middleware on a router level
Right now, i have this setup, where
canExperiment
is also a middleware:
in express, i would do something like:
but i can't seem to make this work in Hono.
to clarify:
- i want all the routes that start with :experimentId
to run through canExperiment
where i need access to the experimentId
parameter.
- i have tried several ways, including creating a sub-router, but i can't seem to make it work
Thank you61 Replies
Hi @rubberduckies,
What you provided should work the
.use()
will apply to all routes defined below in this case. What are you seeing in your case that is making it not work?i don' remember anymore
i'll try to redo it
So I just wrote this example and have no problems with it
so
and in my
canExperiment
experimentId is undefined here
(c.body
comes from my own previous middleware
but in this case, i need for c.req.param('experimentId')
to be available
@Nicoin middleware you can also c.set() then call next()
I was about to bring up the same point
Are you using typescript?
i don't see how that's relevant here... i will use it in another setting
no typescrypt
you can use the one later on as c.var['objname']
So c.body.experimentId is not called yet so that will never be there. It will also never fire because you have return next.
again, my problem here is that i need access to
c.req.param('experimentId')
in my canExperiment middlewareYou need to await next()
i don't think you guys are getting the picture, sorry
or i'm a bit confused, it's also possible
No I understand, can we see the whole middleware?
let me recreate what you are trying to do lets see if we are on the same page
That console log is not showing correct?
And the route is working?
Is there any other custom middleware besides this and the ones you added on the route
in express, i would do something like:
and everything would work
there are two middlewares, running fine.... a bodyParser and a jwt parser to get c.user and c.body
Are you returning next in any?
my problem is here:
i'm getting undefined
Make sure all is
await next()
or it will cause errors
Like thisof course, those are working flawlessly, but i'm not trying to get any params there
its all undefined here if you access this on middleware
That's why I am asking if there is any other middleware. Becuase if it returned next, the context will not be passed to this middleware
i'm not sure where you're getting at, but
c.user
and c.body
are reaching there, so that's not the problem at all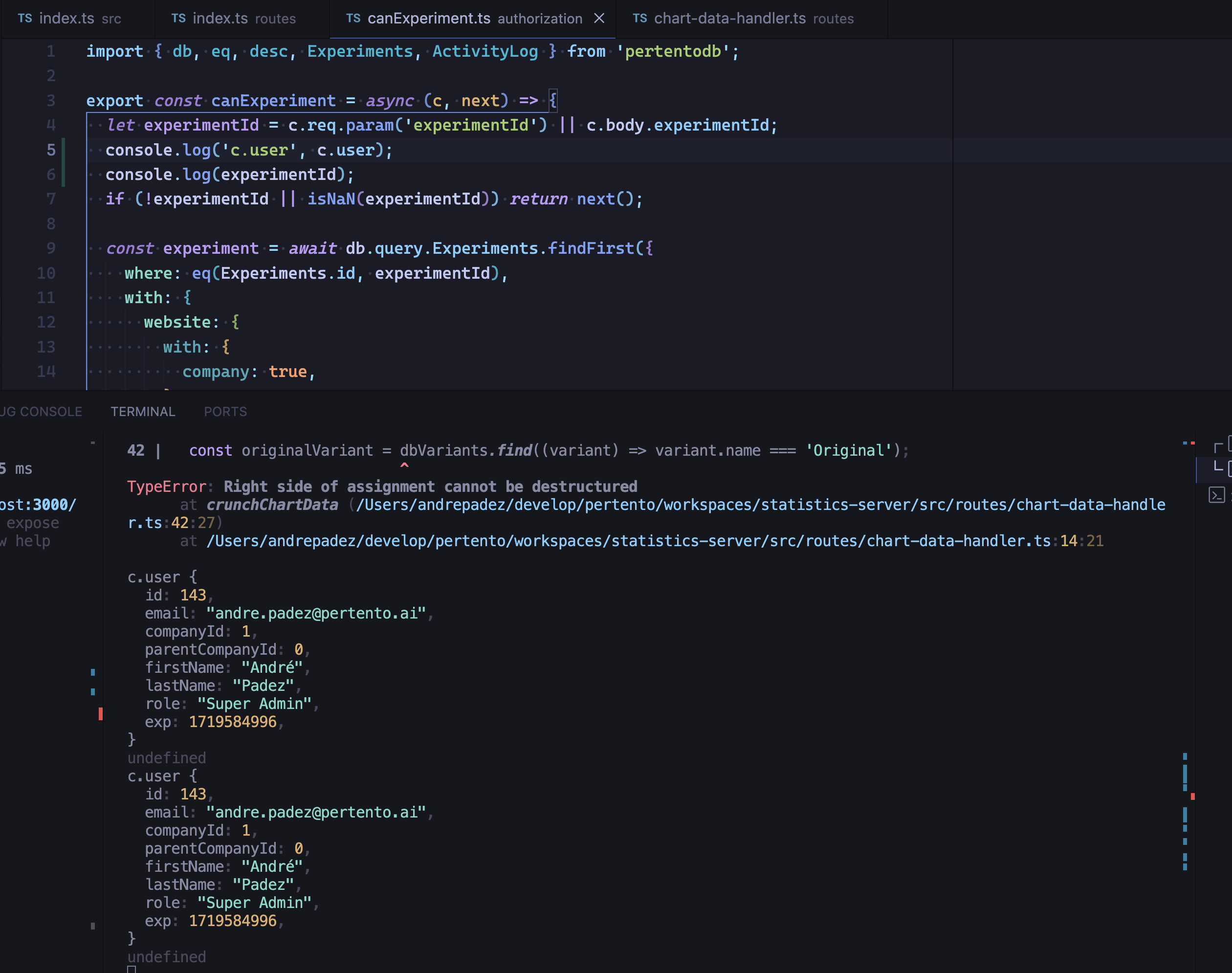
the problem is ONLY with the
:experimentId
param not getting there
forget about anything elseidk why typescript is not shouting at you when you are accesing c.params('ex...') and c.body....
