L10
Why is this empty?
Oh
So you haven't done anything yet
I see
What do you know about games? Have you made any games before?
Have you heard of an "event loop"?
Have you learned how to simulate physics in your class?
At least some basic concepts like direction vectors, velocity, projections on x and y axes, how to handle collisions between a ball and a block
@Array
What's your level at all in this
Yeah I am just starting, not 100% sure what to implement at the start
I have done another game using Vectors called Asteroid Shooter
But it wasn’t using classes or OOP just done in the main Form1.cs
I thought I would get started with a menu bc it is the one with no components of the actual game
Is it being OOP part of the class?
In my class, I had the prof make us use inheritance to make tetris. I just didn't do it, because it's stupid
Hopefully you're not forced to use inheritance at least
Inheritance is really bad for games specifically
Unfort I have to use the Breakout library in the Form1.cs window, it's out of my control 😦
Yeah
We learned it this year
By the way I'll upload the code I did with the asteroid shooter, involving vectors
Sorry for such a long window of time before my responses, my sister graduated Uni today!
So I was out for most of the day
@Anton Here's how I did it without OOP making an asteroid shooter type of gam
I used vectors here so I am degree with them to a degree
Or does anyone have suggestions for what I could put into the menu class
Like I am just not sure what to put specifically or how to make it
any help much appreicated stuck
Just write the game in a single file and split things away when you find it makes what you're doing easier
Do you have to use polymorphism?
If by OOP they just mean methods on objects, it's easy
If they mean polymorphism as well, you'll have to abstract more things
Maybe like a drawable abstraction for displaying objects or whatever
And you'll likely want to separate the view and the model
that is, display logic from the game logic
Just methods that deal with scene transitions I imagine
The idea is probably that you have the logic that does transitions separated from the logic that builds up the ui
It’s kind hard to do that given the template tho like I have to use things like the internal class, menu system, and enum in the way he’s done it
@Anton thoughts so far?
ok
write some more code
there's nothing to abstract yet
Yeah I need to work on the other classes
I was just wondering for the Menu class is this fine
I'll try working on the paddle class
@Anton
Thoughts on my paddle class
I've shortened it a bit
just store the rectangle
keep the brush around in a field
but I'm not a fan of mixing up game logic and visual logic
You think I should just put everything in the main form?
No, this ideally needs a redesign
Separating logic from view
But don't focus on that, because it's more complicated than just moving some code around
Keep doing what you're doing
@Anton
have a small question but remember how I said the game needs Bricks that never disappear
• Bricks that require multiple hits in order to disappear (maybe change colour)
• Bricks that change the ball speed or size (speed up or slow down)
• Bricks that drop items when hit that when caught by a paddle, a paddle effect is added (such
as longer or shorter paddle, ability to fire bullets at bricks)
would i code that in this class
I have a brick and a bricks class, here is brick:
this is bricks
there are 2 classes - one called Bricks and one called Brick - one of them has like a 2d Array of bricks as a private variable (bricks) private Brick[,] mBricks;
The other is just Brickbut this one is a better weird cause it has the enum public enum BrickTypes { None };
I'm guessing Brick is used to like identify which bricks drop items through the enum?
Whereas Bricks is to paint the objects on the board
is that right
you're probably right
though this approach isn't oop at all
you should have an interface for applying the effect on destruction
and define an implementation for each of the behaviors
and then make the brick contain one of these in a field
that would be oop
but don't subclass Brick for this, that's dumb
Yeah it’s kinda strange since I can’t add anymore classes 🫠