Trouble implementing Identity
Hi so basically, I'm making a asp .net core web app and i want to use JWT and Identity (cause i heard google api for login, opts,.. are good)
Thing is, the Database has already been constructed before hand and used scaffold to generate them in my .net project
Therefore I already got a User entity. I watched some vids on yt guiding that I should extend the IdentityContext, IdentityUser, write stuff in program.cs files and how to use them in controllers.
But these 2: IdentityContext, IdentityUser i've only extended, that's it
One more problem is that, some recommended creating ApplicationUser? but I already have my User so is it needed?
I then made my own login controller, and ran on swagger. It returned this
{
Invalid column name 'NormalizedUserName'.
Invalid column name 'Id'.
Invalid column name 'AccessFailedCount'.
Invalid column name 'ConcurrencyStamp'.
Invalid column name 'EmailConfirmed'.
Invalid column name 'LockoutEnabled'.
Invalid column name 'LockoutEnd'.
Invalid column name 'NormalizedEmail'.
Invalid column name 'NormalizedUserName'.
Invalid column name 'PasswordHash'.
Invalid column name 'PhoneNumberConfirmed'.
Invalid column name 'SecurityStamp'.
Invalid column name 'TwoFactorEnabled'.
Invalid column name 'UserName'.
}
Now some told me that it's DB related and suggested I migrate add Identity and then update the Database
but then I got errors from the tables constructed before
Is Identity really needed for outside apis like google,....?
If yes, how should i fix this?
If no, what should I do instead?
70 Replies
Handling auth on your own is a complex thing and not recommended especially when you're the begineer. Identity is a good platform to handle authentication and authorization in your .Net Projects.
You should delete your custom auth implementation and use the one that come with Identity . And yes as you know, if you wanna customize the user i.e wanna add additional properties you can simply extend the
IdentityUser
entitythose errors mean your EF model doesn't match your database structure
Also by using Identity , you'd get a lot of endpoints and pages for the auth ready made so you'd spend less time in managing auth related stuff in your application.
yes that would very much be more convenient
I have tried to extended IdentityUser
I think the problem would be about the database that has been constructed beforehand ?
cause I am unable to update database after migrating
what do you mean by the database is constructed beforehand
by migrations?
I don't really know whether I should just redesign the DB or find another method
no no
if your database hasn't much data already , then you need to delete the previous migrations and add new ones
or even delete the db
you should be using migrations
we were tasked with making the DB first thus creating seperately in the sql server
then we scaffolded it into the .net project
also can you show the new User class that you're extending from the
IdentityUser
?identity and scaffolding are a really bad mix
because identity tables are defined code-first
trying to copy it by hand is error prone and a poor use of time
it's a mess sr
that's wrong. You're also defining those properties that already exist in the Identity User table or model. Please remove them and specify only those that don't exist in the Identity's User model.
I see
here's the corrected model
but that wouldn't mess up the relations in DB right?
what relations
also listen
what the orders, customers and all that stuff need to do with the User entity ? You need to create separate entities for them and don't mix them up with the User.
those relationships look weird to begin with, why so many to the same type of entity
also virtual is bad, that suggests lazy loading which you shouldn't use
they are other entities that are in relations with User. There are seperate entities, but arent these required if one is a Foreign key for another?
These were basically auto generated based on the DB my teamates made. "virtual" and the ICollections included
i'm going to guess you have normalization issues in your database design
for example you probably shouldn't have 4 different tables for orders, but a column to discriminate order types in a single table
something feels off with your database structuring
can you describe your complete current database design ?
yes, lemme go get it
running the whole thing should create the DB smoothly
in SQL server
we're not asking about the literal sql really, more the reasoning behind why certain tables exist
also i can't download files, use $paste if you can
If your code is too long, you can post to https://paste.mod.gg/ and copy the link into chat for others to see your shared code!
that's a lot to read but you could describe it in an easy and simple way
i.e you could list the name of the entities, their purpose and the relations among them
alright, ill try my best cause there's a lot
so the entities are: User, Role, CustomerRequest, Order, Message, 3DDesign, ProductSample, Collection, Gold, Gemstones, Category, PaymentMethod and Insurance
This is a web app for JewelryProduction: make jewelry based on customer's demands
Message: messaging between Users (customer, staff...)