for better typesafety wrap middleware in createMiddleware
i don't care about typescript, not using it, even though the file is named .ts
well if you enable strict true, you will see those things does not exist in its context yet
but i don't, please focus on the problem if you truly want to help
We are trying to help
i know
i'm sorry if i sounded like a dick
not my intention at all
but i feel you're focusing on things that have nothing to do with the problem at hand
coming back to the origin:
if i do this:
the :experimentId param gets to my canExperiment middleware
where did you get
c.params
? i'm using c.req.params('experimentId')
oh mybad
You lost me with how you spoke when we tried to help. Sometimes issues come from other places, that's why I was checking. Looks like @Artifex has got you, another solution is stripping it from you URL. Good luck.
well, thanks for your help
again, i didn't want to offend anyone, i was just getting frustrated
@Nico , @Artifex
i just found the solution
You need to add the url to the middleware
so simple
Are you not using rpc?
no
might want to chain those later on for rpc
what if i don't want to use rpc?
u can also chain it so you get a better readability
that looks good
but it doesn't work for my first route
/monitor
i will definitely use it elsewhereu can append it before the basepath i think
i'll try it
Are you ever planning on using typescript?
🙂 maybe one day, i don't like it
but again, not trying to be a dick, that has nothing to do with the problem i was trying to solve
i'm really thankful for the time you both put into this with me
well c.body.experimentId does not exist totally tho
i know
but it will in other cases
I never mentioned about using it. I just asked because you will see an error to help solve it if you have it on. I was assuming you were not
and if i was using typescrypt it would be a nightmare to get it to work
It was for my debugging purpose
I was going to let you know that too. If you planned on using it I was going to show you two things to add now so it's not a nightmare
i',m sorry @Nico and @Artifex , sometimes written communication doesn't let on exactly the tone we want to convey
again, i'm super thankful for your help
and i hope i haven't gotten into any kind of black list for future inquiries
Nope, you're good
@Artifex , i just tried
and it's not working
i'm gonna keep what i had and am more than good with it
wait haha
yes, this is perfect:
i'm also super tired, i've been at this for more hours than i should today
just append the .get on new Hono()
I would recommend you fix the
return next()
to await next()
I promise you it can cause issues down the road. It can prevent middleware from firing. The only time in middleware a return should be used is if you need to return a responsehave been dealing with Google, to aprove our extension and oauth app
and yes, frustration permiates
all in all, i'm really happy and excited with moving my services to hono
i have worked with express for more than 10 years and it's just the growing pains
maybe 10 years is a bit of a stretch hahah
https://www.youtube.com/watch?v=onzL0EM1pKY
a song for express
FallOutBoyVEVO
YouTube
Fall Out Boy - Thnks fr th Mmrs (Official Music Video)
Official video for Fall Out Boy “Thnks fr th Mmrs” from the 2007 album, ‘Infinity on High’.
Listen to Fall Out Boy: https://Stream.lnk.to/FOB
Watch more videos by Fall Out Boy: https://FallOutBoy.lnk.to/Socials/youtube
New album ‘So Much (for) Stardust’ OUT NOW: https://falloutboy.lnk.to/somuchforstardust
So Much for (Tour) Dust Tickets ON S...
thanks for the memories
but yeah, things are looking good.
Statistics is ported, tomorrow, another 5
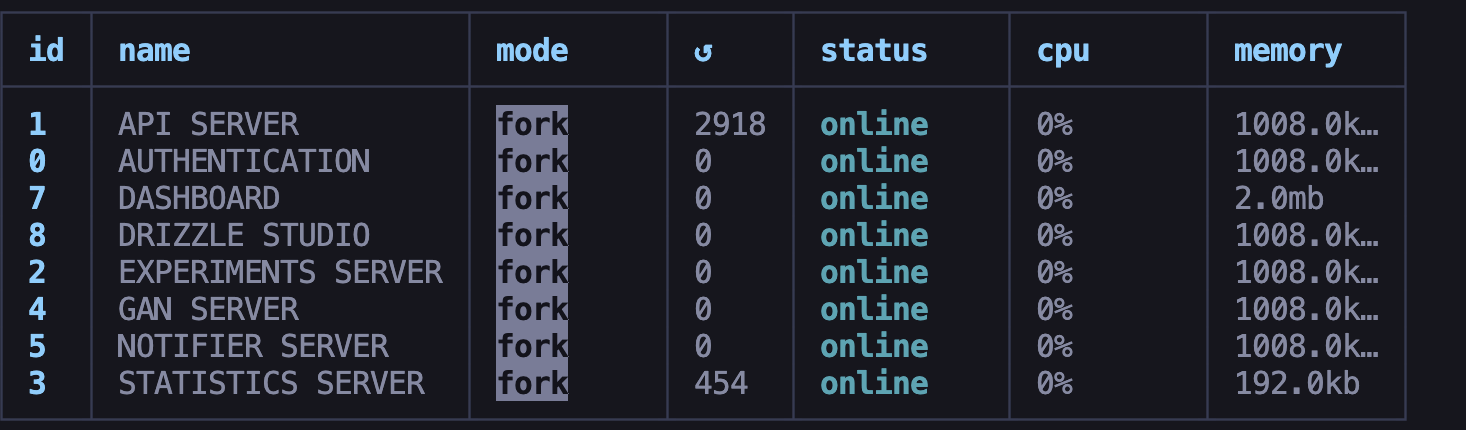