Acces data from another form
I don't understand why in this example it doesn't show the name of the car, if someone can explain it to me in more detail or tell me how to make it work. It only appears that,, the car is,,. Thank you very much! https://paste.ofcode.org/35K3CPDgRTAqSEnwsaWGB57
72 Replies
because car on Form2 is a complete new instance of car
you are creating 2 separated instances of car on each form
leowest
REPL Result: Success
Console Output
Quoted by
<@1102729783969861782> from #bot-spam (click here)
Compile: 520.063ms | Execution: 57.166ms | React with β to remove this embed.
in order to share the same instance of car between both forms you need to pass that instance of car from FormA to FormB, be it via calling a method or other means.
The most common way is called dependency injection, where u pass it via the constructor as a requiriment of the FormB.
and to pass it you would do something like:
@Ale does that make sense to u?
OMG it s works sjdnfsakdfjnsakf , how i find this in internet to seach dependecy injection
thank you soo much
$di
Dependency injection is really just a pretentious way to say 'taking an argument'
See: http://blog.ploeh.dk/2017/01/27/dependency-injection-is-passing-an-argument/
Dependency injection is passing an argument
Is dependency injection really just passing an argument? A brief review.
might be a bit too much to read let me see if there is a simpler version
ah there we go this one is a bit easier to explain it https://www.tutorialsteacher.com/ioc/dependency-injection
the name is a bit scary but the concept as I explained above is really simple
and above that u also have libraries that further implement DI which is a more complex concept
