websocket issue
in this example, the onClose wont work, because the ws isnt the same object as in the array, how do i even identify the the websocket to remove from the array
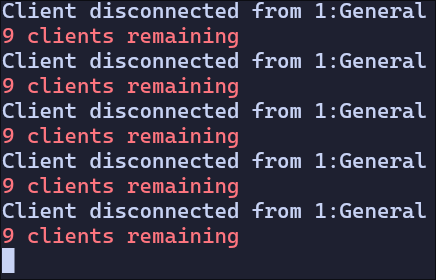
17 Replies
@Micha also needs help with this
@blurSkye π΅πΈπ
I guess, I found a workaround.
I tried to store the
ws
object in a local variable _ws
to create a closure like behavior. _ws
can then be used later on in every other event like onMessage
or onClose
.
It doesn't seem like a general good solution, because we just omit the provided ws
object in the other events.
But as a workaround, it works π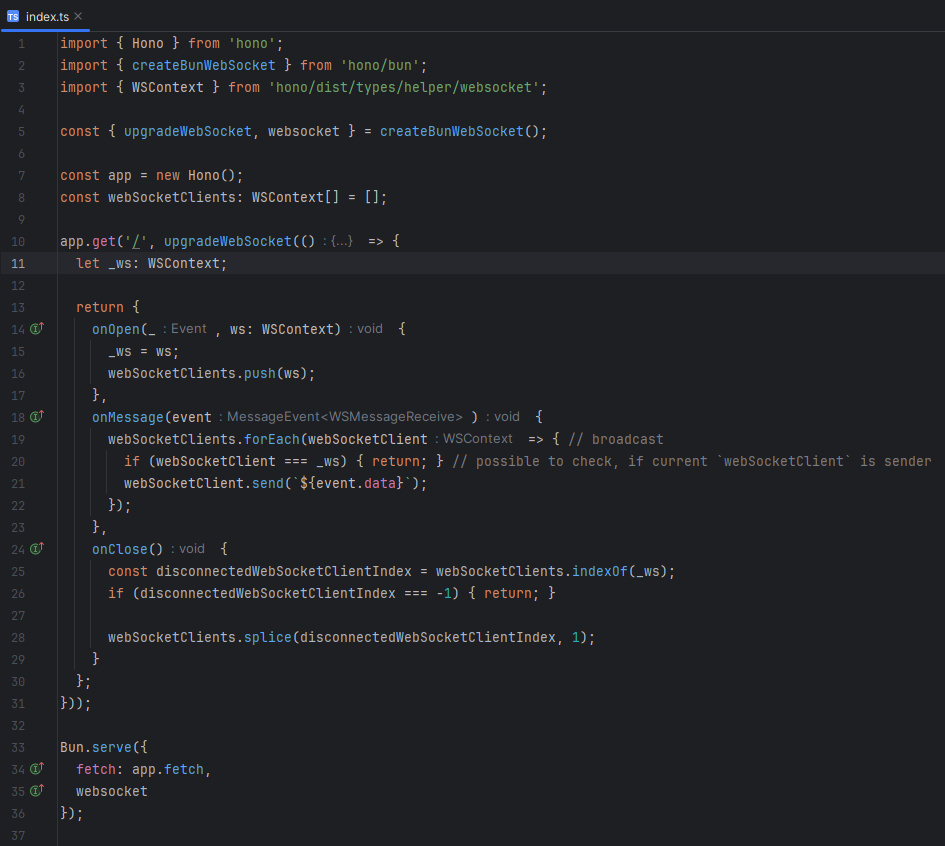
Will the local
_ws
object be garbage collected, when it's removed from the webSocketClients
array?If you are doing it in bun maybe it's better to use the pubsub? Here is simple example.
for reference
https://bun.sh/docs/api/websockets#pub-sub
Bun
WebSockets β API | Bun Docs
Bun supports server-side WebSockets with on-the-fly compression, TLS support, and a Bun-native pubsub API.
probably considering it is orphaned
That's exactly what I need.
Thank you very much!
@Michashiii, cant split app routes that use websocket into a different file
you get this error,
imagineeeeeeeeeeeeeeeeeee
i had the backend entirely written, now i discovery this
I also tried to put all websocket related stuff into its own file.
I exported
websocket
and imported it in my main file where Bun.serve()
is located.
I don't know if you have the same problem, but that worked for me.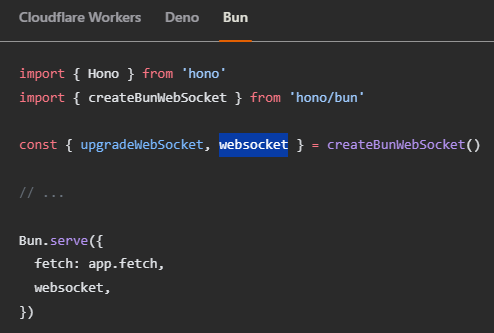
yes what if i have multiple modules using websockets
i do have that
π
Ah I see. In this case, in don't know how that would work.
here is a simple example(no need to do bun install, forgor to remove node modules π )
@Nico sorry to mention you randomly, i think you are a hono js developer?
how does websocket module spliting work? it appears we cant split code that use websocket into a different file
You can always ping me. Iβm not apart of the Hono team, I just moderate on here and help contribute. Iβm not too familiar with websockets on Hono Iβve only used them once. But I can give it a try later today. Also try asking on GitHub discussions, the core team checks that more often
ok found a way, damn how did i not think of this π
you need a file that defines websocket, and import upgradeWebSocket in the routes and import websocket to serve in the main.ts file
one of the interfaces needed for websocket wasnt exported sooo i copied all the interfaces needed π
Nice thanks