LINQ
A sequence of non-empty strings of Latin alphabet characters stringList is given.
Among all strings of the same length, sorted in ascending order, select the last character from
each string, converting it to uppercase, and from the received characters, compose a string.
Arrange the resulting sequence of strings in descending order of their lengths.
46 Replies
I have been trying to solve this for quite some time to no avail, need help!
Sounds like homework. what have you tried?
It is, The closest I have got was this
return stringList
.GroupBy(s => s.Length)
.OrderByDescending(g => g.Key)
.Select(g => string.Concat(g.Select(s => char.ToUpper(s.Last()))))
.OrderByDescending(s => s.Length);
But in this version they are ordered according to ascending list when they should be descending
So do
OrderBy
instead of OrderByDescending
?Nahh now the resoult isnt even simialr to expected one
I guess you want to sort the group tho
Individually
g.Key
is length not stringSTEP 1: Select the last character from each string and convert it to uppercase
STEP 2: Order the original list in descending order
STEP 3: Create new strings by combining the last characters from step 1 in order from step 2.
Thats how i see the task, im quite new to this so i don't know the syntax that well
Again you want to sort individual group by string, not groups by length like you are doing rn
i.e. You'd want to sort each
g
they should be soretd by strings length
stringList: new[] { "bc", "sd", "ac", "sdf", "ewr" },
expected: new[] { "CCD", "RF" });
That's for results
Which is
.OrderByDescending(s => s.Length)
there
What is wrong is .OrderByDescending(g => g.Key)
You are basically sorting the IEnumerable<IEnumerable<string>>
, not individual IEnumerable<string>
return stringList
.GroupBy(s => s.Length)
.Select(g => string.Concat(g.OrderBy(s => s).Select(s => char.ToUpper(s.Last()))))
.OrderByDescending(s => s.Length);
?
Btw how do you darken the code part?
Try and see
with backticks
`
Okey, the first one works
what's the first one
yield return (
stringList: new[] { "bc", "sd", "ac", "sdf", "ewr" },
expected: new[] { "CCD", "RF" });
yield return (
stringList: new[] { "du", "the", "ace", "run", "cut" },
expected: new[] { "ETNE", "U" });
yield return (
stringList: new[] { "ab", "attribute", "cheese", "swim", "cut" },
expected: new[] { "B", "E", "E", "M", "T" });
yield return (
stringList: new[] { "star", "galaxy", "quasar", "planet", "asteroid", "satellite", "comet" },
expected: new[] { "YTR", "D", "T", "E", "R" });
And what doesn't work?
yield return (
stringList: new[] { "bc", "sd", "ac", "sdf", "ewr" },
expected: new[] { "CCD", "RF" });
yield return (
stringList: new[] { "du", "the", "ace", "run", "cut" },
expected: new[] { "ETNE", "U" });
yield return (
stringList: new[] { "ab", "attribute", "cheese", "swim", "cut" },
expected: new[] { "B", "E", "E", "M", "T" });
yield return (
stringList: new[] { "star", "galaxy", "quasar", "planet", "asteroid", "satellite", "comet" },
expected: new[] { "YTR", "D", "T", "E", "R" });
this the orignal but it doesnt make sense
be cose it has T in swim
yield return (
stringList: new[] { "bc", "sd", "ac", "sdf", "ewr" },
expected: new[] { "CCD", "RF" });
yield return (
stringList: new[] { "du", "the", "ace", "run", "cut" },
expected: new[] { "ETNE", "U" });
yield return (
stringList: new[] { "ab", "attribute", "cheese", "swim", "cut" },
expected: new[] { "B", "E", "E", "T", "M" });
yield return (
stringList: new[] { "star", "galaxy", "quasar", "planet", "asteroid", "satellite", "comet" },
expected: new[] { "YTR", "D", "T", "E", "R" });
$code
To post C# code type the following:
```cs
// code here
```
Get an example by typing
$codegif
in chat
For longer snippets, use: https://paste.mod.gg/cs
// yield return (
stringList: new[] { "bc", "sd", "ac", "sdf", "ewr" },
expected: new[] { "CCD", "RF" });
yield return (
stringList: new[] { "du", "the", "ace", "run", "cut" },
expected: new[] { "ETNE", "U" });
yield return (
stringList: new[] { "ab", "attribute", "cheese", "swim", "cut" },
expected: new[] { "B", "E", "E", "T", "M" });
yield return (
stringList: new[] { "star", "galaxy", "quasar", "planet", "asteroid", "satellite", "comet" },
expected: new[] { "YTR", "D", "T", "E", "R" });
$codegif
Better
4th one
Anyways I wouldn't say it is "wrong" since the order is descending by length and they didn't mention tiebreaker
It's just undefined there
I mean it's strange because i have to take last character, but now it expects T but returns M
So in place of T i have an M, I mean if the first case worked why not here
I'd say "B" "E" "E" "M" "T" is preferrable as it maintains the order 😛
But does not matter by the spec
They both right
Whoever made that test case did the bad job
no even if I change this one the next one is even worse
That also doesn't matter
As long as "YTR" is the first element
The rest is same length so order doesn't matter there
But wait it doesn't make sense, the task states that the lists are ordered in ascending order which they are clearly are not
I assume that's just saying you need to sort it which you did
Then what's the issue
That they did the bad job making test case?
Nahh, there has to be a solution
which satisfies the parameters
Ehhh good luck, not an interesting guessing game
Okey I have changed the test case lets see if it goes thru
Whatever those all should be considered "correct" when requirement is just "Arrange the resulting sequence of strings in descending order of their lengths."
Alright thanks
Yo, I have figure it out, if multiple groups have the same length they should maintain their current order which is alphabetical, the problem is i have got no cluq how to implemnt it using only System.Linq
So they do want you to sort things alphabetical order first?
Well take that
OrderBy
out of Select
and put it before GroupBy
then
Probably should've done that in the first placenahh
I do not belive this
whole this time
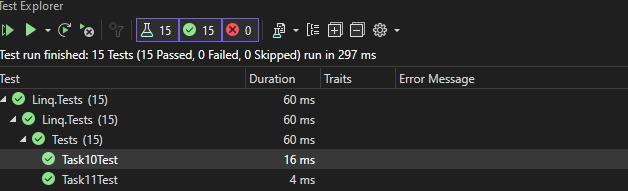
God bless you and your soul
Lol well done