it really seems very difficult. Thank you very much
I'm already waiting to look over the documentation you sent
If you have no further questions, please use /close to mark the forum thread as answered
I have another question related to this topic, if I have the form1 class and then I have form2 and I need those parameters in form3, do I do the same?
form1 for form3
yes
what is form3
another form class where I want to use parameters from formA
and among the classes there is the form B class
Depending on whether u want to access formA method and car, u could instead pass a reference of formA
which would give u access to both too
so instead of say
You would do
and in Form1 u would have Car as
so u could access _parent.Car.Name or _parent.Class1.Message or some method u made public in Form1
u might also want to use ShowDialog instead of Show so that the user needs to finish interacting with Form2 before it can do any action on Form1 again etc
but if I don't need parameters from FormA in Form B, I still can't jump directly to FormC, right?
what u mean jump to formc?
in order to have formc u need to initialize formc and call show from somewhere
be it program.cs or forma
it is a form that separates the form1 or formA from where I need the values ββform3 or formC https://paste.ofcode.org/nTgXXEdYTi25vAUwNwpZYD
i make an example similar but i add a form class between two forms
can u like show some images?
it might help me provide a better answer
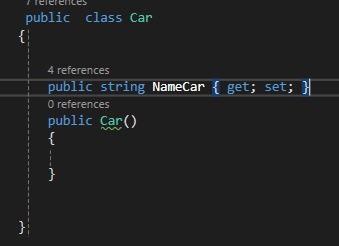
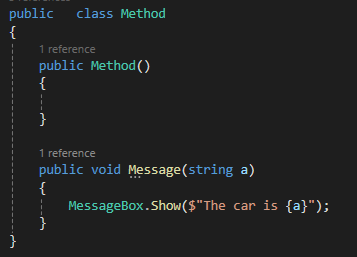
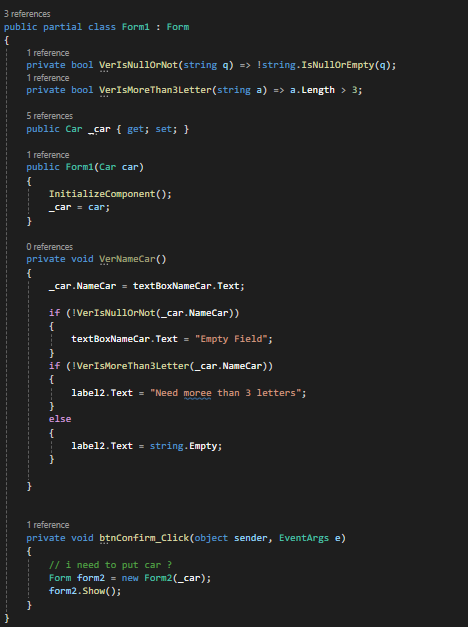
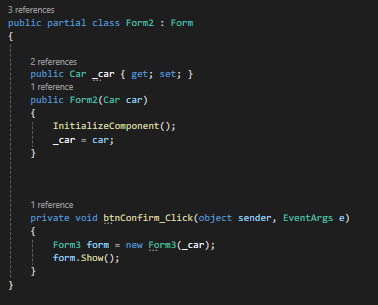
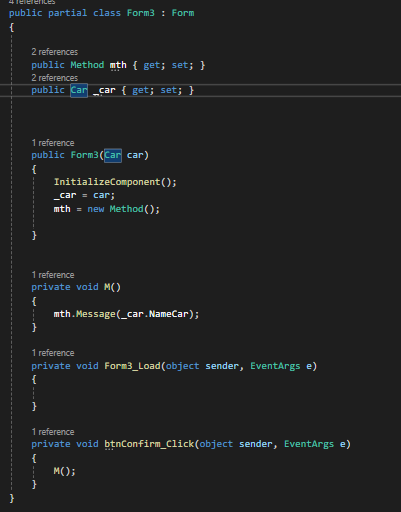
no I mean images of your forms
not the code
