Help improving this code
ok so when i query a favorite row i know its id because its already been inserted, problem arises when i try inserting a favoritedto that i made in the program, i cant know what the id will be for it, but i dont have to either because the add method of my dao will just insert it and sql will generate a key for it. problem is when im trying to save a favorite ride i need to pass a favoritedto as argument but a favoritedto requires a id to be constructed, so i just put garbage number to construct it, there is no issue then all fine. problem is i dont think this is good written and i would refer to this as spaghetti code
83 Replies
⌛
This post has been reserved for your question.
Hey @userexit! Please useTIP: Narrow down your issue to simple and precise questions to maximize the chance that others will reply in here./close
or theClose Post
button above when your problem is solved. Please remember to follow the help guidelines. This post will be automatically closed after 300 minutes of inactivity.
in my favoritesDto i require a key representing the id in the table, thats so when a user wants to edit a favoritedto we can know which one he is editing and use a update query
problem arises when adding a favoritesDto to the table itself, my constructor requires a id, but when adding a id is not needed as the sql will generate one for me (
id
INTEGER NOT NULL PRIMARY KEY AUTOINCREMENT)Unknown User•9mo ago
Message Not Public
Sign In & Join Server To View
yeah I know
it doesnt matter what I put in ID in the constructor
when inserting
but for me its useful to know the id
cuz when i update the db i need the id
@borgrel
for better understanding
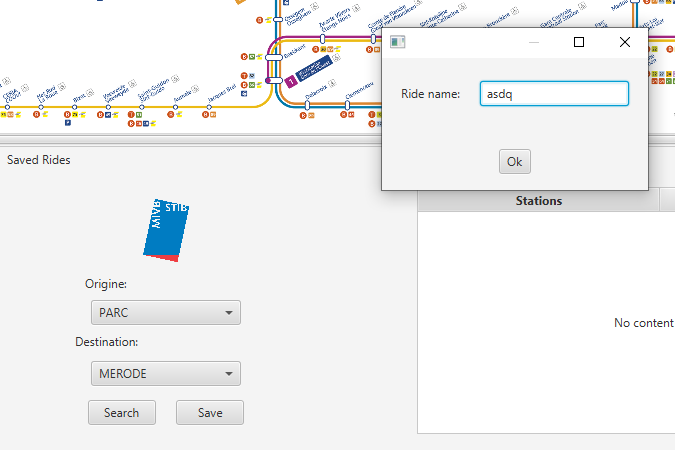
this is when I add an element to the db
i dont need the primary key as it will be generated
problem is here when i modify a row in favorites table
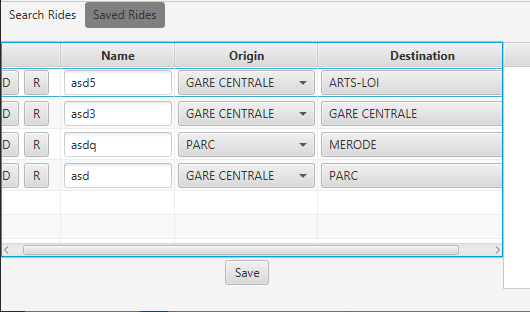
i need the id here
so thats why i have an id attribute in favoritesDto
Unknown User•9mo ago
Message Not Public
Sign In & Join Server To View
but then i need 2 different dto's classes
ok so things go like this:
when i go in the saved rides table:
I get all the rows from the database -> i create a favoriteDto instance that has the name, origin, destination, id -> presenter will ask the view to display all the favoriteDtos -> they get displayed -> now the user can change the name, the origin and the destination station
when user clicks save button -> presenter asks the model to save the changes -> model talks to repository -> repository uses dao to update (the id in favoritedto is useful for this)
Unknown User•9mo ago
Message Not Public
Sign In & Join Server To View
my problem arises when:
user wants to save a ride to his favorite rides, selects destination and origin and name, clicks button -> presenter asks model to save, issue is i cant use same favoriteDto cuz that one requires a key
but bruh i need the id to update the row no ?