3DDesign: the design of a product, required in Order, ProductSample and Request
ProductSamples: product on display
Gold: used to make product and provide price range. Needed in CustomerRequest and ProductSample
Gemstone: diamonds,.... for production, needed in CustomerRequest and ProductSample
Role is just an entity to categorize Users like customer, manager,...
CustomerRequest: A description of the desired product/ A form for customers to provide desired properties for a product
Order: Once the request is done, an order is then created.
Collection for ProductSamples: basically like Category for Product Samples
Category: category for Gemstones
Insurance: Just documents for Orders
Payment method: via Banking, implement sandbox
it's ... complicated
like order requires both User (customer) and User (staff)
1. Why
3DDesign
is required in Order
. It makes more sense in Customer Request
like when customer would request a jewelry of certain design.
2.Instead of Product Samples
I'd rather call it Products
that would be displayed or used across the applications.
3.Collection
entity doesn't make any sense to me here. Category
is also a collection.
4.User
, Roles
and similar entities will be handled by the Identity mostly and you'd customize them according to your needs as discussed previously.
5. Payment Method can just be an enum instead of a class
also for the relationships, you'd need to think very carefully . You don't wanna relate entities in a wrong way together. Think of them like each user can have many orders but each order can belong to a single user so it's a many to one relationship. Many Orders - > 1 User. So in this way try to relate all the entities together.
Personally, while designing schemas and apps, I take help from the GPT as well to figure out the relations and stuff. It's not guaranteed to produce a perfect solution but can be helpful and gives you some pretty good ideas sometimes if asked correctly .Thanks for the reply.
1. The flow is that the request is like a form that customers fill in, like the requirements. Then it is sent to the staffs to evaluate and provide quotation. Only after the quotation have been approved by both parties will the order be created. Now the 3ddesign is required in order as it has not been finalized, so the Design Staff might exchange suggestions with customers for modifications
2. It does make more sense, ill do that yes
3. Collection is like a collection of products related to each other. Like when there's a Summer 2024 Collection that has a necklace, bracelet, ring,... Whereas Category is used to categorized the gemstones only. This could be the Naming that might be confusing
4. I have noted this. So I should only provide properties that aren't in Identity. But we did a db first approach and we had to add necessary attributes to User. Does the part where u suggested I remove properties that exist in Identity model be used in Code only or both Code and DB?
5. I will take this in thanks
If the Identity already has User and Role, then should I still include them in the db before i migrate?
Before Migration, the only thing you need to do is to configure the Identity and roles properly. Like registering the Identity in the Program.Cs, in the DB Context and Customizing the User as discussed before. Nothing else. You don't need to manually add something to the db . When you'll add the migration, Identity will create all the necessary auth tables for you.
And it doesn't matter wether you created the database first or not in this case .
GitHub
GitHub - salmanhaider14/EcommerceWebApiDotNet
Contribute to salmanhaider14/EcommerceWebApiDotNet development by creating an account on GitHub.
Look at this API that I created, see how identity and roles are configured .
Note that this is a web API, and identity config can vary slightly based on the project template you are using i.e blazor or MVC etc.
I think I understand it a bit now after seeing your project
I think im gonna redesign my db
and I understand where i went wrong in my project now
1 question
look at the Order - User pls
let's say the order needs involvement of the customer, sale staff, manager, production staff
then my peers drew up to 4 relationship indicating those 4 relationships, this is still correct right?
