Extending a class while overwriting its properties
Hello, I'm trying to use MudCalendar and for this I need to use a CalendarItem. To make it possible to pass extra data I would like to extend my own DiscordEvent class, and followed by that whenever properties of Calendar Item are queried, retrieve data from the correct properties. I have done part of this already but it has ended with my having duplicate properties like in the picture below.
The duplicate properties have (Heron.MudCalendar.CalendarItem) behind them. How do I fix this?
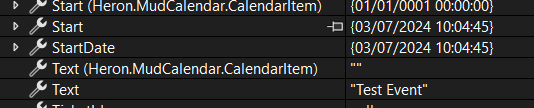
7 Replies
you arent overwriting the properties because of the new keyword you added to every property
So I've removed the "new" keyword and the result unfortunately remains the same.
are the properties you want to overwrite even virtual/abstract?
Apologies I forgot to include the CalendarItem class. None of the properties are virtual or abstract.
then make them virtual
when they are virtual you can use the keyword override in the subclass
@Nakama
Its not my class, its from the MudCalendar, a nugget package I am using
The calendar takes : Items IEnumerable<CalendarItem> The data to display in the Calendar.
https://danheron.github.io/Heron.MudCalendar/api/calendar#properties
then these properties werent intended to be overriden
so you would have to live with that the object has two properties with the same name, base and sub class
what oyu could do tho is to pass the changes of the subtype up to the basetype
eg.
why are you eventrying to override them anyway? just keep and use them
and then add your own stuff