Unknown User•9mo ago
Message Not Public
Sign In & Join Server To View
its not for the displaying purpose
that can be filtered
Unknown User•9mo ago
Message Not Public
Sign In & Join Server To View
the only reason i gave it an id
is so i can update them
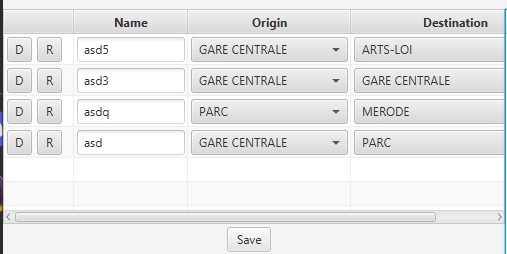
if i click save here what should i do ?
go through each row and update it right
but i need the primary key for each then
maybe im not understanding you or you are not understanding me
Unknown User•9mo ago
Message Not Public
Sign In & Join Server To View
bro thats the point
he can change those
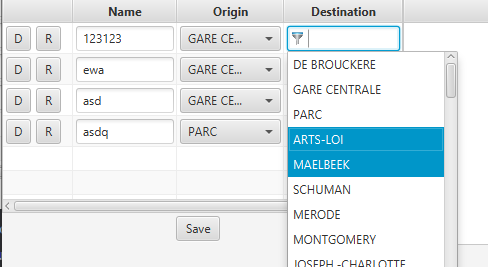
he can change everything
name
origni
destination
there is
ok
wait
:
Unknown User•9mo ago
Message Not Public
Sign In & Join Server To View
yh i guess
Unknown User•9mo ago
Message Not Public
Sign In & Join Server To View
wdym
Unknown User•9mo ago
Message Not Public
Sign In & Join Server To View
the app doesnt allow login system none of that
just a basic app
to calculate shortest path
like this
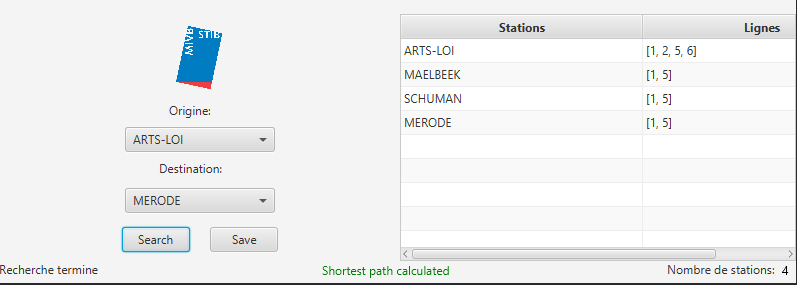
wdym with bridging table
ill show u what a row of my table looks like
Unknown User•9mo ago
Message Not Public
Sign In & Join Server To View
i mean
there is a way
i can get old destination
and old origin
u think thats better ?
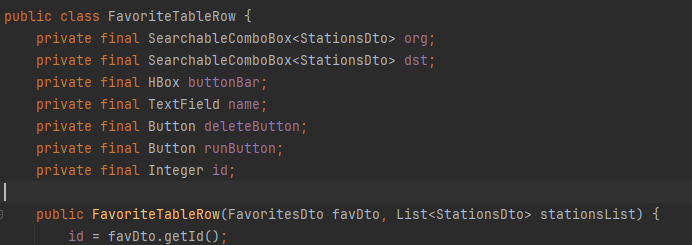
when I load values in my table:
presenter asks model for all rows in favorites table -> model gives them -> presenterp asses them to the view -> makes a favoritetablerow -> adds it to the table
Unknown User•9mo ago
Message Not Public
Sign In & Join Server To View
yh
yeah because of dtos
i need a dto with the key and a dto where i dont care about the key
maybe i give a default value to the key and i add a setter ?
if the dto is not in the table and will be added it can keep the default random value, if its read from the favorites table then set its id
Unknown User•9mo ago
Message Not Public
Sign In & Join Server To View
I know how I can ignore the key
I can save in the FavoriteTableRow the old origin and old destination
and use those for updating
like u mentioned before
with ur query
here
Unknown User•9mo ago
Message Not Public
Sign In & Join Server To View
i thought i needed the key
but i just realised
no
no
i didnt think i needed the key
thats why i gave it a random value
in my code for inserting
because i knew it wouldnt matter fori nserting
because i coded it
but thats spaghetti code i think
I just didnt know how to do it the other way
but now I realise
in my own definition of the table
.
