add members to thread
case "adduser": {
await interaction.reply({ content: `Please provide the ID of the user you want to add to the thread.`, ephemeral: bot.config.discordConfig.ephemeral });
const filter = m => m.author.id === interaction.user.id;
const collector = interaction.channel.createMessageCollector({ filter, time: 1500000 });
const thread = interaction.channel;
if (!thread) {
return interaction.reply({ content: 'Thread not found.', ephemeral: true });
}
collector.on('collect', async (m) => {
if (m.content.toLowerCase() === bot.language.cancel) {
await m.delete().catch(() => { });
collector.stop();
return interaction.reply({ content: language.ticketMaxTickets.cancel, ephemeral: bot.config.discordConfig.ephemeral }).catch(() => { });
}
const userId = m.content.trim();
const userToAdd = interaction.guild.members.cache.get(userId);
if (!userToAdd) {
return interaction.followUp('User not found or invalid ID.');
}
try {
await thread.members.add(userToAdd.user.id);
interaction.channel.send(`User ${userToAdd.user.tag} added to thread ${thread.id} successfully.`);
} catch (error) {
console.error('Error adding user to thread:', error);
interaction.channel.send('Error adding user to thread. Please try again later.');
}
collector.stop();
});
collector.on('end', (collected, reason) => {
if (reason === 'time') {
interaction.followUp('No response received. Command timed out.');
}
});
break;
}
case "adduser": {
await interaction.reply({ content: `Please provide the ID of the user you want to add to the thread.`, ephemeral: bot.config.discordConfig.ephemeral });
const filter = m => m.author.id === interaction.user.id;
const collector = interaction.channel.createMessageCollector({ filter, time: 1500000 });
const thread = interaction.channel;
if (!thread) {
return interaction.reply({ content: 'Thread not found.', ephemeral: true });
}
collector.on('collect', async (m) => {
if (m.content.toLowerCase() === bot.language.cancel) {
await m.delete().catch(() => { });
collector.stop();
return interaction.reply({ content: language.ticketMaxTickets.cancel, ephemeral: bot.config.discordConfig.ephemeral }).catch(() => { });
}
const userId = m.content.trim();
const userToAdd = interaction.guild.members.cache.get(userId);
if (!userToAdd) {
return interaction.followUp('User not found or invalid ID.');
}
try {
await thread.members.add(userToAdd.user.id);
interaction.channel.send(`User ${userToAdd.user.tag} added to thread ${thread.id} successfully.`);
} catch (error) {
console.error('Error adding user to thread:', error);
interaction.channel.send('Error adding user to thread. Please try again later.');
}
collector.stop();
});
collector.on('end', (collected, reason) => {
if (reason === 'time') {
interaction.followUp('No response received. Command timed out.');
}
});
break;
}
10 Replies
- What's your exact discord.js
npm list discord.js
and node node -v
version?
- Not a discord.js issue? Check out #other-js-ts.
- Consider reading #how-to-get-help to improve your question!
- Explain what exactly your issue is.
- Post the full error stack trace, not just the top part!
- Show your code!
- Issue solved? Press the button!
- ✅
Marked as resolved by staffError adding user to thread: DiscordAPIError[50001]: Missing Access
at handleErrors (C:\Users\mrpev\Desktop\[Projects]\Phil_MultiBot\node_modules\@discordjs\rest\dist\index.js:730:13)
at process.processTicksAndRejections (node:internal/process/task_queues:95:5)
at async SequentialHandler.runRequest (C:\Users\mrpev\Desktop\[Projects]\Phil_MultiBot\node_modules\@discordjs\rest\dist\index.js:1133:23)
at async SequentialHandler.queueRequest (C:\Users\mrpev\Desktop\[Projects]\Phil_MultiBot\node_modules\@discordjs\rest\dist\index.js:963:14)
at async _REST.request (C:\Users\mrpev\Desktop\[Projects]\Phil_MultiBot\node_modules\@discordjs\rest\dist\index.js:1278:22)
at async ThreadMemberManager.add (C:\Users\mrpev\Desktop\[Projects]\Phil_MultiBot\node_modules\discord.js\src\managers\ThreadMemberManager.js:100:5)
at async MessageCollector.<anonymous> (C:\Users\mrpev\Desktop\[Projects]\Phil_MultiBot\src\components\buttons\ticket.js:445:21) {
requestBody: { files: undefined, json: undefined },
rawError: { message: 'Missing Access', code: 50001 },
code: 50001,
status: 403,
method: 'PUT',
url: 'https://discord.com/api/v10/channels/1242985113894260867/thread-members/1058739292324765766'
}
Error adding user to thread: DiscordAPIError[50001]: Missing Access
at handleErrors (C:\Users\mrpev\Desktop\[Projects]\Phil_MultiBot\node_modules\@discordjs\rest\dist\index.js:730:13)
at process.processTicksAndRejections (node:internal/process/task_queues:95:5)
at async SequentialHandler.runRequest (C:\Users\mrpev\Desktop\[Projects]\Phil_MultiBot\node_modules\@discordjs\rest\dist\index.js:1133:23)
at async SequentialHandler.queueRequest (C:\Users\mrpev\Desktop\[Projects]\Phil_MultiBot\node_modules\@discordjs\rest\dist\index.js:963:14)
at async _REST.request (C:\Users\mrpev\Desktop\[Projects]\Phil_MultiBot\node_modules\@discordjs\rest\dist\index.js:1278:22)
at async ThreadMemberManager.add (C:\Users\mrpev\Desktop\[Projects]\Phil_MultiBot\node_modules\discord.js\src\managers\ThreadMemberManager.js:100:5)
at async MessageCollector.<anonymous> (C:\Users\mrpev\Desktop\[Projects]\Phil_MultiBot\src\components\buttons\ticket.js:445:21) {
requestBody: { files: undefined, json: undefined },
rawError: { message: 'Missing Access', code: 50001 },
code: 50001,
status: 403,
method: 'PUT',
url: 'https://discord.com/api/v10/channels/1242985113894260867/thread-members/1058739292324765766'
}
@Night Ghost ^^
all ready has that perms it has admin
Does the user have perms to see the channel the thread is in?