because all this chaining of a to b to c
could have been different depending on what they are
could have been just like a menu with a panel that replaces usercontrols
instead of opening new windows
there are many ways to do things
from now on I say it's not who knows what
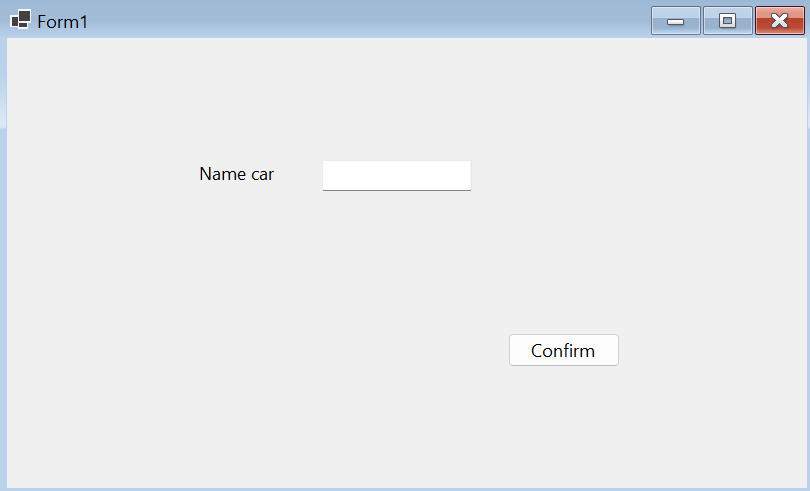
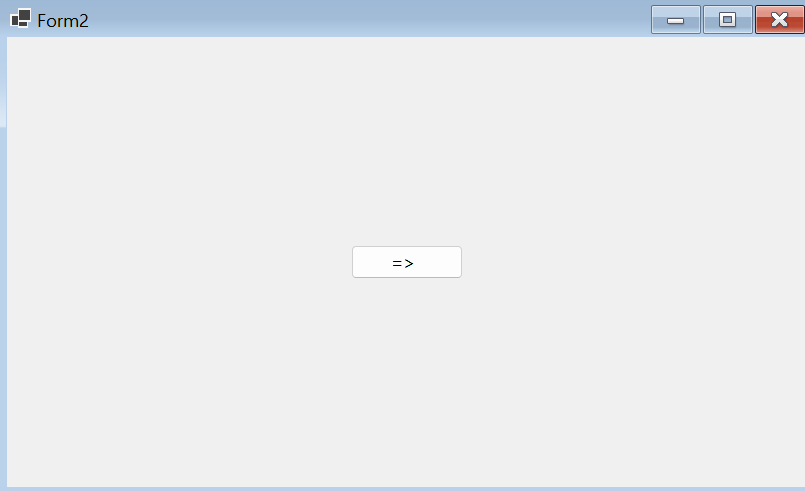
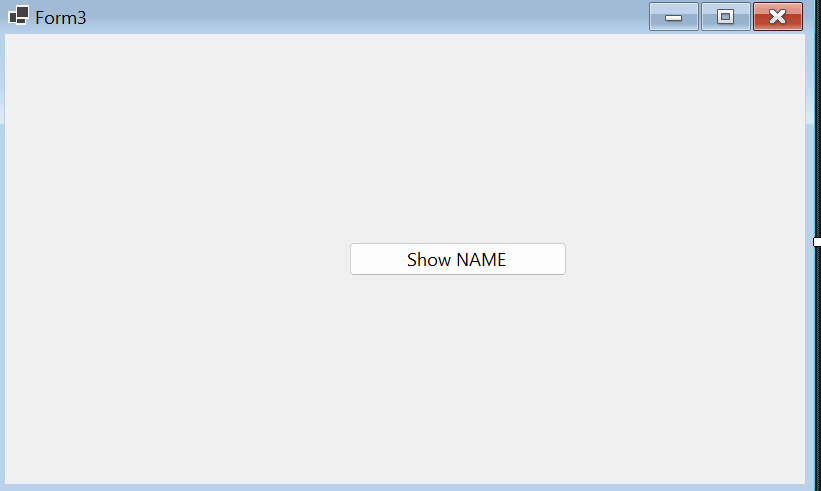
some make more sense then others as being intuitive
so they are like pages to u? u click confirm, it should show the arrow to next?
yes
imagine this then instead of window
u create UserControl
then u add a panel
you do something like
u press confirm, now it changes from InputForm to say next page
this would all be working in a single window
as if u were walking pages
note: this is just hypothetical code, I have not tested I dont usually use winform, but I know its possible
Well, I intentionally put 3 pages because I don't know how to transfer the data from page 1 to page 3
I know that I could do better on one page
but I can understand the transfer of data when a page does not need that data and is fixed in the middle. It seems very complicated to me
u mean this
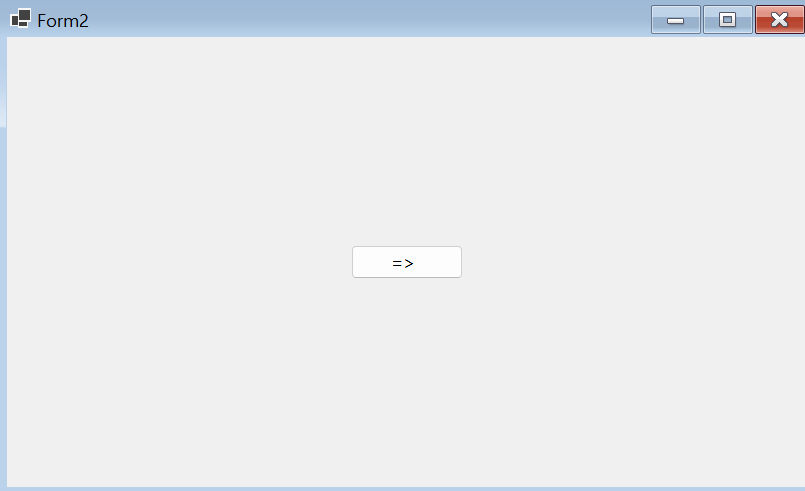
I honestly dont understand why u have it
yes this is the middle page
why can't u go from A to C
umm idk π¦
:waaaaaaaaaahhhhhh:
it would make more sense no?
even if u reverse yoru forms
first form having say a label
and a button called create car
yea i know
70 / 5.000
but I wanted to know how I can do this if there is an extra class in the middle
and once u create the car on the form2 it returns to form1 and upsate the label with the car name
ignore 70/5000
by extra class you're referring to class Method?