UNIQUE (id_origin, id_destination)
means I can identify a row in the table with those 2
and when I make a new FavoriteTableRow I can hold those 2 ids in there and use them for the update query
what do u think @borgrel
Unknown User•9mo ago
Message Not Public
Sign In & Join Server To View
yeah but which one do u think is better
give a default for int to favoritedto and then a setter
or simply not use it and use origin and destination for update
imo latter one looks more correct but if you dont agree we can debate on it
Unknown User•9mo ago
Message Not Public
Sign In & Join Server To View
modify the old one
but i can always make a copy of it when instantiating the favoritetablerows
Unknown User•9mo ago
Message Not Public
Sign In & Join Server To View
idk i cant see it as a normal user because i made it and i know waht it does
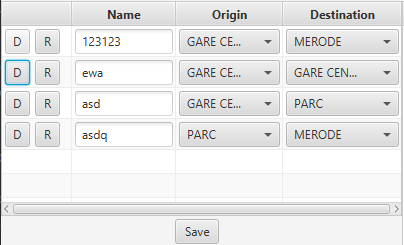
i mean he has to click save
its intuitive no ?
i coded it this way because it was intuitive for me
Unknown User•9mo ago
Message Not Public
Sign In & Join Server To View
ill jut put in the button
SAVE CHANGES
this way its more straightforward ig
Unknown User•9mo ago
Message Not Public
Sign In & Join Server To View
and maybe a button to add a new one
it will just add an entry to the table
Unknown User•9mo ago
Message Not Public
Sign In & Join Server To View
idk what a listview is
i just made this because this is the first thing that came up to my mind
lmao
Unknown User•9mo ago
Message Not Public
Sign In & Join Server To View
they can compute it as much as they want
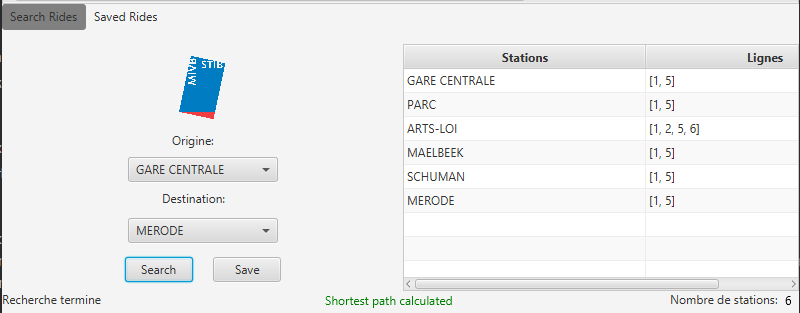
then they have the option to save it too
Unknown User•9mo ago
Message Not Public
Sign In & Join Server To View
yh
Unknown User•9mo ago
Message Not Public
Sign In & Join Server To View
they are metro lines bro
STIB is a metro company
Unknown User•9mo ago
Message Not Public