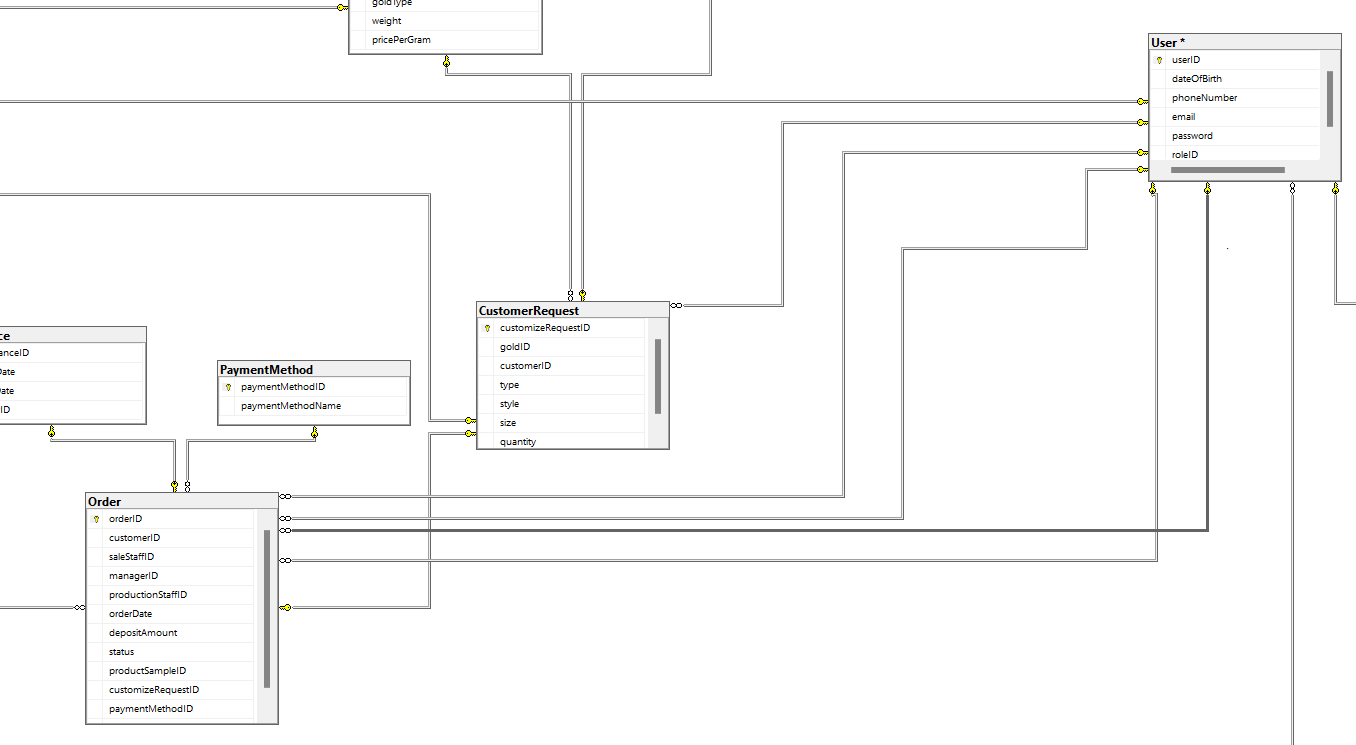
another one is this
if i want a customized User table that uses AspNetUsers
then i should make AspNetUser have a 1:1 relationship with Users right?