I do this thing above because I want to make a project and I made a sketch in my head in which the first form enters some data after the second form does something else and the third form I need data from the first form for a method
form2
well because you're going into a chain
i.e.:
u open form2 from form1 and form3 from form2
then yes u need to chain passing that reference unless you create a singleton of car which I am not sure I would recommend at this point
but if u use, usercontrol as pages and panel then it would be slightly different
so it is not done as shown the first time?
this is sort of what u have right now
that's how I want it to work like you did above
then yes u need to do what I explained earlier
that is what I am doing above
can you show me the code pleaseeeeeeeeeeeee
I already have
π
i made this in formA var formB = new FormB(car);
formB.Show(); and in form B i put this var formC = new FormC(car);
formC.Show();
yes
this is what the other way looks like
without using multiple windows
this is using UserControl and a single window
the process is similar to what u do with window in terms of passing the car over, but it all exists in a single window and feels more consistent as if u were navigating from page to page
and you also put the instance of car here, right?
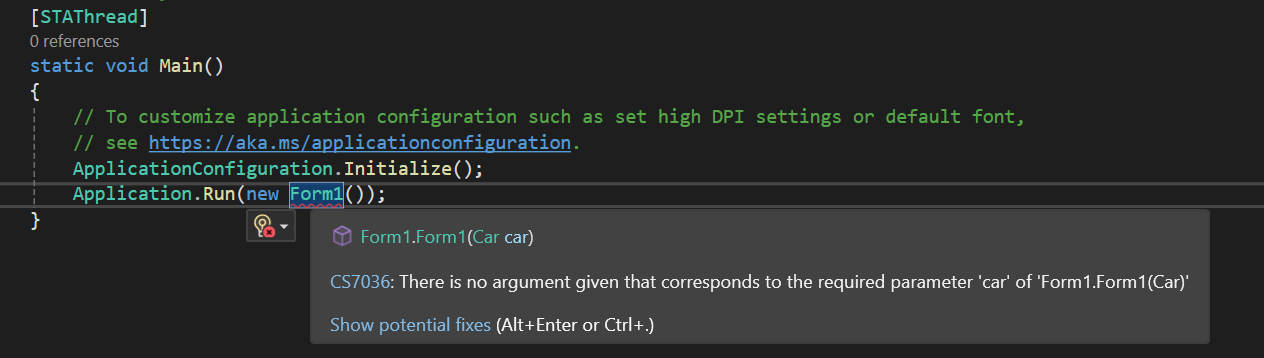
no
u dont change Form1