Sign In & Join Server To View
yeah
MOVIB STIB LOGO
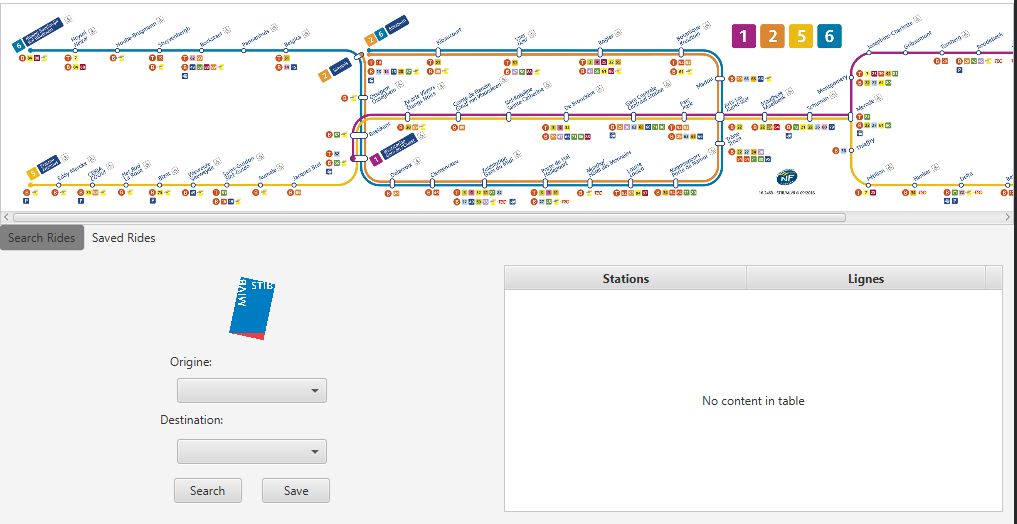
there is the lines in the top also
the metro network @borgrel
Unknown User•9mo ago
Message Not Public
Sign In & Join Server To View
@borgrel
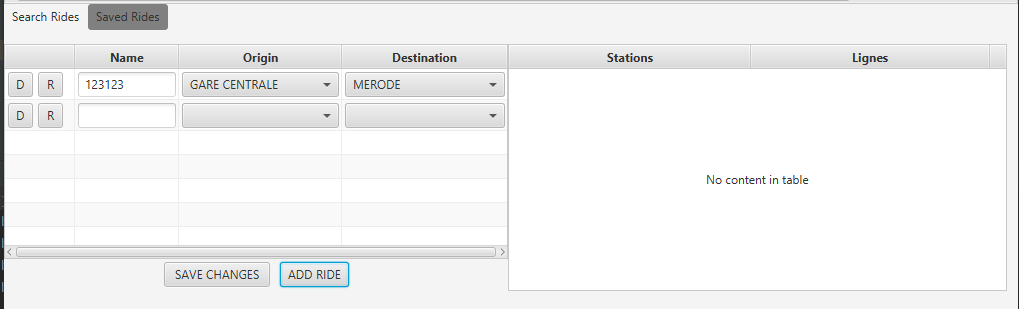
yeah thats how they are known
1 is stockel
2 is simonis
5 is erasmus
6 is elisabeth
Unknown User•9mo ago
Message Not Public
Sign In & Join Server To View
no they are shown without the square brackets
they are shown as
1,5
instead of
[1,5]
just realised while recording this that there was an error we should be able to compute a ride before saving changes
the issue relies here
im doing presentation.search(f)
except f
is
at this poiint
a favoritedto
with no origin
nor destination
Unknown User•9mo ago
Message Not Public
Sign In & Join Server To View
its hard when u
make the program
and the view
i should make the view one day '
and the other the model
issue is im doing everything at once
so i look at it more like a puzzle
putting things together to get soemthing that works in the end
but ig that just spaghettifies the code
Unknown User•9mo ago
Message Not Public
Sign In & Join Server To View
@borgrel
so what about the error handling lmao
do i use observer observable
@borgrel
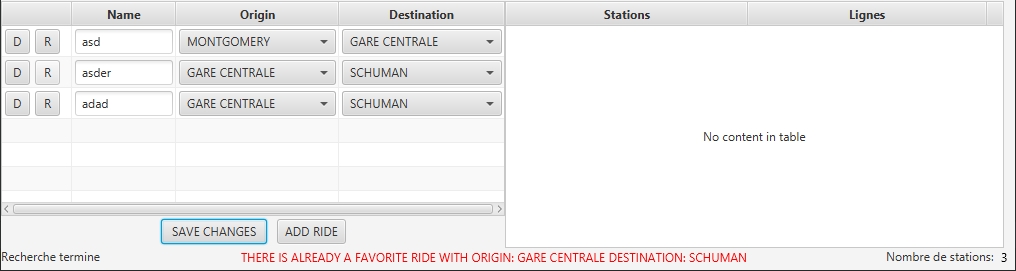
im going to add a red background maybe
on the origin and destination searchablecomboboxes
the ones that interfere
Unknown User•9mo ago
Message Not Public
Sign In & Join Server To View
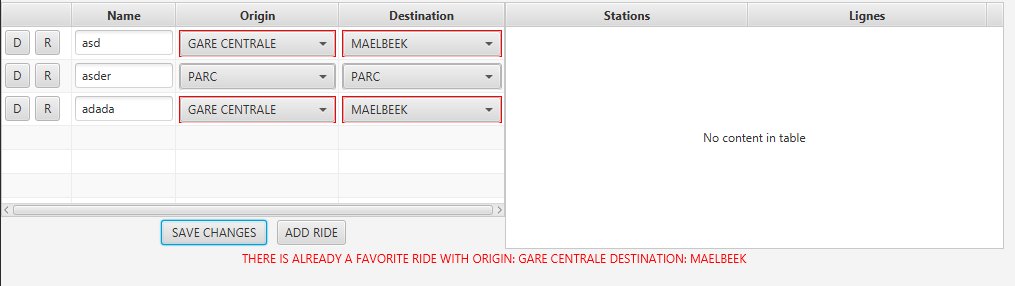
how does it look
💤
Post marked as dormant
This post has been inactive for over 300 minutes, thus, it has been archived.
If your question was not answered yet, feel free to re-open this post or create a new one.
In case your post is not getting any attention, you can try to use /help ping
.
Warning: abusing this will result in moderative actions taken against you.