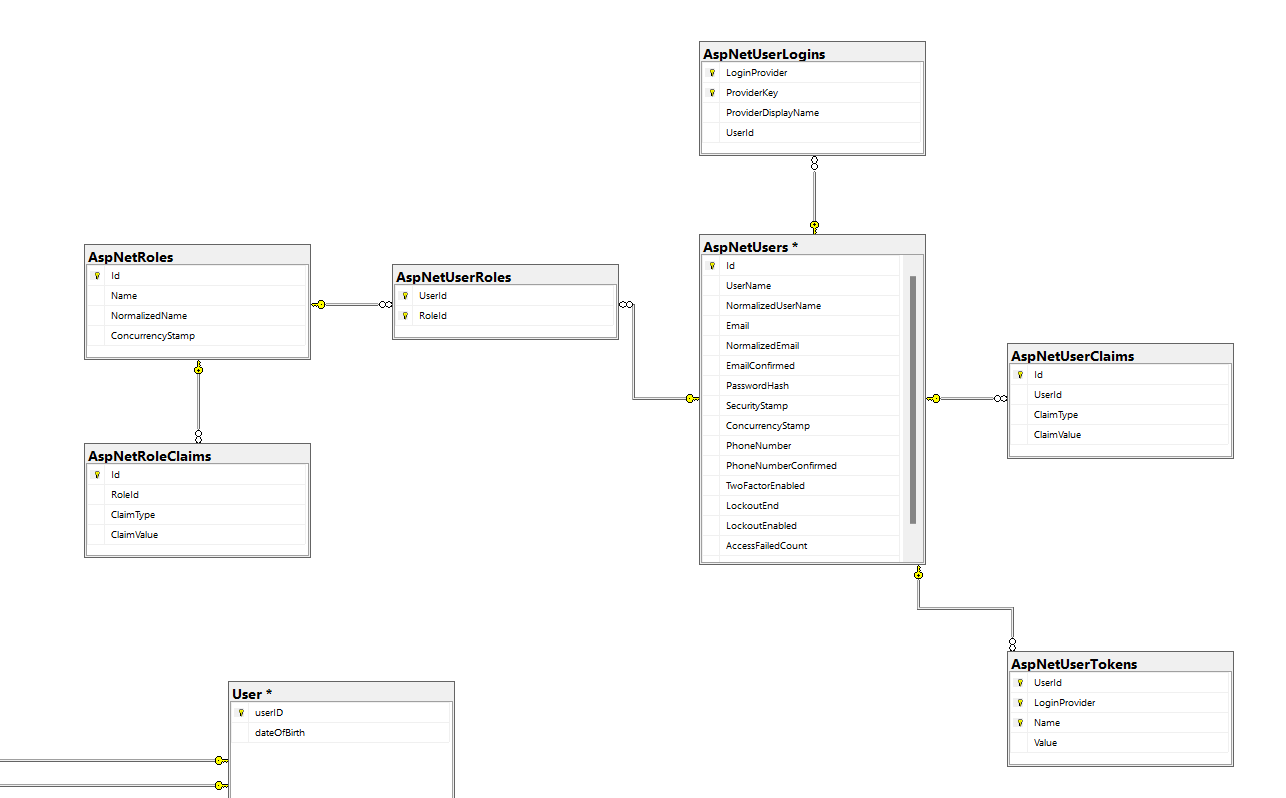
I mean what kind of involvement all those roles would require in Order ? And how you want those roles to interact with Order in the application.
Users and AspNetUsers are the same thing. You don't need to do that. You just extend the Identity user, add the additional fields and that's all .
Ah yes so like, customer can obviously track their order right? Then the sale staff can and manager should also be able to track it (manager is optional of course). And then the production staff should be able to update the status of the order for instance like whether the product is done with the body, done with the gemstone or whether it is done or stuff like that.
ah so in db i should just scrap the User and use AspNetUsers directly. Whereas in the Code, I can do a User class which extends IdentityUser right?
u might be asking why the sales staff is involved, we intend to make them like the jack of all trades. Involving with the process of a jewelry production process from start to finish. Basically like customer service
The simplest approach can be that you make multiple roles i.e Customer, Staff etc. And then set the authority i.e Customer can view their orders and track it. Staff Role can not only view the order but update the status as well . And yes for this simple approach you would need to add multiple Foreign Keys (FK) in the Orders table pointing towards each role.
I see, then I guess we correct on that part
if i do this approach, then would the User identity as the AspNetCore in the db?
When you'll make a new user class that'd extend the existing IdentityUser, now this new class will be used in the code i.e In the DB Context and in the Program.cs etc. And to access the user and get the information. You would use the apis provided by the Identity .
