How to block the execution of a method when running EF migration
Hello,
I have a background service registered in Program.cs. It does an HTTP call to an external service that is essential for getting some configuration settings.
This HTTP call should not happen when
ef
commands are executed, so I was wondering what solutions might be there.16 Replies
What is calling these EF commands? The BG Service or the HTTP server?
just run migrations before you even start the background service
running migrations while your app is running at all sounds like a bad idea, i run them at startup
The background service is an
IHostedLifecycleService
. I also create an instance of that just to do a http call, but all of this happens once.
When I run the EF command dotnet ef migrations add ...
it also executes the HTTP call. The app is not running when the migration is being created
For the app that external service call is essential, but it also gets executed whenever I create a migration. I think that is because the app is built one time before the EF command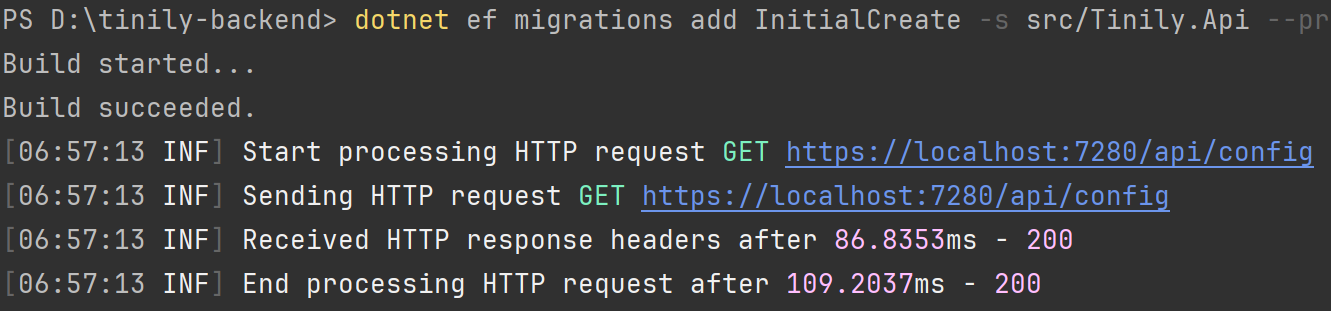
when you run a migration your app is run up until its services are configured and you build the host
so if you're actually making external calls when you're supposed to just be configuring services it will cause problems
if that's unavoidable then your only option is to set up an IDesignTimeDbContextFactory afaik https://learn.microsoft.com/en-us/ef/core/cli/dbcontext-creation?tabs=dotnet-core-cli
Design-time DbContext Creation - EF Core
Strategies for creating a design-time DbContext with Entity Framework Core
Sorry, my notifications were turned off. Thank you, I will look into this as soon as I can
@Jimmacle it's been a while. I iplemented the IDesignTimeDbContext, but the outcome is the same. The HTTP call is still executed.
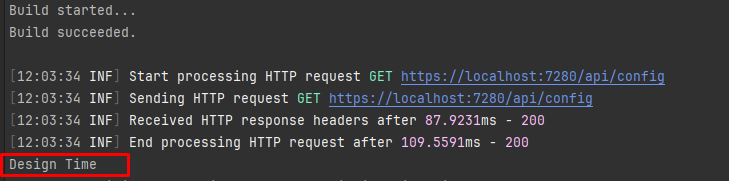
Sorry, it turns out that
IDesignTimeDbContextFactory
is no longer supported in .NET 6 and above. I found a discussion on GitHub about itwhere did you read that?
i'm using it in .NET 8 with no problems
yeah same
it's also still in the EF core documentation that i linked above
Yes, and that is what I implemented. My output shows that the DesignTimeFactory was executed and not the regular DbContext
I found a solution which is available from .NET 7. EF exposes a static flag trough
EF.IsDesignTime
. I simply organized the Program.cs
content in a way to only have code needed for EF in design time.that should not happen if you are doing everything right
- how/from where (exact command, directory) are you running migrations
- where is the dbcontextfactory located (project..)
- is it same project as the dbcontext
my suspicion is that it was not even using that factory you made
i would rather focus on solving the actual problem than workaround
It was, I did a Console.Write in the factory
It was located in the main project, not in which the dbcontext. But either way, it should work from the main project, because for EF commands both the source project and the other project where the dbcontext is located should be specified