yea

The user to add doesn't have permission to view the channel.
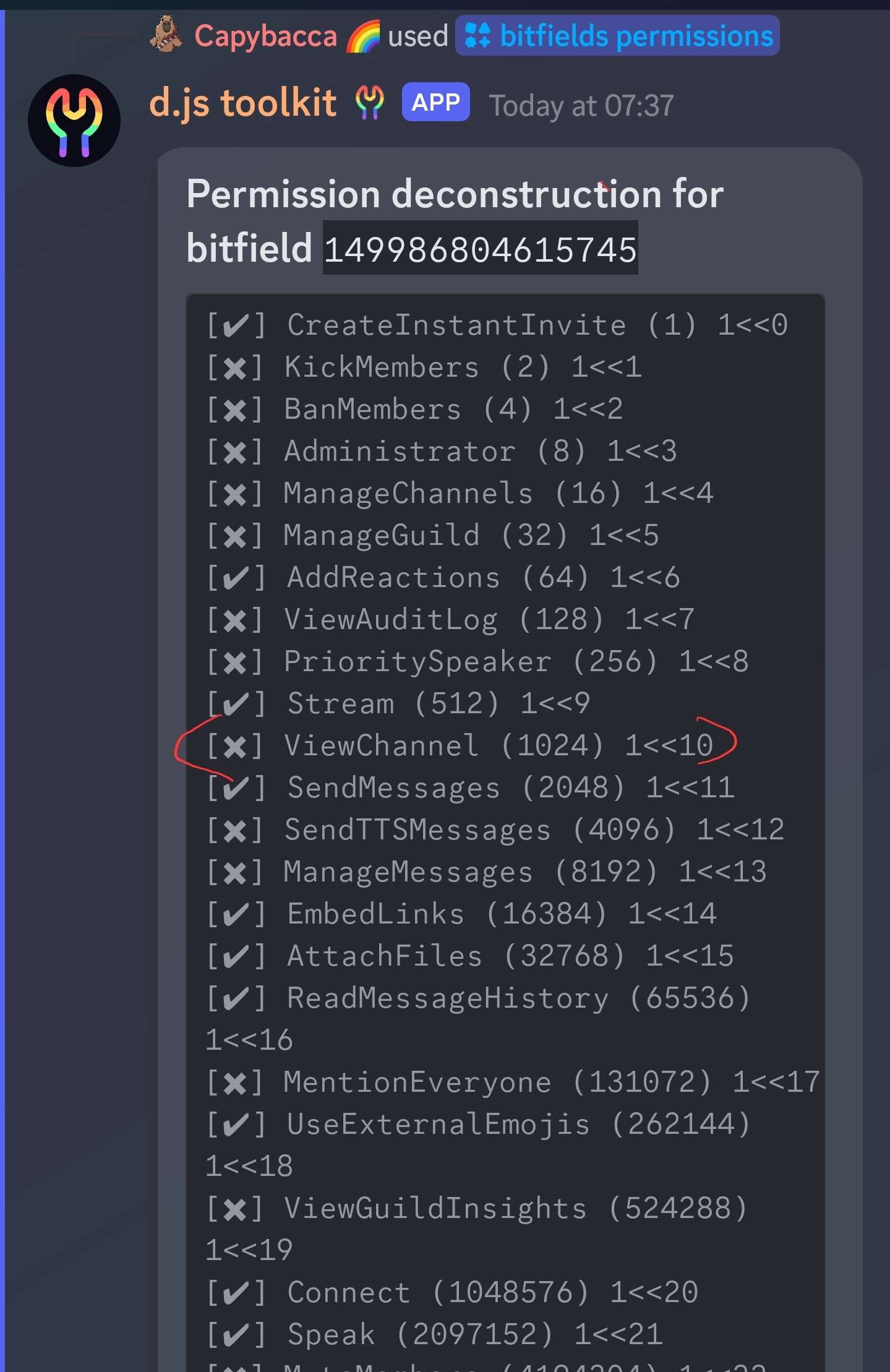
um
ty worked was the channel for reason it was updated