Are you making a Web Api ?
ah yes i am
what will be the frontend in ?
React
fine
then make sure you Apis are consistent and well organized so that the frontend can consume it easily
I'll try yes
At first, i didnt use Identity but only JWT in which we tested it on the FrontEnd side. It ran fine. It's just the Identity that confuses me
also IdentityApiEndpoints are not customizable, as you can see in my project I had to make my own register endpoint to add the roles support. Because by default the
/register
endpoint of Identity doesn't support adding roles.
There's nothing confusing when you understand it .only at first yeah, the tutorials on yts are kinda diverse with different methods and stuff so it was confusing
One separate approach can be that while registering the user on the frontend weather it's the customer or staff. You send the request to the api and the api will assign the role to the user. So instead of making another
/register
endpoint you can rather make a /assignRole
endpoint that will take a user and role and will assign that role to the user .Patrick God
YouTube
.NET 8 Authentication with Identity in a Web API with Bearer Tokens...
🚀 Join the .NET Web Academy: https://learn.dotnetwebacademy.com
💖 Support me on Patreon for exclusive source code access: https://patreon.com/_PatrickGod
🚀 Get the .NET 8 Web Dev Jump-Start Course for FREE: https://dotnet8.patrickgod.com
🐦 Let's get social on Twitter/X: https://twitter.com/_PatrickGod
🔗 Let's connect on LinkedIn: https://www.li...
It's a very good tutorial .
i havent seen this one yet, will do thanks
i'll try to update once I finish modifying them
Identity model customization in ASP.NET Core
This article describes how to customize the underlying Entity Framework Core data model for ASP.NET Core Identity.
Official Guide for customizing Identiy User and other configurations and models .
oh sr, 1 more question
sure
public class Order
{
public Guid Id { get; set; }
public required Guid UserId { get; set; }
public required DateTime OrderDate { get; set; }
public required OrderStatus OrderStatus { get; set; }
public IdentityUser? User { get; set; }
public required List<OrderItem> OrderItems { get; set; }
}
how did u make the relationship between User and Order?
was it the line public IdentityUser? User { get; set; }? cause i didnt see the relationship in the AppContext either
If I were to make a customized User class then i should also add it into both the order and the AppContext right?
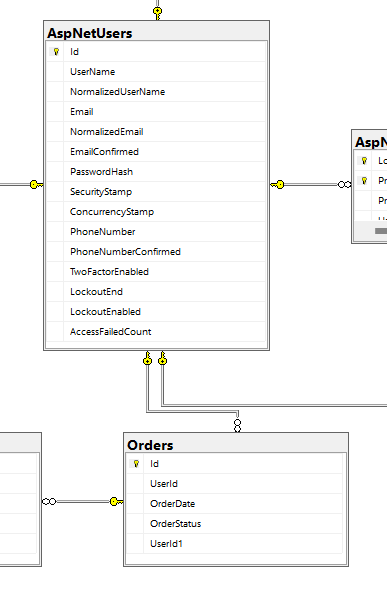
In the Orders table I'm adding a FK
UserId
which points to the User to whom the order belongs. And IdentityUser
is the navigation property that EfCore will fulfill automatically for you . So this is a relation . And if you're making a new User then instead of IdentityUser
you'll use the newly created user class. To be sure, you need to look into the official guide once .
and in your newly created user class you'll add this property as well like :
i see, but wait does mean that the IdentityUser navigation property automatically recognizes the UserId as the fk?
I'll recommend you to must go through this official guide for relations once :
https://learn.microsoft.com/en-us/ef/core/modeling/relationships
Introduction to relationships - EF Core
How to configure relationships between entity types when using Entity Framework Core
in your situation of course
yes , Identity automatically knows that
User
and UserId
are related. You need to name your properties like that to left no room for confusion for Identity .holy, that's neet
And to be more sure you can define your relationships manually in the DbContext class like I did but that's not required if your entities are well made .
i see i see
this is a must read blog
I'll go read it right away
thanks a bunch
np