only Form2 and 3
Car already exists in Form1
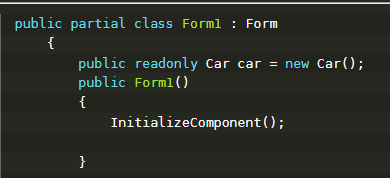
u can remove the readonly on Form1
leave on the others
i dont know what i do but don t show the name of car
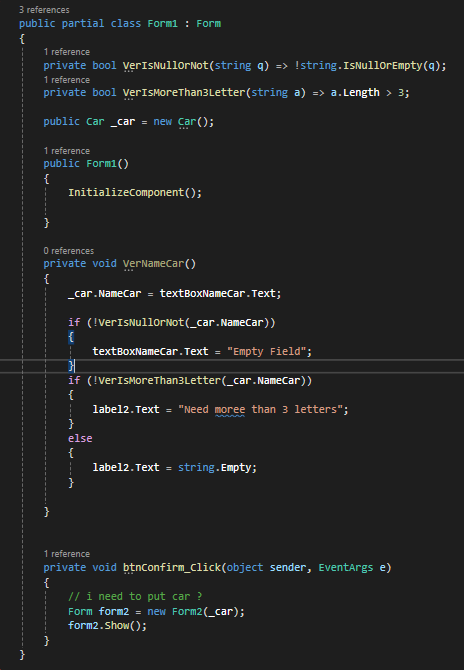
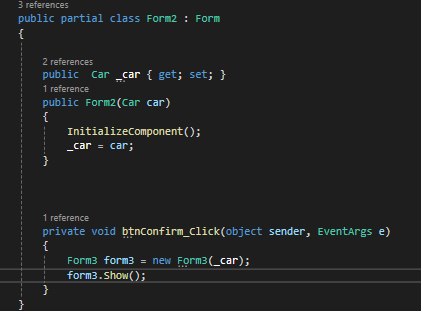
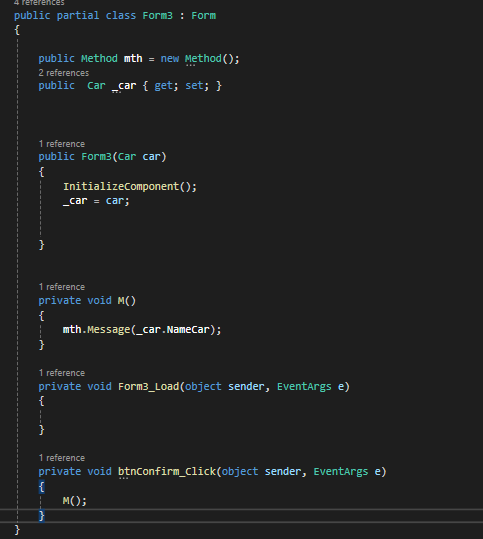
looks fine
what I would change is
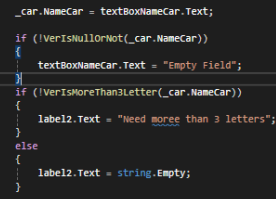
dont do this
u assign textBoxNameCar.Text to _car.NameCar before testing if textBoxNameCar.Text is safe to use
don t appear the name of car
can you show me the Car class
when i press the button show name
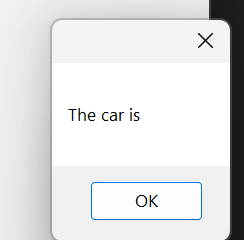
yes I understand that, I am trying to help u by fixing the things I see that are wrong
such as the ones I pointed above
leowest
can you show me the Car class
Quoted by
<@1102729783969861782> from #Acces data from another form (click here)
React with β to remove this embed.
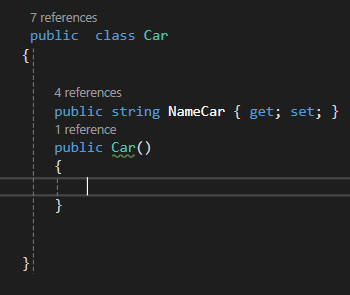
ok looks fine...
u forgot to
?
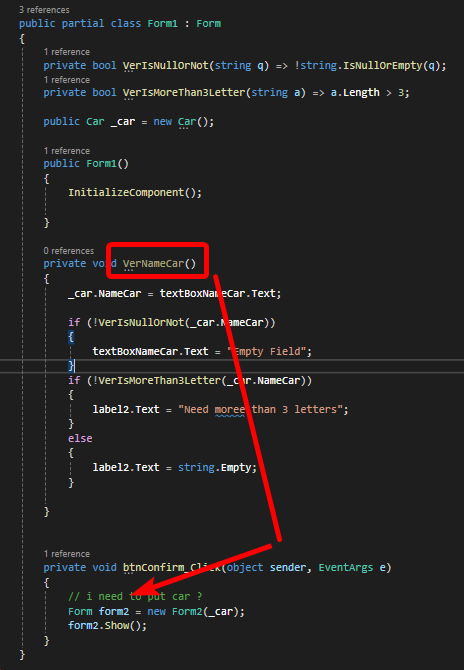
VerNameCar is not being called
so it never sets the car name when u hit confirm
:catderp:
damn i forgot :Smadge:
:waaaaaaaaaahhhhhh:
anyway I will be back in 20 minutes need to leave my seat
it worked, thank you very much for everything :catHeyHello:
np π
If you have no further questions, please use /close to mark the forum thread as answered