Websocket error when using Pulse
When I tried pulse it was working for some minutes but then it crashes with this error. I don't have any idea why this keep happening. Even increasing the event Listener, it still crashes
Btw, I am using Supabase as my hosting provider.
The error:
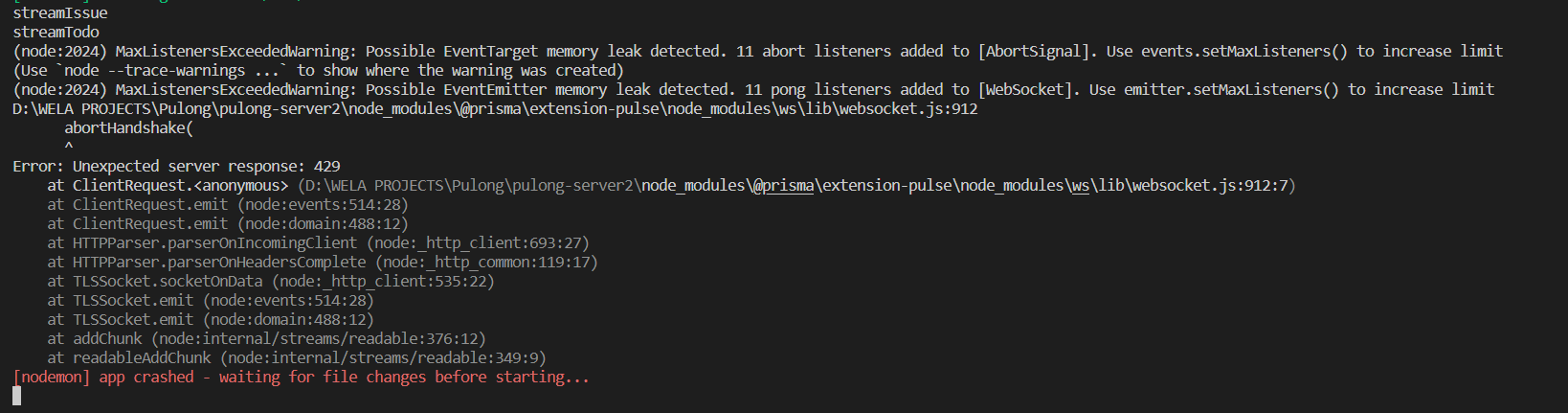
18 Replies
My code:
I just started using pulse last week, and that error kept showing. Whenever I started the server, the pulse worked for some minutes, then the MaxListenerExceedWarningError kept appearing and then crashed. @batmanwholaughz
this is how i implemented in my sveltekit app
@jisoon Are you still facing this issue?
yes @Nurul (Prisma) I still facing this issue
Is your repository open source by any chance?
I would like to run this locally to debug.
Also, are you using latest version of Prisma (5.15.0) and Pulse Extension (1.1.0)?
Also, what happens if you change
prisma.todo.subscribe();To
prisma.todo.stream();Do you get the same error?
yeah i still get the same error using stream();
i am using "prisma": "^5.14.0", and "@prisma/extension-pulse": "^1.1.0",
This error doesn't happen immediately, right?
It happens after a few minutes?
yes
this is the example server that i created https://github.com/COROTANjayson/prisma-pulse-test
GitHub
GitHub - COROTANjayson/prisma-pulse-test
Contribute to COROTANjayson/prisma-pulse-test development by creating an account on GitHub.
i actually just copied the example implementation from prisma pulse docs
Thanks! Let me try to run this locally with a supabase database and check if I can reproduce this.
To confirm, you were receiving a few database events successfully and then you got this event listener error, correct?
yes the pulse events are working
Okay, thanks for confirming.
I am trying to reproduce this
Hello @Nurul. May I kindly ask if there are any updates? Thank you!
Hey, Apologies for the delay.
This slipped from my radar. I’ll get back to this latest by tomorrow 🙏
I added 1000 events to test this, but I don't seem to get any errors.
I created a streamTest function similar to your streamIssue and streamTodo functions.
And created 1000 tests records like this:
After how much time did you used to get this error? Can you comment the socket part and check if you still get the same error?
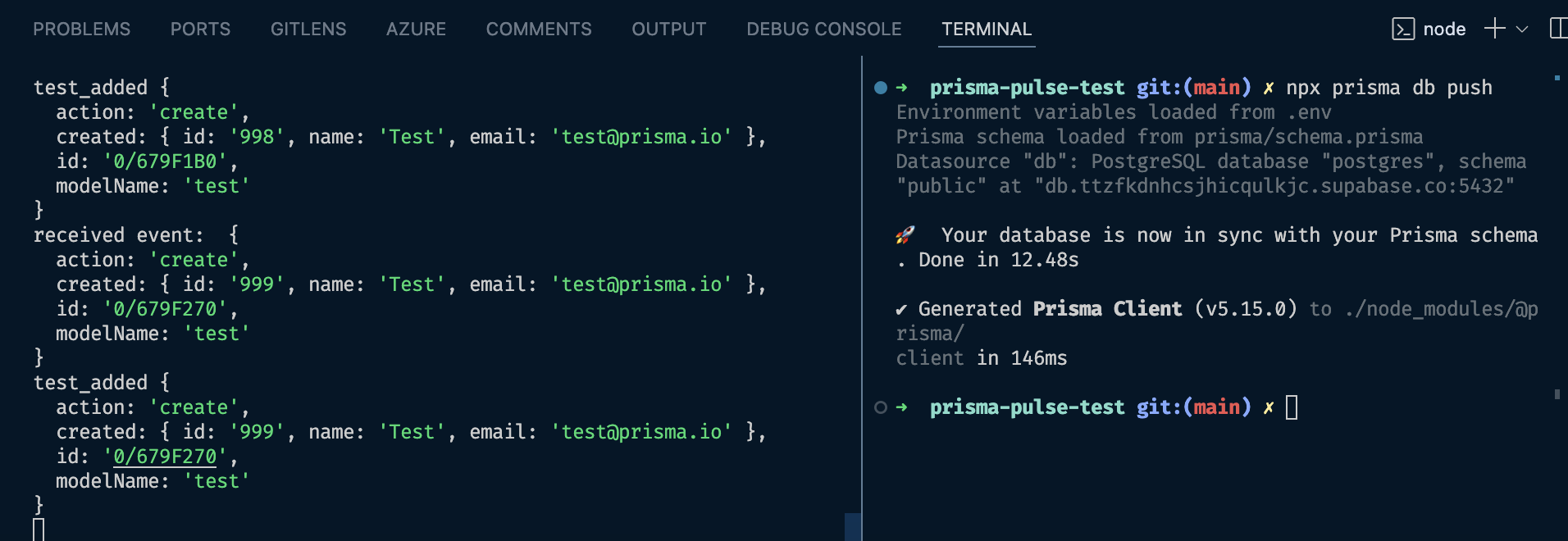
hello @Nurul (Prisma) sorry for the very late reply, I commented the socket part and there is no error. I think it only appear when the socket is on.
Thank you btw
No worries!
We will be releasing a new version of Pulse Extension very soon, that will improve the performance and alleviate the error you were observing. I'll let you know once it's out.
The new Prisma Pulse extension version 1.1.1 is out. Can you try this and check if you still get the error even after adding the socket part?
https://www.npmjs.com/package/@prisma/extension-pulse
npm
@prisma/extension-pulse
Prisma Client extension for Pulse. Latest version: 1.1.1, last published: 2 days ago. Start using @prisma/extension-pulse in your project by running
npm i @prisma/extension-pulse
. There are no other projects in the npm registry using @prisma/extension-pulse.